Why Do People Want to Code in Higher Level Programming Languages?
I haven't posted on Steemit in quite a while, so I decided to do that today with an interesting topic. There are a lot of programming languages out there to use, but what is the real difference? Some is just preference, but sometimes a language will have a real advantage over another. This brings me to my topic...
High vs Low Level Programming Languages
So what do these terms mean? Well, I'll put it this way: high-level is closer to what a human would understand, and low-level is closer to what the computer understands, lowest being binary, or high/low voltage on circuits (high voltage = 1, low voltage = 0). The higher a language goes, the more tasks it does under the hood. When you program in assembly, you are dealing with registers, actual locations in your computer's processor. When you deal with a mid to high level languages, you are most likely dealing with variables. What you might not have realized, is that these variables are actually being stored in registers (and the stack -- the heap if using dynamic allocation, although they will most likely be loaded to registers eventually).
So what is the point of programming is assembly (or even understanding the binary) if it is so much more to deal with? The biggest advantage is just being able to understand how the computer and instructions actually work. Other than that, you may want to go in and edit your assembly code for the sake of speed. This is probably not very likely though; current compilers are extremely good at optimization. Lastly, in extreme cases, you may resort to looking at the assembly code for debugging. If you can think of another reason to program at such a low level, please leave a comment.
Moving on, high level languages are great. They take care of everything you don't need to worry about. The advantages of using these languages are obvious. It is much easier to understand! They may lack a bit in optimization, but in most cases, the difference would be minuscule.
For fun, here is a "Hello World" program in different languages. I had the assembler/compiler convert the same C program into assembly, then into binary. Let me know which ones you would rather interpret!
C
#include <stdio.h>
int main()
{
printf("Hello World!\n");
return 0;
}
Assembly
.file "hello.c"
.section .rodata
.LC0:
.string "Hello World!"
.text
.globl main
.type main, @function
main:
.LFB2:
pushq %rbp
.LCFI0:
movq %rsp, %rbp
.LCFI1:
movl $.LC0, %edi
call puts
movl $0, %eax
leave
ret
.LFE2:
.size main, .-main
.section .eh_frame,"a",@progbits
.Lframe1:
.long .LECIE1-.LSCIE1
.LSCIE1:
.long 0x0
.byte 0x1
.string "zR"
.uleb128 0x1
.sleb128 -8
.byte 0x10
.uleb128 0x1
.byte 0x3
.byte 0xc
.uleb128 0x7
.uleb128 0x8
.byte 0x90
.uleb128 0x1
.align 8
.LECIE1:
.LSFDE1:
.long .LEFDE1-.LASFDE1
.LASFDE1:
.long .LASFDE1-.Lframe1
.long .LFB2
.long .LFE2-.LFB2
.uleb128 0x0
.byte 0x4
.long .LCFI0-.LFB2
.byte 0xe
.uleb128 0x10
.byte 0x86
.uleb128 0x2
.byte 0x4
.long .LCFI1-.LCFI0
.byte 0xd
.uleb128 0x6
.align 8
.LEFDE1:
.ident "GCC: (GNU) 4.1.2 20080704 (Red Hat 4.1.2-55)"
.section .note.GNU-stack,"",@progbits
Binary
01111111 01000101 01001100 01000110 00000010 00000001
00000001 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000010 00000000
00111110 00000000 00000001 00000000 00000000 00000000
11000000 00000011 01000000 00000000 00000000 00000000
00000000 00000000 01000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 01101000 00001010
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 01000000 00000000
00111000 00000000 00001000 00000000 01000000 00000000
00011101 00000000 00011010 00000000 00000110 00000000
00000000 00000000 00000101 00000000 00000000 00000000
01000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 01000000 00000000 01000000 00000000
00000000 00000000 00000000 00000000 01000000 00000000
01000000 00000000 00000000 00000000 00000000 00000000
11000000 00000001 00000000 00000000 00000000 00000000
00000000 00000000 11000000 00000001 00000000 00000000
00000000 00000000 00000000 00000000 00001000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000011 00000000 00000000 00000000 00000100 00000000
00000000 00000000 00000000 00000010 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000010
01000000 00000000 00000000 00000000 00000000 00000000
00000000 00000010 01000000 00000000 00000000 00000000
00000000 00000000 00011100 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00011100 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000001 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000001 00000000 00000000 00000000
00000101 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 01000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 01000000 00000000
00000000 00000000 00000000 00000000 01110100 00000110
