HTML5 Tutorial
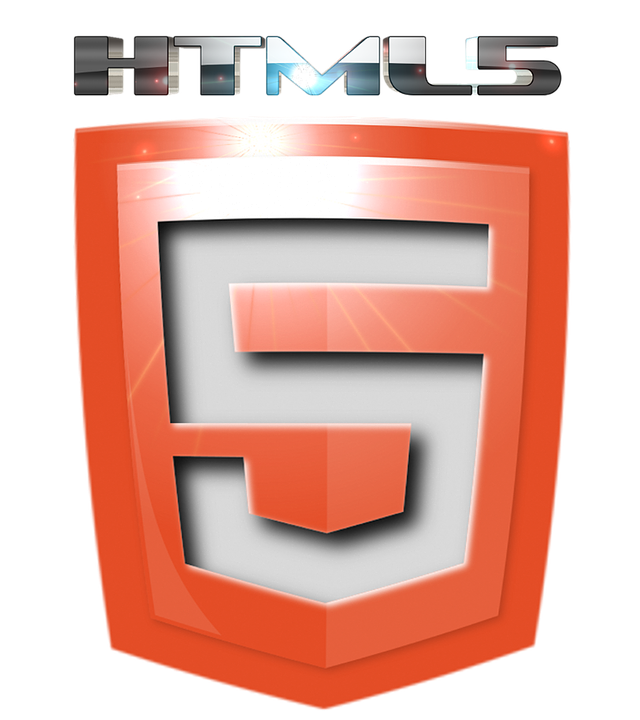
What is HTML?
HTML(Hyper Text Markup Language) is the language that is used to describe the structure of a webpage. It's not considered a programming language, but a markup language. HTML is basically the skeleton of a webpage, and the other technologies like CSS and Javascript are the "meat" of the webpage. HTML is an interpreted language, not a compiled language. Elements are the basic entities on which HTML is built.
How to Write and Run HTML Code
- You can write code in any text editor(notepad, Sublime Text, Atom etc)
- Save the file with a
.html
file extension. - Open the file in a browser using the "Open with" option in the right click menu.
HTML5 is the latest, and most popular version of HTML(there's a minor revision, HTML5.1, that came out last year, but it has very few changes).
Tip: If you want to see the HTML of a website, go to the website in Chrome/Firefox and press Ctrl+Shift+I
to open the dev tools. In the Elements tab, you'll see the markup of the site you've loaded.
Note: Most markdown viewers can also interpret HTML. So for example, you can use HTML in Steemit posts and comments. That said, all HTML outputs in this tutorial are screenshots in order to show how they would look in a normal page.
Overview of a HTML Element
This is an example of an element:
<p class="classname">Stuff</p>
These are the different parts of an element:
- The
<p...>
part is called the opening tag. - The
</p>
part is called the closing tag. Stuff
is called the content of the elementclass="classname"
is called an attribute of the element. Attributes are additional properties that can be set in order to change the layout or appearance of the content of the element. The value of an attribute is generally enclosed in either double, or single quotes.
Not all elements have all these parts. For example, the img element, used to add images, has no closing tag, and generally, it has no content.
A Basic HTML document
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Page Title</title>
</head>
<body>
(html comment removed: This is an awesome comment )
</body>
</html>
This is a basic HTML document, without any actual content. If you opened this in a browser, you'd see a blank page.
Let's go through the different elements in this document:
<!DOCTYPE html>
This tag is used to denote the version of HTML inside the document. Since most HTML written today is HTML5, this is mostly the same on every webpage.<html>...</html>
This is the top level HTML tag.Every other tag must be inside this tag.<head>...</head>
The head tag is generally used to put information not for the user,but for the web browser, and for search engines.So anything inside this tag is not visible on the web page.<meta charset="utf-8">
The meta tag has several attributes, likename
andcontent
, but in this case, thecharset
attribute is used to set the character set toUTF-8
. Again, almost all English language webpages have the charset attribute set to UTF-8, so this is the same on most pages.<title>...</title>
The title tag is used to set the page title. You can see the title on the handle of the tab in a browser.
This screenshot shows where the title is displayed in the browser.
<body>...</body>
The body tag contains the main content of the webpage. Everything that you can see on a website, is in the body tag.(html comment removed: This is an awesome comment )
This is a comment. Comments are not displayed on the webpage.
HTML Entities
In HTML, characters like <, >,",' and & are part of the syntax itself. So, if you want to include them in your text, you have to use special codes called HTML entities to represent them. They are given below.
Character | HTML Entity |
---|---|
< | < |
> | > |
" | " |
' | ' |
& | & |
For example, in order to write this in a HTML document:
This is a <p> tag
You have to write this:
This is a <p> tag
Text Formatting in HTML
Headings
There are 5 types of heading elements, h1 through h6. h1 is the largest, while h6 is the smallest.
<h1>This is h1 text</h1>
<h2>This is h2 text</h2>
<h3>This is h3 text</h3>
<h4>This is h4 text</h4>
<h5>This is h5 text</h5>
<h6>This is h6 text</h6>
This is how they look in the browser:
Paragraphs
The <p>
tag is used to create paragraphs. Paragraphs automatically get newlines added below them.
