One Thing to Know About Javascript
Right now, we can probably all agree that Javascript is the programming language of the future. When it comes to web, it's the language recognized by every browser and there is almost no web page out there that does not use it.
With the rise of single-page applications so the Javascript became more and more popular and whole bunch of new frameworks are coming out every month allowing it's easier implementation.
Node.js created Javascript environment on the server side, many frameworks allow writing desktrop apps, but probably the biggest shift is happening with Ionic and React native that allow writing cross platform native mobile apps using Javascript. This is huge giving the fact that mobile traffic is increasing constantly.
Creating DOM elements
If you are a beginner web designer and you don't know much about Javascript, let me share this quick tip that will benefit you throughout your daily practice.
In every web design project you'll need a way to manipulate the DOM or a page when some event happens. For example, user clicks a button and a dropdown menu slides down, or a clicked tab becomes visible while others hide.
Let's say we have a button
<button>Click me!</button>
and an input element with a class of text
<input type="text" class="text">
We can add couple of styling rules to make it prettier.
button {
height: 30px;
width: 160px;
background-color: #449FBD;
color: white;
border: 1px solid #DDD;
margin-right: 15px;
}
input {
width: 450px;
height: 26px;
padding-left: 4px;
border: 1px solid #DDD;
box-shadow: 0px 2px 8px rgba(0,0,0, .2);
background-color: rgba(0,0,0, .04);
}
Let's now see how we can manipulate this input class with the button, using Javascript. Grasping this simple concept, can open up a whole lot of doors and possibilities for you.
Adding and removing classes
In CSS, we access elements by simply selecting them. In Javascript we do something similar. First of all, we can create a variable called button
to hold the reference to our button element. No fancy ES6 syntax (like using const or let), we'll keep it simple. querySelector
function grabs the first element specified in the brackets. And let's attach event listener with a console message to test if it's working.
var button = document.querySelector('button');
button.addEventListener('click', function() {
console.log('clicked');
});
Let's see how we can control our input by adding and removing class from it. We can create a special class called hidden
that if present, hides the input element from the DOM. Or we can add class dark
to invert it's colors. To do that, let's add new style for the dark input and edit our button event listener from above.
// css
.dark {
background-color: #282F3B;
color: white;
}
// js
button.addEventListener('click', function() {
var input = document.querySelector('.text')
input.classList.toggle('dark');
});
You can see how, in our original HTML markup, we didn't have this dark class, but we were able to add it using Javascript. When the button is clicked, we can do whatever we want.
We can hide it by adding opacity:0
and a subtle transition to our CSS class.
As a web designer, if you only learn this little piece of Javascript, that could be very empowering. By simply selecting an element (we could have used jQuery for this, but there's no need for it in such a small example), attaching event listener to it and then adding and toggling classes, we can manipulate the DOM to meet our needs. You can see examples of this on every web page, where you click on a link and an active class changes, a menu pops up, or search box slides in.
Simple but very powerful concept.
Hope you liked this! If you do, don't forget to upvote and resteem it. Also make sure to follow @alcibiades to stay updated about future posts .
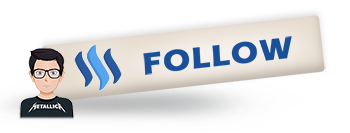
Well written article :) Keep up the good work!
Thanks, glad you liked it! :)
you're right, specially with ES 6 who has many improvements.
Yes, newest versions of Ecmascript have many great built-in features.
The native DOM api can be boring for someone new to JS. Especially if they decided to skip it and jump into jQuery and one of the frameworks like angular, its hard to get back to as it has literally been abstracted out of most of these modern frameworks. But it is an essential knowledge if one wants to become a serious Javascript developer. Always know whats under the hood! Thanks for the post.