SIMPLE TIC-TAC-TOE GAME - LEARNING TO CODE WITH PYTHON Ep 7
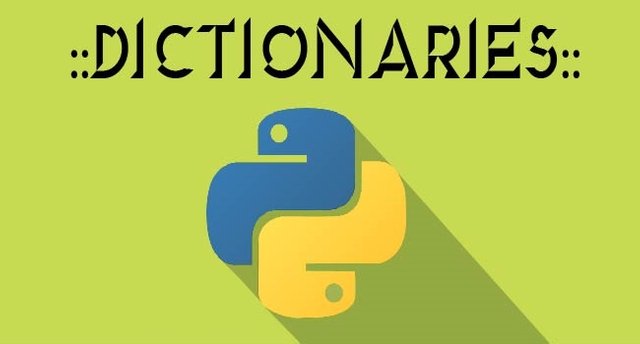
Hello friends, this is a blog about my programming journey, I am basically teaching myself how to code. This is a formal documentation about my progress.

Today we're going to learn about dictionaries and use them to create a basic shell game in python. From the name dictionary, it is a bit self-explanatory. dictionaries consist of words which have meanings. In python, we can create our own custom dictionary, but in python, there is a simple twist, the words are called keys, and the meanings are called values.
In order to know the syntax for creating dictionaries, let us create a simple dictionary called diet, it will contain what we'll have for breakfast, lunch and dinner.
diet = {'breakfast':'eggs and bread' ,
'lunch' : 'rice and chicken',
'dinner' : 'steak and wine'}
The dictionary titled "diet" consists of words(keys) and meanings(values), the keys are (breakfast, lunch, rice), the values of the respective keys are the strings (egg and bread, rice and chicken, steak and wine)
All these data can be stored and edited during program run. for example the dictionary can be edited as follows.
diet[breakfast] = 'beef and noodles'
This single line above can change the breakfast value from "eggs and bread" to "beef and noodles"
diet[lunch] = 'nothing'
This line can also change the value inside the lunch key... following the same syntax we can edit the dictionary as we please.
In order to display the content of our dictionaries, we can just use the print function.
print(diet)
This line of code below can be used to display the values stored in the key-breakfast.
print(diet[breakfast])
There are other methods you can also use to manipulate dictionaries but for the purpose of our basic game, this is the method we'll need.

Create a simple tic-tac-toe game in python

First we will create the dictionary titled "theBoard", this is basically a dictionary that assigns values to the 9 nodes in our tic-tac-toe game. I titled the node from 1-9, each node represents the 9 positions on our game board
theBoard = {'7': ' ', '8': ' ', '9': ' ',
'4': ' ', '5': ' ', '6': ' ',
'1': ' ', '2': ' ', '3': ' '}
Each node-position is a key in our dictionary and the values (X or O) can be stored in any of those keys. I illustrated the numbered node below, we are following this simple grid representation.
But the values stored in those numbered-nodes are empty at the moment.
Next, we will define(def) a function titled "display_board" which displays the game board. It basically consists of print statements that prints each value in the dictionary and spacing them by putting dashes and space-tabs in-between.
def display_board(theBoard):
print(' ', theBoard['7'], ' |',' ' ,theBoard['8'], ' |', ' ',theBoard['9'])
print('-------+--------+--------')
print(' ', theBoard['4'], ' |',' ' ,theBoard['5'], ' |', ' ',theBoard['6'])
print('-------+--------+--------')
print(' ', theBoard['1'], ' |',' ' ,theBoard['2'], ' |', ' ',theBoard['3'], '\n')
Each time we call the function above, it displays the values in our dictionary, similar to this.
Next we will define(def) another function(titled "instruction") that print some instructions to the user, It consists of print statements that instructs the user on what keys to press on the keyboard to place the (X or O)
def instruction():
print("press the numbers to place your X or O in position\n")
print(' ', '7', ' |',' ' ,'8', ' |', ' ','9')
print('-------+--------+--------')
print(' ', '4', ' |',' ' ,'5', ' |', ' ','6')
print('-------+--------+--------')
print(' ', '1', ' |',' ' ,'2', ' |', ' ','3', '\n')
print('=========================\n')
If we run this function above it displays a prompt like this.