For example:
<p>This is a paragraph of text</p>
This is a line of text
This is how it looks in the browser:
Lists
Lists are of 2 types - unordered and ordered, denoted by the <ul>
and <ol>
tags. Inside these, <li>
tags are used to denote list items.
Unordered Lists
Example of unordered lists:
<ul>
<li>This is a list item</li>
<li>This is a list item</li>
<li>This is a list item</li>
<li>This is a list item</li>
</ul>
This is how it looks like in the browser:
The <ul>
tag has a style attribute list-style-type
that can be set to disc
,circle
or square
. For example, if you replace the opening ul tag with <ul style="list-style-type:square">
, this is how it will look:
Ordered Lists
Example of ordered lists:
<ol>
<li>This is a list item</li>
<li>This is a list item</li>
<li>This is a list item</li>
<li>This is a list item</li>
</ol>
This is how it looks like in the browser:
The <ol>
tag also has a type
attribute for changing the list type. Values can be 1
, A
, a
, I
and i
For example, here's a list with roman numerals:
<ol type="i">
<li>This is a list item</li>
<li>This is a list item</li>
<li>This is a list item</li>
<li>This is a list item</li>
</ol>
Bold and Italic Text
You can make text bold using the <strong>
or <b>
tags.
This is an example of <strong>strong text</strong>
<br>
This is an example of <b>bold text</b>
You can make text italic using the <em>
or <i>
tags.
This is an example of <em>emphasised text</em>
<br>
This is an example of <i>italicised text</i>
For most people, <b>
and <strong>
are visually going to look the same. It's the same for the italic tags.
So What's the difference?
Practically, the difference is mostly on things like screen readers. The screen reader will change its voice when reading content inside a <strong>
or a <em>
tag in order to let the listener know that the content is important/emphasised. But with the <b>
and <i>
tags, there's no such changes. It will sound the same, because these tags are only for visually changing text.
So which one should i use?
You should almost always use <strong>
or <em>
. The only time you should use the other 2 tags is when you only want to change how the text looks, but not attach any importance to it.
You might also have noticed the <br>
tag in those examples. That is used for going to a new line.
Drawing a line to separate content
The <hr>
tag is used to draw a line.
This is a line of text.
<hr>
This is another line of text.
Super and Subscript
The <sup>
tag is used for superscript text, while the <sub>
tag is used for subscript.
This tutorial is pretty Small<sup>TM</sup>
<br>
The chemical formula of Water is H<sub>2</sub>O
Showing Unformatted, Raw Text
If you want to add text as it is, with no formatting changes, use the <pre>
tag.
<pre>
* One Star
** Two Stars
*** Three Stars
**** Four Stars
</pre>
Whereas, without the <pre>
tag, this is how it would look like.
Tables in HTML
You can tabulate data using the <table>
tag. Inside this tag, data is arranged in rows, using the <tr>
tag. Inside the row, is a list of table elements using the <td>
tag. For headers, use the <th>
tag.
This is the code you need to write for a simple table:
<table border="1px">
<tr>
<th>Person</th>
<th>Age</th>
</tr>
<tr>
<td>Tom</td>
<td>1009</td>
</tr>
<tr>
<td>John</td>
<td>-18</td>
</tr>
<tr>
<td>Ron</td>
<td>12</td>
</tr>
</table>
And this is how it looks:
Notice the border="1px"
in the opening tag. Without that, the table by default has no borders.
But really, those borders look pretty bad. For a normal, reasonable border, we set the border-collapse
style attribute to collapse
.
Code:
<table border="1px" style="border-collapse:collapse">
<tr>
<th>Person</th>
<th>Age</th>
</tr>
<tr>
<td>Tom</td>
<td>1009</td>
</tr>
<tr>
<td>John</td>
<td>-18</td>
</tr>
<tr>
<td>Ron</td>
<td>12</td>
</tr>
</table>
This is how it looks:
Column and Row Spanning
Sometimes, you want a row or column to span more than a single cell. To span columns we use the colspan
attribute, while for spanning rows, we use the rowspan
attribute.
Example of Column Spanning:
table border="1px solid black" style="border-collapse:collapse">
<tr>
<th>Person</th>
<th>Age</th>
<th>Marks</th>
</tr>
<tr>
<td>Tom</td>
<td>1009</td>
<td>90</td>
</tr>
<tr>
<td>John</td>
<td colspan=2>-18</td>
</tr>
<tr>
<td>Ron</td>
<td>12</td>
<td>20</td>
</tr>
</table>
Output:
As you can see, the 3rd row only has 2 cells. But the 2nd cell spans 2 columns.
Row spanning is quite similar:
<table border="1px solid black" style="border-collapse:collapse">
<tr>
<th>Person</th>
<th>Age</th>
<th>Marks</th>
</tr>
<tr>
<td>Tom</td>
<td>1009</td>
<td rowspan=2>90</td>
</tr>
<tr>
<td>John</td>
<td>-18</td>
</tr>
<tr>
<td>Ron</td>
<td>12</td>
<td>20</td>
</tr>
</table>
Output:
Images, Audio and Video
Images
Images can be embedded using the img tag. The src
attribute is for the source of the image. The source can either be on the local disk, or it can also be from a different website.