00000000 00000000 00000000 00000000 00000000 00000000
01110100 00000110 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00100000 00000000
00000000 00000000 00000000 00000000 00000001 00000000
00000000 00000000 00000110 00000000 00000000 00000000
01111000 00000110 00000000 00000000 00000000 00000000
00000000 00000000 01111000 00000110 01100000 00000000
00000000 00000000 00000000 00000000 01111000 00000110
01100000 00000000 00000000 00000000 00000000 00000000
11101100 00000001 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000010 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00100000 00000000 00000000 00000000 00000000 00000000
00000010 00000000 00000000 00000000 00000110 00000000
00000000 00000000 10100000 00000110 00000000 00000000
00000000 00000000 00000000 00000000 10100000 00000110
01100000 00000000 00000000 00000000 00000000 00000000
10100000 00000110 01100000 00000000 00000000 00000000
00000000 00000000 10010000 00000001 00000000 00000000
00000000 00000000 00000000 00000000 10010000 00000001
00000000 00000000 00000000 00000000 00000000 00000000
00001000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000100 00000000 00000000 00000000
00000100 00000000 00000000 00000000 00011100 00000010
00000000 00000000 00000000 00000000 00000000 00000000
00011100 00000010 01000000 00000000 00000000 00000000
00000000 00000000 00011100 00000010 01000000 00000000
00000000 00000000 00000000 00000000 00100000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00100000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000100 00000000 00000000 00000000
00000000 00000000 00000000 00000000 01010000 11100101
01110100 01100100 00000100 00000000 00000000 00000000
10111000 00000101 00000000 00000000 00000000 00000000
00000000 00000000 10111000 00000101 01000000 00000000
00000000 00000000 00000000 00000000 10111000 00000101
01000000 00000000 00000000 00000000 00000000 00000000
00100100 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00100100 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000100 00000000
00000000 00000000 00000000 00000000 00000000 00000000
01010001 11100101 01110100 01100100 00000110 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00001000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00101111 01101100 01101001 01100010
00110110 00110100 00101111 01101100 01100100 00101101
01101100 01101001 01101110 01110101 01111000 00101101
01111000 00111000 00110110 00101101 00110110 00110100
00101110 01110011 01101111 00101110 00110010 00000000
00000100 00000000 00000000 00000000 00010000 00000000
00000000 00000000 00000001 00000000 00000000 00000000
01000111 01001110 01010101 00000000 00000000 00000000
00000000 00000000 00000010 00000000 00000000 00000000
00000110 00000000 00000000 00000000 00001001 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000001 00000000 00000000 00000000 00000001 00000000
00000000 00000000 00000001 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000001 00000000 00000000 00000000
00100000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00011010 00000000 00000000 00000000
00010010 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
10001100 00000001 00000000 00000000 00000000 00000000
00000000 00000000 00011111 00000000 00000000 00000000
00010010 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
10100101 00000001 00000000 00000000 00000000 00000000
00000000 00000000 00000000 01011111 01011111 01100111
01101101 01101111 01101110 01011111 01110011 01110100
01100001 01110010 01110100 01011111 01011111 00000000
01101100 01101001 01100010 01100011 00101110 01110011
01101111 00101110 00110110 00000000 01110000 01110101
01110100 01110011 00000000 01011111 01011111 01101100
01101001 01100010 01100011 01011111 01110011 01110100
01100001 01110010 01110100 01011111 01101101 01100001
01101001 01101110 00000000 01000111 01001100 01001001
01000010 01000011 01011111 00110010 00101110 00110010
00101110 00110101 00000000 00000000 00000000 00000000
00000000 00000000 00000010 00000000 00000010 00000000
00000000 00000000 00000001 00000000 00000001 00000000
00010000 00000000 00000000 00000000 00010000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
01110101 00011010 01101001 00001001 00000000 00000000
00000010 00000000 00110001 00000000 00000000 00000000
00000000 00000000 00000000 00000000 00110000 00001000
01100000 00000000 00000000 00000000 00000000 00000000
00000110 00000000 00000000 00000000 00000001 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 01010000 00001000
01100000 00000000 00000000 00000000 00000000 00000000
00000111 00000000 00000000 00000000 00000010 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 01011000 00001000
01100000 00000000 00000000 00000000 00000000 00000000
