Google’s Flutter framework lets you easily and rapidly create native mobile apps for Android and iOS
Flutter is a new framework from Google for creating mobile apps that is currently in alpha but is already being used to great success in production mobile apps on both the Google Play store and Apple’s App store, by Google itself and by third parties. I’m using it right now to create a native Steem app for Android and iOS, and I found the framework so nice to use that I want to talk about it a bit here.
If you want to see how well apps made with Flutter run, there is a gallery app that you can install on Android which shows off all widgets and three example applications.
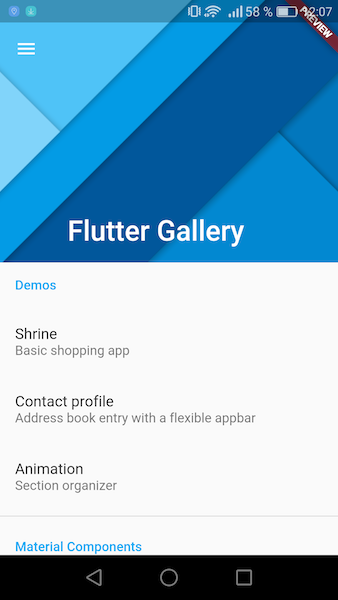
There are three things that are most interesting about Flutter:
- The apps are native on both Android and iOS (no slow web views, no Dalvik virtual machine like Java and Kotlin use, gets compiled directly to byte code)
- The apps run on both Android and iOS and possibly desktop in the future
- Flutter is very easy to learn and use since it uses Google’s Dart programming language and takes inspiration from Facebook’s React framework, it makes native app development as easy as web development
Let’s talk about these three things in detail. On iOS, native apps aren’t special, all apps written in Objective-C and Swift are. On Android however, the two main languages Java and Kotlin which you use to create Android apps go through a Java virtual machine called Dalvik. You can however use something called the Android NDK to create truly native Android apps in C/C++, games often do that because they need the added speed it gives them or because they are ported from a C++ codebase and this way they don’t have to be rewritten from scratch. Flutter makes use of this feature and compiles the Dart code you write to native byte code, bypassing the virtual machine.
Flutter doesn’t use web views, yet the apps you write are still cross platform. How? Flutter doesn’t use native widgets provided by the underlying operating system, instead it adds it’s own set of widgets that closely mimic the official ones, Google went to extreme lengths to get every microsecond of animations right so that you don’t notice the difference. Flutter has its own rendering engine. And it is by design super easy to create your own widgets, because it’s been shown that most of the successful apps in the stores do just that, they create beautiful widgets with their own brands design, but before Flutter that tended to be harder and more complex to do than it would need to be, it was easier to just use stock widgets.
Flutter is super easy to learn and use. You need to write much less code to create an app and it’s all structured in an easy to understand tree of widgets with parent and child widgets. And putting it all together is done using Google’s own Dart programming language, a language with a syntax very close to JavaScript with added types like Microsoft’s TypeScript, but with a lot of powerful features underneath that were inspired by timeless language classics such as Smalltalk-80, which allows for superior tooling and debugging. It’s a pure object oriented language, everything is an object, there are no primitive types, but if you want to read more about that I wrote an overview article about Dart here on Steemit.
Flutter can access operating system (and third party) APIs through use of plugins. There are already many plugins available using Dart’s pub package manager, but you can also write your own using Java/Kotlin for Android plugins or Objective-C/Swift for iOS plugins. So when you switch to Flutter, your prior knowledge of these other languages won’t be for nothing, you will just have to use it much more rarely, if at all.
Let’s take a look at a very simple Flutter app, this is the template that is created when you create a new Flutter app. It has text with a button click counter in the center, a floating action button in the lower right and if you click the button, the counter is increased by one. There is also an app bar at the top, with the title “Flutter Demo Home Page”.
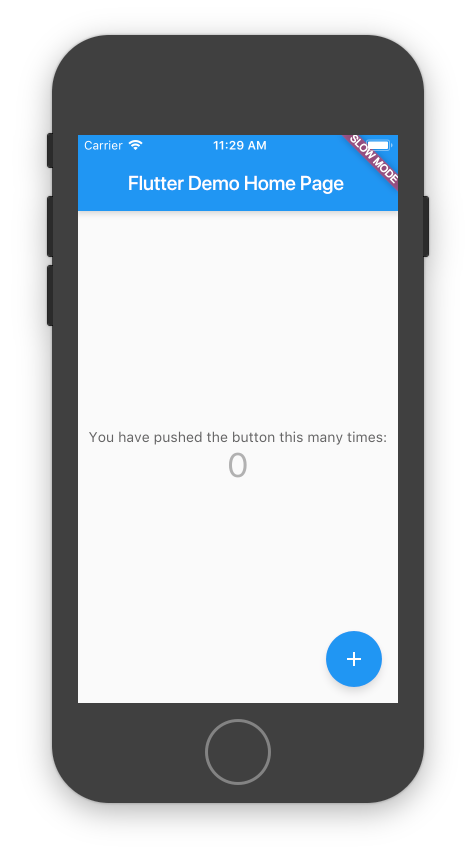
Here you can see the whole code:
Quite nice, right? Fits all on the screen.
