A C++ program to encrypt text
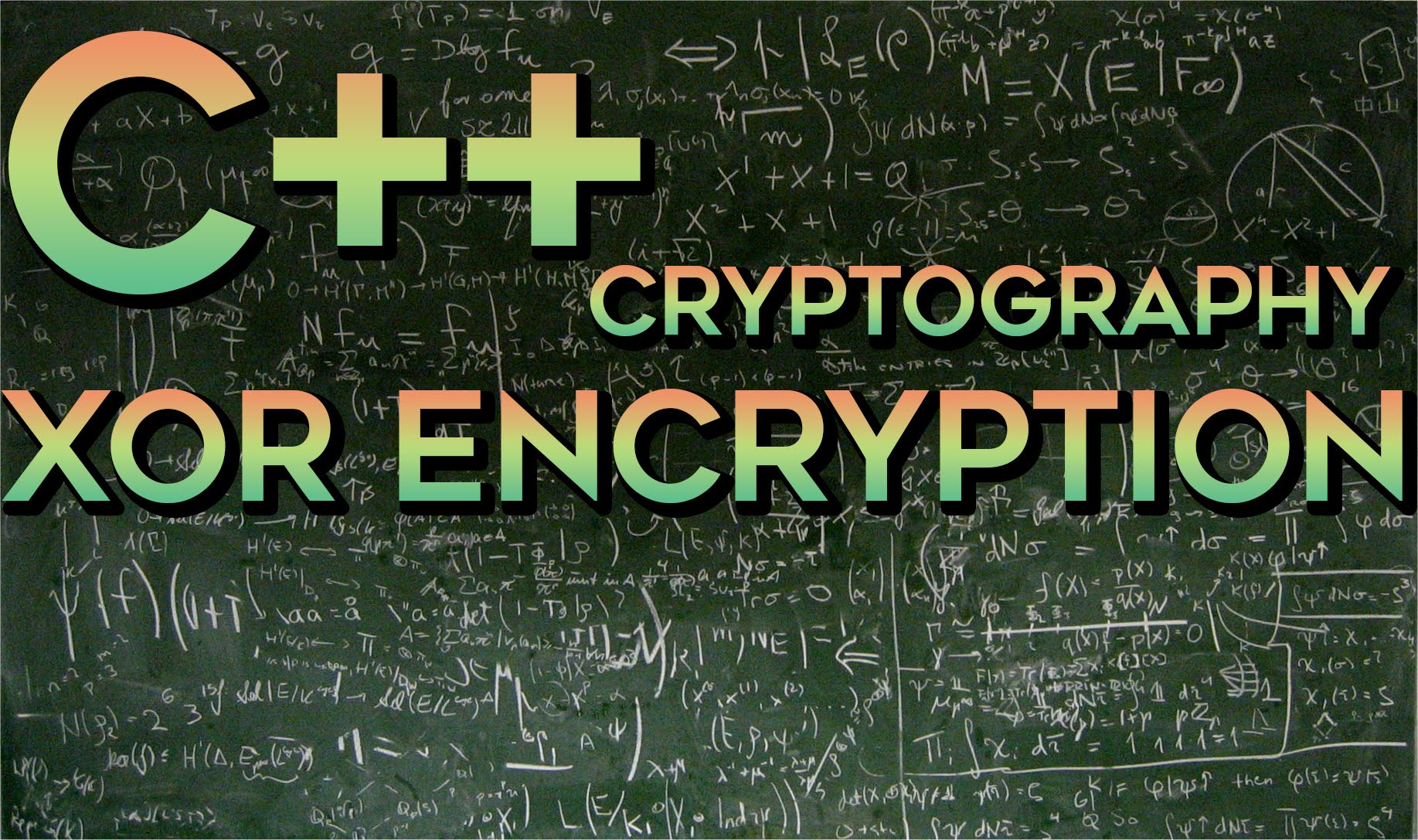
For me computer programming can be define as something quite difficult and complex since you have to deal with objects, functions, variables, and other component parts. It was two years ago when i took the subject: Introduction to Computer Programming or CSC 101 and it was truly difficult but the good thing is that despite of the complications I got to learn some of the basic parts in depth. But to get a sense of how this program fits and work together you must see a complete working program. All you need to start coding is to download Codeblock.
How to download: Visit Codebloack.com and select Download tab and click on Download binary release, choose operating system as Windows and select the latest version available for download. You can use this link to download https://sourceforge.net/projects/codeblocks/

To make a program to encrypt text or messages were just one among the many task and activities we had in the computer laboratory during the time i had my computer programming subject and we have to create a program that yield encrypted result as presented in the katipunan alphabet. See photo above.
Activity: Encryption time!
Encryption is the process of transforming a message into something uncomprehensible in order to hide the information from other parties/people. This is currently used by government organizations (including spy organizations), military, police, business sectors, and even in the normal life. The reverse process is known as "decryption": transforming encrypted messages into some understandable language we are using.
In the old days, people have devised ways to encrypt certain messages. This is also normal in the early Filipino days. Way back during the Katipunan revolution, their leaders devised an encryption algorithm that will cloak their messages from suspicion against the Spanish officials. A key-map has been devised and can be found here (https://en.wikipedia.org/wiki/Katipunan#Language_and_alphabet). (Please consider LL as L).