00000111 00000000 00000000 00000000 00000011 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00000000 00000000 00000000 00000000 01001000 10000011
11101100 00001000 11101000 01110011 00000000 00000000
00000000 11101000 11110010 00000000 00000000 00000000
11101000 11001101 00000001 00000000 00000000 01001000
10000011 11000100 00001000 11000011 11111111 00110101
10110010 00000100 00100000 00000000 11111111 00100101
10110100 00000100 00100000 00000000 00001111 00011111
01000000 00000000 11111111 00100101 10110010 00000100
00100000 00000000 01101000 00000000 00000000 00000000
00000000 11101001 11100000 11111111 11111111 11111111
11111111 00100101 10101010 00000100 00100000 00000000
01101000 00000001 00000000 00000000 00000000 11101001
11010000 11111111 11111111 11111111 00000000 00000000
00000000 00000000 00000000 00000000 00000000 00000000
00110001 11101101 01001001 10001001 11010001 01011110
01001000 10001001 11100010 01001000 10000011 11100100
11110000 01010000 01010100 01001001 11000111 11000000
10110000 00000100 01000000 00000000 01001000 11000111
11000001 11000000 00000100 01000000 00000000 01001000
11000111 11000111 10011000 00000100 01000000 00000000
11101000 10111111 11111111 11111111 11111111 11110100
10010000 10010000 01001000 10000011 11101100 00001000
01001000 10001011 00000101 00111001 00000100 00100000
00000000 01001000 10000101 11000000 01110100 00000010
11111111 11010000 01001000 10000011 11000100 00001000
11000011 10010000 10010000 10010000 10010000 10010000
10010000 10010000 10010000 10010000 10010000 10010000
10010000 10010000 01010101 01001000 10001001 11100101
01010011 01001000 10000011 11101100 00001000 10000000
00111101 01010000 00000100 00100000 00000000 00000000
01110101 01000100 10111000 10010000 00000110 01100000
00000000 01001000 00101101 10001000 00000110 01100000
00000000 01001000 11000001 11111000 00000011 01001000
10001101 01011000 11111111 01001000 10001011 00000101
00101100 00000100 00100000 00000000 01001000 00111001
11000011 01110110 00011110 01001000 10000011 11000000
00000001 01001000 10001001 00000101 00011100 00000100
00100000 00000000 11111111 00010100 11000101 10001000
00000110 01100000 00000000 01001000 10001011 00000101
00001110 00000100 00100000 00000000 01001000 00111001
11000011 01110111 11100010 11000110 00000101 00001010
00000100 00100000 00000000 00000001 01001000 10000011
11000100 00001000 01011011 11001001 11000011 00001111
00011111 00000000 01010101 01001000 10000011 00111101
00011111 00000010 00100000 00000000 00000000 01001000
10001001 11100101 01110100 00010110 10111000 00000000
00000000 00000000 00000000 01001000 10000101 11000000
01110100 00001100 10111111 10011000 00000110 01100000
00000000 01001001 10001001 11000011 11001001 01000001
11111111 11100011 11001001 11000011 10010000 10010000
01010101 01001000 10001001 11100101 10111111 10101000
00000101 01000000 00000000 11101000 11110010 11111110
11111111 11111111 10111000 00000000 00000000 00000000
00000000 11001001 11000011 10010000 10010000 10010000
11110011 11000011 00001111 00011111 10000000 00000000
00000000 00000000 00000000 00001111 00011111 10000000
00000000 00000000 00000000 00000000 01001100 10001001
01100100 00100100 11100000 01001100 10001001 01101100
00100100 11101000 01001100 10001101 00100101 10100011
00000001 00100000 00000000 01001100 10001001 01110100
00100100 11110000 01001100 10001001 01111100 00100100
11111000 01001001 10001001 11110110 01001000 10001001
01011100 00100100 11010000 01001000 10001001 01101100
00100100 11011000 01001000 10000011 11101100 00111000
01000001 10001001 11111111 01001001 10001001 11010101
11101000 01111001 11111110 11111111 11111111 01001000
10001101 00000101 01110110 00000001 00100000 00000000
01001001 00101001 11000100 01001001 11000001 11111100
00000011 01001101 10000101 11100100 01110100 00011110
00110001 11101101 01001000 10001001 11000011 10010000
01001000 10000011 11000101 00000001 01001100 10001001
11101010 01001100 10001001 11110110 01000100 10001001
11111111 11111111 00010011 01001000 10000011 11000011
00001000 01001001 00111001 11101100 01110101 11101000
01001000 10001011 01011100 00100100 00001000 01001000
10001011 01101100 00100100 00010000 01001100 10001011
01100100 00100100 00011000 01001100 10001011 01101100
00100100 00100000 01001100 10001011 01110100 00100100
00101000 01001100 10001011 01111100 00100100 00110000
01001000 10000011 11000100 00111000 11000011 10010000
10010000 10010000 10010000 10010000 01010101 01001000
10001001 11100101 01010011 10111011 01111000 00000110
01100000 00000000 01001000 10000011 11101100 00001000
..........
Well, you get the point...
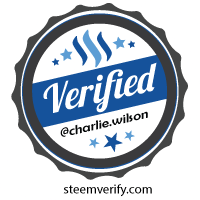
Hi, @charlie.wilson,
Nice explanation and comparison! This ought to help non-coders better understand programming. ;)
😄😇😄
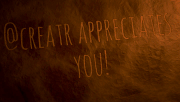
Пирамида )))