Now we'll get to the real meat of the program, we'll define a function(check_winner) that checks the value of each keys in our dictionary, if any 3 (X or O) forms a straight line a winner is found. we will create several if statements which checks each numbered keys(in 3 groups) that are equal to each other meaning they are in a straight line, for example the keys(7, 4 and 1) are in a straight line. we will repeat the same pattern for all the win outcomes.
def check_winner(theBoard):
check = 0
if theBoard['7'] == theBoard['8'] == theBoard['9'] != ' ' :
check = 1
elif theBoard['7'] == theBoard['4'] == theBoard['1'] != ' ':
check = 1
elif theBoard['7'] == theBoard['5'] == theBoard['3'] != ' ':
check = 1
elif theBoard['4'] == theBoard['5'] == theBoard['6'] != ' ':
check = 1
elif theBoard['1'] == theBoard['2'] == theBoard['3'] != ' ':
check = 1
elif theBoard['1'] == theBoard['5'] == theBoard['9'] != ' ':
check = 1
elif theBoard['9'] == theBoard['6'] == theBoard['3'] != ' ':
check = 1
elif theBoard['2'] == theBoard['5'] == theBoard['8'] != ' ':
check = 1
return check
Each if-statements and consecutive elif-statements in the code above uses the mathematical python syntax which check if 3-straigh-line nodes are equal(==) to each other and are not empty (!=' ').
Also the logic behind this function is to use a basic check method. the variable (check) is given a default value of 0 but if any if-statement is true, check is changed to 1. at the end of the function the value of check is returned. if check is 0 then there is no winner but if check is equal to 1 then a winner has been found.
We'll now define our final function(titled "play"), play will basically consist of our game play codes which will change the turns of each player, call on the other previously defined functions, and also confirm our winner, I will try to explain each line of the code below. The explanation would be lengthy but I must break it down for my further understanding and yours.
- def play(theBoard):
- node = "X"
- for i in range(9):
- instruction()
- display_board(theBoard)
- print('it is ', node, 'turn to play \n')
- turn = input()
- while True:
- if theBoard[turn] == ' ':
- theBoard[turn] = node
- break
- else:
- print("you've already played that move, place your", node, "elsewhere\n")
- instruction()
- display_board(theBoard)
- turn = input()
- check = check_winner(theBoard)
- if check == 1:
- print('The winner is', node)
- break
- else:
- print("No winner, play again?")
- if node == 'X':
- node = 'O'
- else:
- node = 'X'
line 2
At the beginning of the "play" function. The node will be equal to X meaning the first player will use the first-X-node. This node will be changing between X and O several times in the program.line 3
A basic for loop is initated to run 9-times because the both players gets a total of 9 turns in the game, there are only 9 positions to the played.line 4
Inside the for loop, we will first call the instruction() and display_board(theBoard) function which were defined earlier in the code. the purpose of these function is to display the instruction and the current-game-board within the 9-times-loop. the game board will keep displaying and updating throughout the game play.line 5
Next is a simple print-statement(display prompt that shows the current player-node) followed by an input function that accepts the numbered-keys between(1-9) from the user and stored in the variable(turn), each of these numbers have a positional value in our dictionary-grid as explained earlier.line 8
When one of the players fufil his/her turn and stores an(X or O) value in the dictionary. we do not want another user to override his play and input into a filled spot. so we will create an infinite loop with the (while True) statement. infinite loops are used when we are uncertain about the number of times or loops a user would perform a task. since we do not know how many times the user would make this mistake, this infinite loop would contain a condition that would break out of the loop when the user performs the right task- putting the(X or O) into an empty spot.line 9
Inside the while True infinite loop, it contains an If-statement which checks if the position on the board is empty. If it is empty it stores the X-node into the postition(turn) entered by the user, and breaks out of the loop in the next line.line 12
Another provision is made in form of an else-statement. if the position is already filled, the else-statement-code block is activated, it instruct the user about the mistake, displays the current board with the pre-defined functionss and accept a new input for the variable(turn), the variable will keep testing against the dictionary in the infinite-loop, if the the dictionary-position is occupied the loop will keep running.line 17
The predefined check_winner function is called and checks for the winner. the value(1 or 0) returned from the function is stored in the variable(check).line 18
The if statement checks if the returned value is 1. if (check = 1) then it runs the if-code-block which declares the winner and breaks out of the 9-times for-loop in line 3. If the value of check is not 1, the else-statement is activated- which declares the there is no winner.line 23
This block of if and else statement toggles the turn between X and O.At last the functions are called in the main program.