You must create a program that will implement the Katipunan secret message algorithm. Your program must get the message from the user and it will be print out the encrypted message. On convertion, spaces and other special characters like * . ! and others will not be included. So, the convertion, as per the key-map, only applies to alphabet characters only. You can upgrade your program by making the user to choose to continue encyrpting or not. If not, the program exits. To get the string input, you can use the gets() function.
Running Example:
Original Message: Mabuhay si Bonifacio!
Encrypted text: VZBXHZY SN BCLNHZKNC!
C++ program to encrypt text:
#include<stdio.h>
#include<string.h>
void beauty(){
printf("\nPress: 1 - Encrypt \n");
printf(" 2 - Exit \n\nYour selection : ");
}
void loveyou (){
int c,d;
for (c=1;c<=24576;c++){
for (d=1;d<=24576;d++){
}
}
}
int main (){
printf("******************************************\n");
printf("* \n");
printf(" ENCRYPTION TIME \n");
printf(" *\n");
printf("******************************************");
char JOJO[1];
char text[1234];
int a, b;
while (1) {
beauty();
gets(JOJO);
a = strcmp(JOJO, "1");
b = strcmp(JOJO, "2");
if (a == 0){
printf("\nKindly Enter Plain Text Here: ");
gets(text);
int i, s = strlen(text);
printf ("\n\nThe Encrypted Text Is: ");
for (i=0;i<s;i++){
if (text[i]== 'a'){ printf("z"); }
else if (text[i]== 'A'){ printf("Z"); }
else if (text[i]== 'b'){ printf("b"); }
else if (text[i]== 'B'){ printf("B"); }
else if (text[i]== 'c'){ printf("k"); }
else if (text[i]== 'C'){ printf("K"); }
else if (text[i]== 'd'){ printf("d"); }
else if (text[i]== 'D'){ printf("D"); }
else if (text[i]== 'e'){ printf("q"); }
else if (text[i]== 'E'){ printf("Q"); }
else if (text[i]== 'f'){ printf("h"); }
else if (text[i]== 'F'){ printf("H"); }
else if (text[i]== 'g'){ printf("g"); }
else if (text[i]== 'G'){ printf("G"); }
else if (text[i]== 'h'){ printf("h"); }
else if (text[i]== 'H'){ printf("H"); }
else if (text[i]== 'i'){ printf("n"); }
else if (text[i]== 'I'){ printf("N"); }
else if (text[i]== 'j'){ printf("l"); }
else if (text[i]== 'J'){ printf("L"); }
else if (text[i]== 'k'){ printf("k"); }
else if (text[i]== 'K'){ printf("K"); }
else if (text[i]== 'l'){ printf("j"); }
else if (text[i]== 'L'){ printf("J"); }
else if (text[i]== 'm'){ printf("v"); }
else if (text[i]== 'M'){ printf("V"); }
else if (text[i]== 'n'){ printf("l"); }
else if (text[i]== 'N'){ printf("L"); }
else if (text[i]== 'o'){ printf("c"); }
else if (text[i]== 'O'){ printf("C"); }
else if (text[i]== 'p'){ printf("p"); }
else if (text[i]== 'P'){ printf("P"); }
else if (text[i]== 'q'){ printf("k"); }
else if (text[i]== 'Q'){ printf("K"); }
else if (text[i]== 'r'){ printf("r"); }
else if (text[i]== 'R'){ printf("R"); }
else if (text[i]== 's'){ printf("s"); }
else if (text[i]== 'S'){ printf("S"); }
else if (text[i]== 't'){ printf("t"); }
else if (text[i]== 'T'){ printf("T"); }
else if (text[i]== 'u'){ printf("x"); }
else if (text[i]== 'U'){ printf("X"); }
else if (text[i]== 'v'){ printf("m"); }
else if (text[i]== 'V'){ printf("M"); }
else if (text[i]== 'w'){ printf("w"); }
else if (text[i]== 'W'){ printf("W"); }
else if (text[i]== 'x'){ printf("u"); }
else if (text[i]== 'X'){ printf("U"); }
else if (text[i]== 'y'){ printf("y"); }
else if (text[i]== 'Y'){ printf("Y"); }
else if (text[i]== 'z'){ printf("a"); }
else if (text[i]== 'Z'){ printf("A"); }
else { printf ("%c", text[i]);}
}
printf ("\n\n");
printf ("\n");
printf("******************************************\n");
printf("* *\n");
printf("* ENJOY!!!!! *\n");
printf("* *\n");
printf("******************************************");
}
else if (b == 0){
printf ("\nQuitting Program!\nPlease wait!\n\n\n");
loveyou();
printf ("Press Any Key To Close!\n\n");
break;
}
else {
printf("\nYou Have Selected An Incorrect Selection!\n\n");
}
}
return 0;
}
"The difficulty of learning complex subject, like computer programming, is that so much of what you learn depends on everything else there is to learn". Through it you will learn to expose to different field and it could help you widen your knowledge.
Did I miss something? You are very free to comment down below for further questions and reactions. For more updates, do follow me @mr-jobean. Upvotes and so as resteems are highly appreciated. Thank you steemians!!!.
References:
You'd be far better off replacing that ridiculous if-else tree with two arrays, one for the unencrypted letters and one for the encrypted letters and then write a single for loop that goes through the text and matches each letter to its encrypted counterpart. Your code will be much shorter and much less prone to error.
Also the "loveyou" function does absolutely nothing from what I can see.
thank you @chonusone for the info.. it really help me a lot
@mr-jobean, change your tags, the lesson to ocd-resteem, tutorial to steemiteducation, computer to philippines. :)
ok @shairanada thank you...