Let’s add a normal button to that which does the same thing as the floating action button, just to show you how you’d add more widgets here.
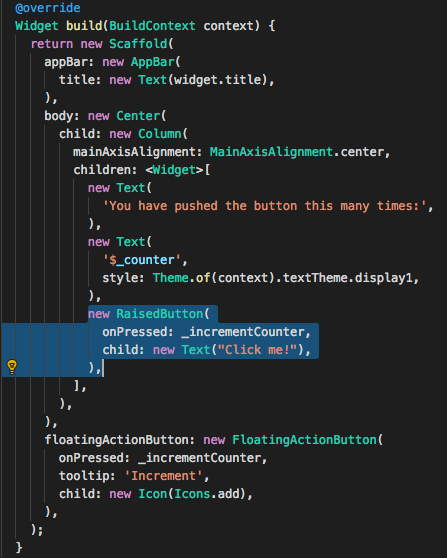
Result:
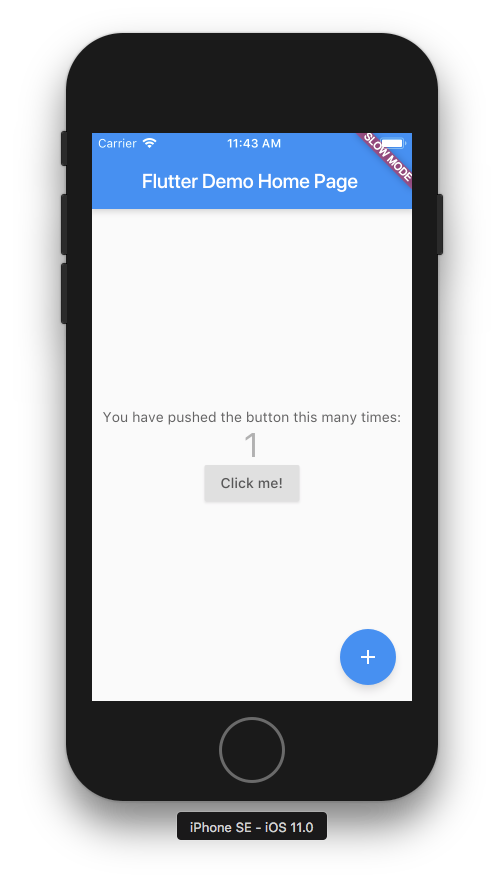
And lets add an icon to that button. For that we wrap it in a layout widget called Row, which lays out its children in a row.
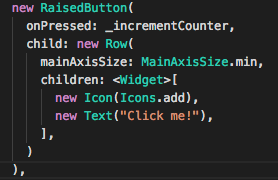
Result:
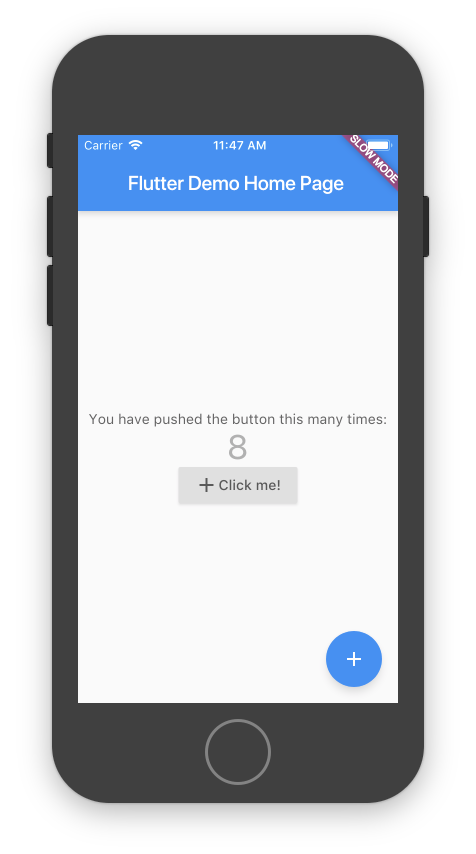
And the button looks a bit boring, so let’s change the color to blue.