play(theBoard)
display_board(theBoard)
The play function runs the game play and the display_board function displays the board at the end of the game.
Example of game running below:
That is all for today, thanks for visiting my blog
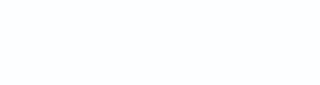

Congratulations! This post has been upvoted from the communal account, @minnowsupport, by abmakko from the Minnow Support Project. It's a witness project run by aggroed, ausbitbank, teamsteem, someguy123, neoxian, followbtcnews, and netuoso. The goal is to help Steemit grow by supporting Minnows. Please find us at the Peace, Abundance, and Liberty Network (PALnet) Discord Channel. It's a completely public and open space to all members of the Steemit community who voluntarily choose to be there.
If you would like to delegate to the Minnow Support Project you can do so by clicking on the following links: 50SP, 100SP, 250SP, 500SP, 1000SP, 5000SP.
Be sure to leave at least 50SP undelegated on your account.
Interesting. So, is "dictionary" python's actual term or just your own? I mean, in php or java, those would be arrays. Never really did get into learning python yet, though I have seen some of its syntax before when it's used for graphing. From your codes, I find that python's else if (elif) sounds cute. Haha.
In my limited understanding of Python, I think it is more like objects in JavaScript (probably PHP and Java too, but I'm not completely familiar with them). They contain multiple pieces of information stored in key:value pairs.
Dictionary is a python term... python also have lists... which is more similar to arrays. but dictionary is a more versatile way of storing data in an unordered format. thanks for visiting my blog @kdee916
I'm really enjoying these posts @abmakko. Python is a great language. So versatile and the syntax is super clean without a lot of the punctuation many other languages have.
Yeah... Python is very though on indentations and code neatness. a simple misplaced space bar can trigger an error. thanks for following my progress. @steveblucher.
That is pretty simple compared to the C# tutorial for one I did, but that also was much more in-depth.
Python syntax is quite simple compared to other programming languages... Thanks for the compliment
Any advantage to using a dictionary over a list of lists?
Proud member of #steemitbloggers @steemitbloggers
I think it depends on the application and the task you are running. for this simple game I don't need to order my data, because I am mostly retrieving and storing data in different and unpredictable positions, so dictionary is much more neat for me. and also in my opinion dictionary is faster in retrieving data because it does not need to scan through in an ordered fashion. but lists also have its advantages over dictionaries, It all boils down to the application.
Thanks for passing by :-)
Wow, I haven't needed to program anything since my university days... I keep meaning to get back and learn Python, but family life means I'm a little on the time restricted side of things... Seeing this post does give me a renewed purpose though!
I am glad that you are motivated to pursue this. A few hours a day can go a long way.. thanks for visiting my blog :-)
This reminds me of one of the things I did when I was learning how to create databases in MBasic - I put together a program so I could keep track of my records. I had a few hundred albums (yes, vinyl - lol) back in the day, and some of my collection went with me to college, some friends borrowed others, etc. I found creating the program went a long way towards helping me figure it out, much more so than simply reading about it. Another excellent lesson, @abmakko!
Thanks for passing by @traciyork... automating tasks with programming can go a long way in easing our workload
You're welcome and I completely agree!
I attended a programming course at school but quickly understood that was not for me :D It's just too difficult to grasp for me.
I'm amazed that you're learning it yourself and it looks like you're good already! :)