5 - Learn Python, The cycles
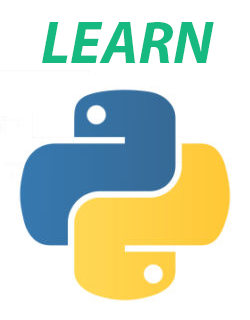
There are two types of loops in this language, for and white. They are also called loops.
FOR
For executes a specific block of code and returns the elements one after the other, for example lists, etc.
Given five numbers, ten are added to these:
seq = [10, 50, 100, 150, 200]
for n in seq:
print n, '+ 10', '=', n+10
Output
10 + 10 = 20
50 + 10 = 60
100 + 10 = 110
150 + 10 = 160
200 + 10 = 210
But sometimes it can happen that you have to repeat the cycle an established number of times, this helps us with the range function.
for n in range(0, 10):
print 'The number is ', n+1
Output
The number is 1
The number is 2
The number is 3
The number is 4
The number is 5
The number is 6
The number is 7
The number is 8
The number is 9
The number is 10
WHILE n = 1 A particular aspect of this language is that you can add an else at the end of the cycle
The while loop, unlike for, executes a block of code as long as the starting condition is true
n = 1
while n < 6:
print(n)
n += 1 # n=n+1 the same thing
Output
1
2
3
4
5
In some cases we need to exit the loop as soon as a condition has occurred, so we will use break
while n< 6:
print(n)
if n == 3:
break
n += 1
Output
1
2
3
On the other hand, if you want to continue to execute the cycle and therefore you no longer want to use the current condition, use continue
n = 0
while n< 6:
n += 1
if n == 3:
continue
print(n)
Output
1
2
4
5
6
n= 10
while n > 6:
print(n)
n -= 1
else:
print 'smaller 6'
Output
10
9
8
7
smaller 6