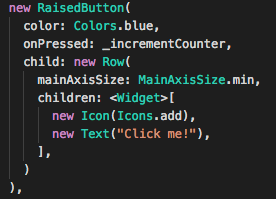
Result:
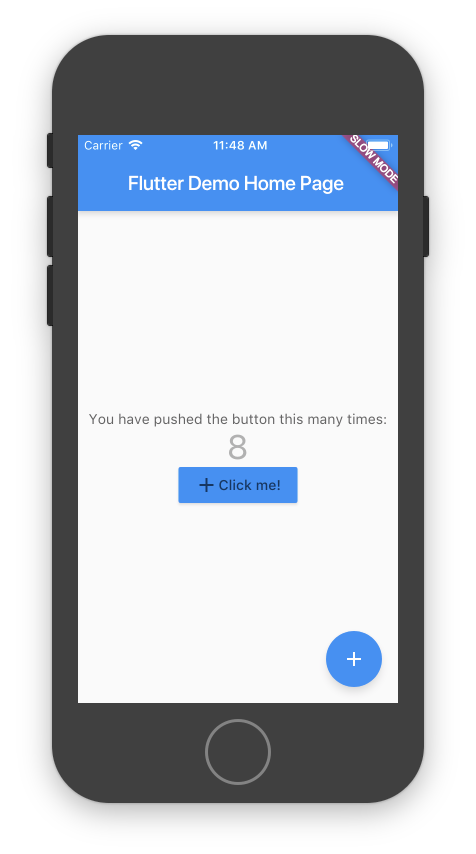
As you can see, the tree structure makes it very easy to add things. It’s all parent child relationships. You add a widget, that widget can have a child, so you add one to it, that child might have another child or many children if it’s a widget that is doing layout like a row or a column. It’s really simple.
Another nice thing about Flutter is that it does hot reload. During all of the changes I made, I had to just press the hot reload button and saw the change reflected in the iPhone simulator instantly. I could have used a real phone as well, or the Android emulator. A small Flutter app like this compiles in under a second anyhow, but compilation speed isn’t the only thing hot reloading gives you, it also preserves your state. So if you change the color here and do a hot reload, the text we displayed (the counter) hasn’t changed, but the color has!
All in all, Flutter gives you the ease of web development but brings it over to mobile app development, but with fully native code. Again, no web views, this is not HTML.
Installation
While Flutter is still in alpha there isn’t a nice installer that you can just double click and it does all the rest, but it’s still remarkably easy to set everything up. The command line flutter tool that Google developed for this makes it really easy.
The first step is cloning a git repository to get the flutter tool, and adding the folder to your PATH.
git clone -b alpha https://github.com/flutter/flutter.git
Next you will have to run the command flutter doctor
. The first time you use the Flutter tool, it downloads all the dependencies it can to set up your development environment, so it takes a while the first time. The flutter doctor command you will use multiple times during installation, it outputs a checklist of things you still need (and how to install them). This is what makes it so easy, it’s an interactive install helper which shows if everything was done correctly and tells you how to fix things.
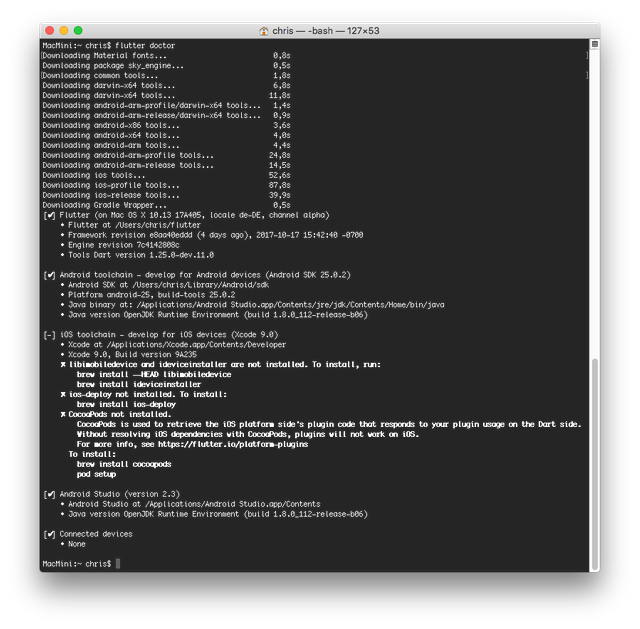
On macOS, for Android I didn’t have to install anything because I already had Android Studio installed and flutter automatically found it. For iOS I had to install three packages with homebrew, I already had XCode and the iPhone Simulator installed, so that wasn’t hard either.
At that point you just connect a mobile phone, or fire up either an Android or an iOS emulator and flutter doctor
will automatically list it under connected devices.
The next step is to create your first flutter project and run it on said device. flutter create projectName
creates a new flutter “Hello world” template. cd projectName
followed by flutter run
will compile it and open it automatically on your emulator or connected mobile device (open the emulator first).
