JavaScript Programming Pt2 (Variables and Statements)
Variables and Statements
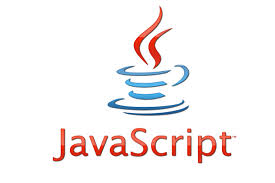
Source
Javascript Programming Part One
I have done my best to up my game here a little bit so I will be providing this second part of this exploration in two parts; video format and written(text and images)
Video
Written and Images
Here we are going to cover defining a number of different types of variables:
Please for the time being ignore the stuff that is greyed out, we will talk about adding comments which is what those all are right now.
When we are defining variables in javascript there are basically three parts to doing so:
"var" tells javascript that we want to define the variable, the next piece we define the name which is fairly arbitrary we can really name our variable anything we like, however it is a good idea to name it something that makes sense to us.
in the line var number1 = 12;
we tell javascript that we want that to have the value of 12 and then we finalise the statement with a semicolon ;
each of these lines allow us to define the variables in slightly different ways, for examples surrounding a string of text with "" allow us to define our variable as a string.
var string1 = "This is a string.";
Here we do exactly that.
Take a look at each of these and try console logging each.
If you are having trouble reading the yellow text in firefox you can change the theme by going into the toolbox on the right hand side where the gearbox is.
Alright we should now have some handle on how to define a variable and assign it some value.
An "IF" Statement
Alright so whats going on here? Well lets take a look at what the browser has to say about it and then we can talk about that, so save the file and then refresh the file in the browser and you should see something along these lines:
Our console logged "true"
Ok there are a couple pieces of an if statement to understand the first is of course if next we define our arguments or argument in the case of both of these if statements we are only going to play with one argument. That argument in the first if statement can be found inside the brackets () and it is bool2, now if we remember we defined that as a variable at the top of our script and when we did so we set it with a value of false. For the if statement to complete we need to pass it an argument that is true so for the first if state it is not going to console log because the argument is false.
Lets look at the second if statement where the argument is bool, at the top of our script we defined that variable as well and when we did so we gave it a value of true, that means that the second if statement is going to pass and the code that we put inside its curly {} brackets is going to run, that is why we see true in the console on the browser because we told it to console log bool if it passes.
Moving along...
A "FOR" Statement
Lets talk about what is happening here now that we have had a look. A for statement is pretty fun and can be used for a fair amount.
A for statement to define it is a little more complex then an if statement, but in simplicity we could think of making a statement in the english language of "For something if true continue doing this until it isn't." I hope that makes sense but lets break this down further and go through the whole process.
for(var loopcount = 0; loopcount < forest.length; loopcount++){
console.log("Current type is: " + forest[loopcount].type);
};
for(var loopcount = 0;
This is the first part of our statement and here we define the variable that we want our for loop to run based on and set it to zero.
loopcount < forest.length;
This is what we run the loop based on here we see if loopcount is less then forest.length if we console log forest.length we will see the result is the length of forest which has a bunch of entries in it.
loopcount++)
This is the last piece of the for statement itself here we just tell javascript to add one to loopcount each time it goes through.
{
console.log("Current type is: " + forest[loopcount].type);
};
Immediately after that we need to tell the for loop what we want to do while the loop is running.
And that is about it we have now gone through how these work and I hope this has been helpful.
Javascript Programming Part One