Programming lesson 2: Maximum Caloric Deficit Calculator (in C++)
Note: I wrote this app because in my own health research I noticed there wasn't a calculator I could find anywhere on the web which does this. Since I had to do the calculation by hand I figured I would code up an automated way and use it as an opportunity to teach others some C++.
Max Caloric Deficit Calculator
#include <iostream>
using namespace std;
int bodyweight;
float bodyfatpercent;
int totalbodyfat;
int maxdeficit;
int main() {
cout <<"This calculator will determine the maximum safe caloric deficit without muscle loss! \n";
cout << "To begin, please enter your current body weight in pounds: \n";
cin >> bodyweight;
cout << "Next, enter your current body fat percentage, (for example if 20% enter 0.20): \n";
cin >> bodyfatpercent;
cout << "Finally, we calculate your max deficit by multiplying your body weight and body fat percentage to produce your total fat weight!" << endl;
cout << bodyweight*bodyfatpercent << endl;
cout << "Now enter your result displayed on the screen, as it is your total bodyfat in pounds: \n";
cin >> totalbodyfat;
cout << "Below is your maximum safe caloric deficit \n";
maxdeficit = totalbodyfat * 31.4;
cout << maxdeficit;
return 0;
}
This code is very simple and relies on the formula popular on body building forums and based on this academic research paper. We know a pound of fat is 3500 calories roughly. We know that people who have higher body fat percentages can withstand larger caloric deficits because 1 gram of fat contains 9 calories which is higher than protein or carbs. In essence fat contains more energy than protein or carbs but when body fat becomes low the body still requires a baseline level of energy to stay alive (basal metabolic rate) and if the deficit puts the body below the minimum threshold and there aren't enough calories available from fat then muscle is the only thing left which can be used to keep the body alive.
The formula states that 31.4 calories can be extracted per pound of fat per day. If you have more pounds of fat than you can preserve more muscle while in a deficit. Inputs include the total body weight in pounds, the body fat percentage, both of which can be multiplied to find the total body fat in pounds. This total body fat number becomes an input and is then multiplied against 31.4 to produce the total amount of calorie deficit an individual can handle safely without losing muscle.
The source code is straight forward but I will review some aspects below:
using namespace std;
int bodyweight;
float bodyfatpercent;
int totalbodyfat;
int maxdeficit;
This part of the code specifically includes the input output stream, and the standard namespace is used. This allows for the use of cout and cin. The integers are then declared, bodyweight is a number, total bodyfat is a number, bodyfatpercent is a float because you're dealing with decimals. Floats are commonly used for dealing with decimals in C++.
The main part of the program is straight forward, it presents text to the user using cout and requests input from the user using cin which stores that input into the global variables which were declared in the beginning.
The main calculations occur at:
```cout << bodyweight*bodyfatpercent << endl;```
and
maxdeficit = totalbodyfat * 31.4;
Which we finally display to the user using:
cout << maxdeficit;
Overall something like this will take 15 minutes or less to write but it shows how useful programming can be for automating mundane yet routine calculations. C++ is not the only language to do this in, as it is even easier to do in a language like Python. In addition this does not include a GUI but a GUI could be included trivially as the code would not have to change much. A simple calculator like this would be useful for a fitness website or smart phone app. Perhaps if I get bored enough I'll write the GUI and go further.
References
Alpert, S. S. (2005). A limit on the energy transfer rate from the human fat store in hypophagia. Journal of theoretical biology, 233(1), 1-13.
Web:
- http://forums.lylemcdonald.com/archive/index.php?t-11223.html
- http://www.bodyrecomposition.com/fat-loss/the-energy-balance-equation.html
nice article..
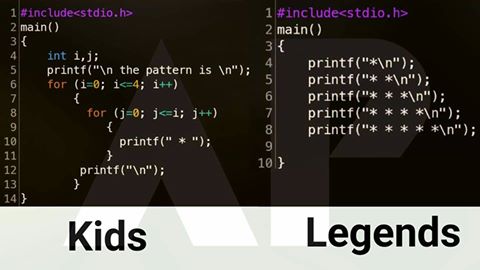
Are you legend ? :D @dana-edwards @alanw
I don't understand the question. But apparently the legends could just use the for loop to accomplish the same thing the kids are doing. So the kids are being more concise from what I can see.
i'd like your posts and follow you I hope you want to like post US with iklas also follow me
This is amazing. I dont know how to code yet but when I start learning I'll be combining a few resources this being one.
I'm also huge into fitness so this lesson is right up my alley. 👌👌👌
Super cool post.
Congratulations @dana-edwards! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honnor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
If you want to support the SteemitBoard project, your upvote for this notification is welcome!