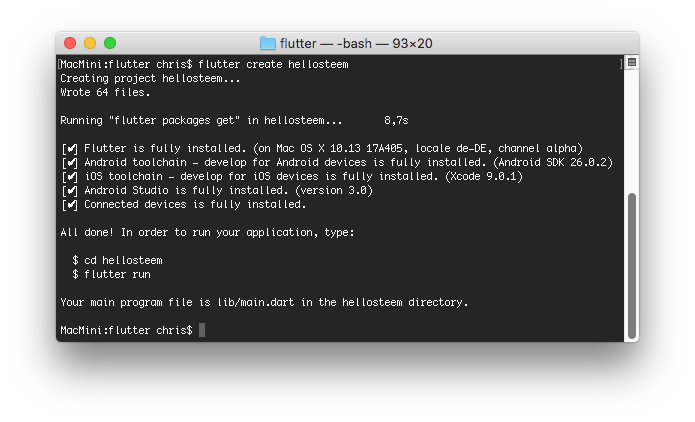
Hitting r
in the terminal where you’ve run flutter run
will do a hot reload, R
(that’s shift+r) will do a full reload. And q
quits the app both on your mobile device/emulator and in the terminal. Another neat trick is pressing p
which will show you the bounds of your widgets, helps learning how the different layout widgets work.
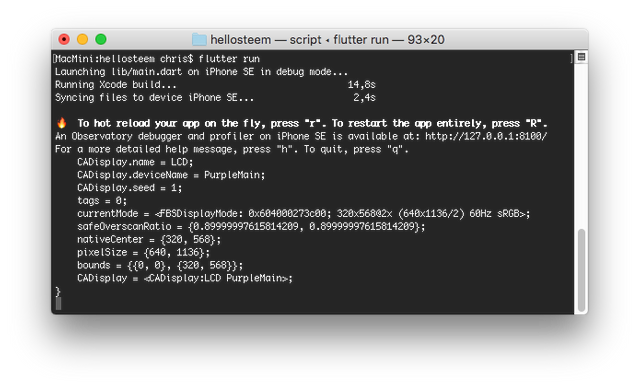
To build an apk for Android that you can install on your phone, just run flutter build apk
, that takes care of everything and the resulting file is 7.4 Mb.
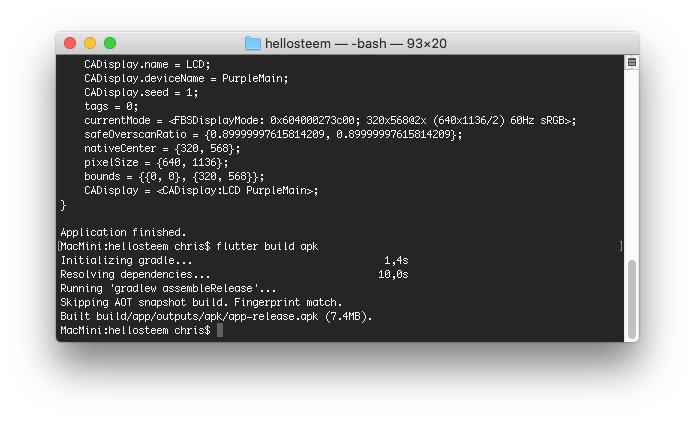
As an IDE, your best options right now are Android Studio (Beta channel, you need at least Android Studio 3.0), Intellij Idea (either Ultimate or Community Edition), WebStorm or Microsoft’s Visual Studio Code with the Dart code plugin.
For the screenshots in this article I’ve been using Microsoft’s Visual Studio Code, it works really well with Flutter and is very lightweight, but use whatever you prefer. Ultimately, Google’s Android Studio will probably become the best option all around, so if you don’t know any of these tools yet, it makes sense to start with that one. For all options, you need to manually install the Flutter plugin, it doesn’t yet come bundled with any of the IDEs (still alpha, like mentioned earlier), but that is an easy automated process in all of the IDEs.
There are also some nice video tutorials about Flutter on YouTube already. I found this series particularly easy to follow (uses Visual Studio Code), it’s nine short videos starting at the very beginning and giving you a good overview of the things you will need to know for basically every app you’d want to develop. You will need to know Dart, but if you already know JavaScript you can learn Dart in an evening, there are tons of video tutorials about Dart programming on YouTube, or just follow Google’s own text tutorials on the Dart website. They’ve got a few codelabs which are really nice and this interactive tutorial (work in progress, 15 lessons so far).
brilliant!! I am going to try to flutter my wings a bit now.
Have fun! :) It works remarkably well for an alpha release and is already so nice to use and get started with. Google is also using it as the framework (and language when it comes to Dart) for their next operating system, called Fuchsia, so what you learn here will also be relevant beyond Android and iOS development for the next generation of mobile and smart home devices.