First Steps On The Steemit Blockchain - Programming in Ruby - Part 2
Expanding on my last article I exlore further API calls to pull some data from my account and present it in the terminal. Follow along!
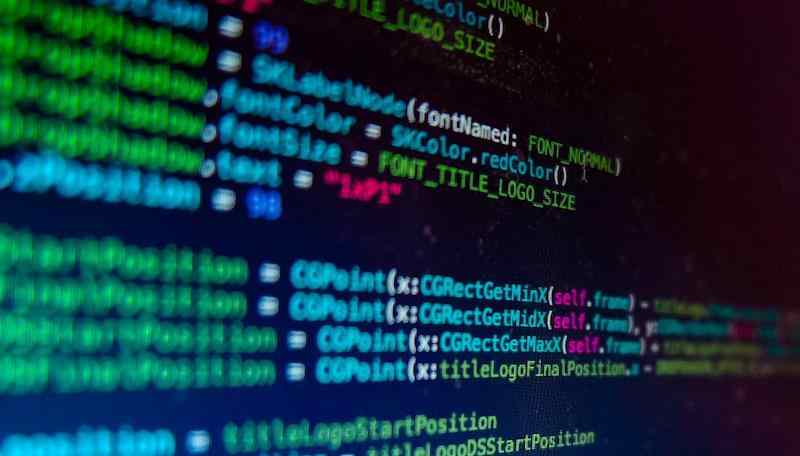
(image source)
Let's jump right in
If you would like to follow what I'm doing this at home you should check out Part 1. Last time we pulled a list of my followers from my account. Today we want to get some more account data.
This tutorial is written in Ruby with the help of Radiator API client provided by @inertia.
Fallback nodes
To interact with the steem blockchain we need to connect to a public api. If that node fails, Radiator provides the functionality to fallback on a different node. We can implement it like this:
options = {
ur: 'https://api.steemit.com',
failover_urls: [
'https://api.steemitstage.com'
]
}
api = Radiator::Api.new(options)
Pulling account data
Let's begin by grabbing my account and printing the username like this:
my_account = api.find_account('cryptonik')
puts "Looking up account data..."
puts "Account: #{my_account.name}"
Now we can get my STEEM balance, by calling the balance method and converting it to a float:
$steem_balance=my_account.balance.to_f
Very similar for SBD balance:
$sbd_balance=my_account.sbd_balance.to_f
Print it to console with puts:
puts "Balance (STEEM): #{$steem_balance}"
puts "Balance (SBD): #{$sbd_balance}"
We can now pull the voting power, and to get it right (in percent) we divide the stored value by 100:
$voting_power=(my_account.voting_power/100.to_f)
Let's get my reputation. This one is a little tricky. The reputation is stored in a different format and we have to first change it to a human readable format.
Here is @sevinwilson breaking down the math:
- Calculate the log base 10 of the raw score from the desired user at steemd.com/@username. LOG base 10 of 26727073581 = 10.4269514092915
- Subtract 9 from this number. 10.4269514092915 – 9 = 1.42695140929153Multiply this number by 9. 1.42695140929153 x 9 = 12.8425626836238
- Add 25. 12.8425626836238 + 25 = 37.8425626836238
- Once you get this number (37.8425626836238) and round down you have your reputation score = 37.
Here is me putting that into code:
$reputation=((Math::log10(my_account.reputation.to_f)-9)*9+25)
puts "Reputation: #{$reputation}"
=>51.989230344184215
That looks pretty messy. Let's limit our float to 2 decimal points:
puts "Reputation: %.2f" % $reputation
=>51.99
Much better, we can use the same format for the other puts.
Now we can append a slightly modified followers count from last time. This time we won't print the whole list but rather just grab the number of followers. Remember, we can call the length method on the array of users constructed by map.
followapi = Radiator::FollowApi.new
response = followapi.get_followers('cryptonik', 0, 'blog', 1000)
followers_ary = response.result.map(&:follower)
$sum_followers=followers_ary.length
puts "followers: #{$sum_followers}"
Let's put it together!
Make a file in your editor of choice and save it with the *.rb extension.
require 'radiator'
options = {
ur: 'https://api.steemit.com',
failover_urls: [
'https://api.steemitstage.com'
]
}
api = Radiator::Api.new(options)
my_account = api.find_account('cryptonik')
puts "Looking up account data..."
puts "Account: #{my_account.name}"
$steem_balance=my_account.balance.to_f
$sbd_balance=my_account.sbd_balance.to_f
$voting_power=(my_account.voting_power/100.to_f)
$reputation=((Math::log10(my_account.reputation.to_f)-9)*9+25)
puts "Reputation: %.2f" % $reputation
puts "Voting power: %.2f" % $voting_power
puts "Balance (STEEM): %.2f" % $steem_balance
puts "Balance (SBD): %.2f" % $sbd_balance
followapi = Radiator::FollowApi.new
response = followapi.get_followers('cryptonik', 0, 'blog', 1000)
followers_ary = response.result.map(&:follower)
$sum_followers=followers_ary.length
puts "followers: #{$sum_followers}"
Action!
$ruby myfollowers.rb
Feel free to try this at home and if you get stuck you can always ping me.
Link to Part 1 of this series.
Resources:
Radiator docs
Radiator github
Steem github
More Articles like this:
Do you want know about Google alternatives?(Read article)
Do you want to know more about free and open software? (Read article)
- Nick ( @cryptonik ) -

Excellent post Nik.
Regarding another subject - whats with the steemit wallets being "black background" and the reputation number being 25 - have you heard of any changes I have missed?
Thank you, Arthur. Yeah, it's just a temporary glitch on this website. Steemit.com is just a visual interface sitting on the Steemit blockchain - if you use a different website to access the steem blockchain, like busy.org or utopian.io you will not encounter this error.
Thanks for the info Nik - cheers!
So is reputation on the blockchain then? I wasn't sure if it was.
Yep, and you can look it up at: https://steemd.com/@canadianrenegade
It's just stored in a different format, this is your reputation before conversion:

Thanks for the link. MY reputation is getting pretty close to 59!
I am wondering how someone gets a reputation in the mid to high 70's. Who is upvoting them that has a higher rep? My rep is going up at snails pace and I occasionally get upvotes from high rep individuals. Did they change the algorithm to make it harder to build rep or something?
I trying to figure it out, but I believe that the whole answer is included in this formula:
You collect MVESTS based on the payout of your posts, at the same rate. However, when converted to Steemit_reputation that cheecky "log 10" is what's causing all the trouble: log10(1000)=3, log10(100,000)=5 ... You see how that logarithm growing super slow with large numbers?
I think that is all. But I haven't verified this assumption yet, there could be more to the algorithm.
Dan designed it so it would grow at a slower rate with increasing numbers, thats for sure. I have seen only '77' rep so far in existence. You may be pleased to know, that according to the steemit statistics you belong to a very small 58'er group ;)
Right click image and select "view image" to enlarge ;)
I swear papa-peppers rep went from the high 60's to 77 in less then 6 months. Maybe I got him confused with someone else?
Possible, if posted super frequently + whale votes ;)
Great post, really informative and helpful. Thanks for posting
Thanks mate