This is an example of an image from a different site embedded into our page.
Output:
Audio
The <audio>
tag is used for streaming audio. As with images, you can also stream audio from a different website. Inside the audio tag, we use <source>
tags for actually adding audio sources.
Example of audio:
<audio controls>
<source src="audiotrack.mp3" type="audio/mp3">
</audio>
This is how it looks in the browser:
As you can see, the <audio>
has the controls
attribute set, without which there wouldn't be any controls. In <source>
the type
attribute isn't always necessary, but it is a best practice.
Video
The <video>
tag is used for streaming video. It's actually very similar to the audio tag, in that we use <source>
tags inside the main tag in order to add the video source.
Example of video:
<video controls>
<source src="bunny.mp4" type="video/mp4">
</video>
This is how it looks in the browser:
The audio and video tags also have other attributes, like autoplay
, that autoplays media, and loop
that plays the media source in a loop.
Note: ALWAYS add the controls
attribute while embedding audio/video, because if you don't there are no controls by default. This is especially important if you're using the autoplay
attribute.
Hyperlinks
Of course, the internet wouldn't be a network if we couldn't link to other pages. The <a>
tag is used to link to other pages. The href
attribute contains the page we want to link, and the content inside the tag is what's displayed on our page.
And example of linking to steemit:
<a href="https://steemit.com">Go to Steemit!</a>
In this screenshot, The link is purple because I clicked the link to go to steemit. Unopened links are blue.
Adding links to images
It's possible to nest the img tag inside the <a>
tag to create an image that links to another page.
Example:
This would show you a normal image, but if you clicked it, it would take you to steemit.com
And with that, we finish this overview of the basics of HTML5. There are a few other features I haven't covered, but those are only really useful once you learn CSS and Javascript, and besides, this is the basics, so this will suffice.
I know this is a big post, but I hope it's written in simple enough language to be easy to understand. If you have any questions, please ask them in the comments below, and thank you for reading this VERY LONG post.
Other Great Resources for Learning HTML
Sources
I referenced MDN's HTML guides a lot while making this tutorial.
Header image from pixabay.
All other images are screenshots.
What does MSPL stands for? I noticed that this tag has been used specifically for writing about coding. Does it stand for Minnow Support Project Learning?
mspl stands for Minnow Support Project Library
I see and when exactly this tag should be used? If we are writing post about some coding stuff?
This post was actually a submission for a contest held by @aggroed. It was a requirement for that contest that the post have 'mspl' as one of its tags.
Thanks, this will be extremely useful to me as it has been forever and a day since I have worked with html coding. Resteemed
Thanks for reading and resteeming :-)
You are welcome
Hi, thanks for the article and the work.
I do not want to come across that I bleat but I have to improve a little bit.
Most what you mentioned is more HTML4 (or XHTML). video and audio is HTML5. In addition, HTML5 is much more. I have here once a graphic what HTML5 are realy is :-)
image in big
(image source wikipedia https://en.wikipedia.org/wiki/HTML5)
The post title are misleading a little bit. The real new HTML5 Tags are
<header>
<section>
<article>
<nav>
<main>
<footer>
and many more, like your
<video>
or<audio>
what you mentioned. But the most important stuff of HTML5 are the device communication.The tags you have mentioned arent really useful unless you style them with CSS, and since this is a beginner-level tutorial, I didn't include them.
As for the other things being HTML4, there are a few small differences between it and HTML5, which can be confusing to beginners. And obviously, if you're learning HTML right now, you have to learn HTML5.
But anyway, thanks for reading :-)
Hm that's not exactly correct. SEO is important, syntax / text interpretation is important. 100 DIVs helps nobody, but if you structure your page with article, sections ... bots, scripts and all technical stuff can interpret it.
Than, if you use sections, you can use header element and your text structure is more clear than in (X)HTML :-)
Its the same as in 2000`s, everybody use table as design elements. But table are not developed for design, it is for structured data.
I do not want to say that the tutorial is bad. for beginners it is a good start! But it becomes even more confusing for beginners when someone comes and say "use real HTML5 ... connect to the Share API" "what abput canvas" :-) ... I hope I could explain it well what i want to say
This will surely help... thank you!!! ☺️
Thanks for reading :-)
So can i apply HTML5 to my stem posts, I think i can believe the video and audio what of if i have a html code will it work if i paste it in my stem post. sorry just a novice. or can it work as a steemit app ?
Hello, I am doing a new project, where I will mention few really good articles. Do you mind, if I include yours? I am going to post it today or tomorrow :)
Yeah sure! Glad you loved the post :-)
Yes, really good for beginners!
Great works, I wish you success
!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!
Good post I would be very thankful if you please follow and upvote me thanks.This post recieved an upvote from sanjeev.kapoor . If you would like to recieve upvotes from sanjeev.kapoor on all your posts, simply FOLLOW @sanjeev.kapoor