[Piston-API] Tutorial - Make a vote and post on your own app using Python
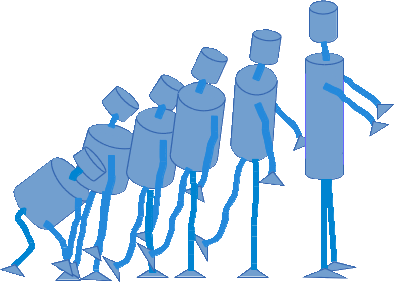
Introduction
Thanks to @xeroc who have made python library for steem.
There are several python libraries on the github but this is one of well-wrapped library from the others. Especially, you don't need to dig into the JSON structures for RPC when you begin - maybe you need it later, though. Also he provides end point server on public!
The server address is as below:
wss://this.piston.rocks
The library is well documented, but I think code example helps to understand and to attract more python based developers.
Requirements
- Python3
Install the library
You can install it through pip. As below.
pip3 install steem-piston
Connect to end point server
If you just want test, you can use that piston rocks server as default setting. And you should set posting private key for post and vote as a parameter in the constructor. (You can easily find at Permissions)
from piston.steem import Steem
steem = Steem(wif='posting private key') # using default server
Or if you have own end point,
steem = Steem(node='Your end point(ex. ws://..)', wif='posting private key')
Let's cast a vote
Just put author and permlink of the post.
steem.vote(identifier = '@author/permlink', weight=100.0, voter='Your Steemit Nickname')
# if weight is under 0, will be downvote
That's it.
So, entire code would be as follows:
from piston.steem import Steem
steem = Steem(node='Your end point(Optional)', wif='posting private key'
steem.vote(identifier='@author/permlink', weight=100.0, voter='Your Steemit Nickname')
Then, how to write a post?
These are the only things you need to write a post: Title, Contents, Your Steemit Nickname, Category.
No more. Same with when you write a post on the website, right?
Let me write a well-known programming idiom.
steem.post(title='Piston API test', body='Hello, World!', author='Your Steemit Nickname',
category='category') # I recommend using "spam" category for the test
Entire code would be:
from piston.steem import Steem
steem = Steem(node='Your end point(Optional)', wif='posting private key')
steem.post(title='Piston API test', body='Hello, World!!', author='Your Steemit Nickname',
category='category')
And, you can write a comment also using post function. Because comments are treated as a titleless sub post.
from piston.steem import Steem
steem = Steem(node='Your end point(Optional)', wif='posting private key')
steem.post(title='', body='Annyeong, I\'m a bot.', author='Your Steemit Nickname',
reply_identifier='@author/permlink of post')
Multiplication Robot
Let's mix them all.
from piston.steem import Steem
import random
username = 'robot'
key = 'arbitrary_private_key'
i = random.randrange(1, 10) # make random number between 1 ... 9
post_title = 'This is multiplication table of ' + str(i)
payload = ''
tag = 'spam'
for j in range(1, 10):
payload = payload + str(i) + ' X ' + str(j) + ' = ' + str(i * j) + '<br>'
steem = Steem(wif=key)
# Writing a post
steem.post(title=post_title, body=payload, author=username, category=tag)
# Upvoting this post
steem.vote(identifier='@robot/this-is-multiplication', weight=100.0, voter=username)
# Leaveing a Comment
steem.post(title='', body='Oh, You\'re clever.', author=username,
reply_identifier='@robot/this-is-multiplication')
# Yes, I know it's quite dirty code.
So, you can build a bot or AlphaGo for Steem whatever you want.
Let's access blocks directly and utilize it next.
it is always worth to mention, to use latest version of steem-piston. It is develop very quickly! :)
Yes You're right.
Thanks for this!