How to easily create an Google Amp site in 9 steps using PHP
How to easily create an Google Amp site in 9 steps using PHP
The goal is to create a site easily, fast,without dependencies and without sacrify performance.
Ingredients
- Some knowledge on PHP and CSS.
- PHP installed 5.6+ or higher. (free)
- Mysql Server (optional, free)
- Some images and content.
- 20 minutes and only 9 steps (or 10 if you consider to edit your canonical web).
- AMPGeneratorOne library(free, open source)
https://github.com/EFTEC/AMPGeneratorOne
- A text editor. I recommend Visual Studio Code (free) or PHPStorm (paid).
- A browser and an AMP extension. I use “AMP Browser Extension” (Firefox and Chrome)
Directions
A- Creating the barebone.
- Create an empty folder inside your website; maybe the folder could be called amp.
- Download AMPGeneratorOne, you could use Composer or download directly in some folder. You only need the file AmpGeneratorOne.php. I downloaded in the folder lib. I also created the folder resources and the folder resources/logo. The folders are only are recommendation
amp/
amp/lib/ (AmgGeneratorOne goes here)
amp/resources/ (images goes here)
amp/resources/logo/ (logo images goes here) - Let’s create the initial code. I called landing.php that is the landing page.
<?php
use eftec\AmpGeneratorOne\AmpGeneratorOne;
use eftec\AmpGeneratorOne\ButtonModel;
use eftec\AmpGeneratorOne\FooterModel;
use eftec\AmpGeneratorOne\HeaderModel;
use eftec\AmpGeneratorOne\HeadModel;
use eftec\AmpGeneratorOne\LinkModel;
use eftec\AmpGeneratorOne\SectionModel;
use eftec\AmpGeneratorOne\StructureModel;
include "lib/AmpGeneratorOne.php";
$canonical="https://www.canonical.com";
$base='http://localhost/currentproject/ampgeneratorone/example/MediumExample';
$amp=new AmpGeneratorOne($canonical,$base);
$amp->startAmp(new HeaderModel("Example","AMP Generator One"));
// sidebar menu
$menu=array();
$menu[]=new LinkModel("Cupcakes", $base."/detail.php?id=cupcake", "");
$menu[]=new LinkModel("Muffins", $base."/detail.php?id=muffin", "");
$amp->sidebar($menu);
$amp->head(new HeadModel("Cupcakes","/resources/logo/logo.png"),70,70);
$amp->render();
Explanation
$canonical="https://www.canonical.com";
$base='http://localhost/currentproject/ampgeneratorone/example/MediumExample';
$canonical is your original non-amp page.
$base is where your amp will be located. It could change!. After all, Google may cache your page, so the location could be different.Let’s say that your site is called www.mysite.com and your amp is located at www.mysite.com/amp then:
$canonical="https://www.mysite.com";
$base='http://www.mysite.com/amp';
B- Let’s start with the constructor
$amp=new AmpGeneratorOne($canonical,$base);
$amp->startAmp(new HeaderModel("Example","AMP Generator One"));
- Example is the title of the page.
- AMP Generator One is the description. Search engines use the description so it is essential.
C- Sidebar
We created a list of links (the first value is the text, the second is the link. The link could be everything. In this case, it points to detail.php that it’s not yet created.
// sidebar menu
$menu=array();
$menu[]=new LinkModel("Cupcakes", $base."/detail.php?id=cupcake", "");
$menu[]=new LinkModel("Muffins", $base."/detail.php?id=muffin", "");
$amp->sidebar($menu);
D- Head
(the upper “menu”). The name of the site is called “Cupcakes”, I also added a logo. The size of the logo is 70x70 pixels.
$amp->head(new HeadModel("Cupcakes","/resources/logo/logo.png"),70,70);
Z- Render (final step)
And finally, we render (show) the page
$amp->render();
Modifiers
Right now, there are three modifiers:
- setClassTextColor(class) you could change the color of the text using a css class. It doesn’t include the text of the head and slider, the could be changed in the constructor.
$amp=new AmpGeneratorOne($canonical,$base,"red","blue","text-black"); // where red is the background color of the sidebar, blue is the color of the text of head and burger (menu) and text-black is the class of the color of the sidebar.
- setBgImage(image) you could change the background image
- setBackgroundColor(color) you could change the background color.
- setPadding(top,botton) you could change the padding of the container. You couldn’t change the padding of left and right.
The modifier could be stacked. For example
$amp->setClassTextColor(‘text-white’)
->setBackgroundColor(‘black)
->…
B- Constructor (revisited)
Let’s modify the constructor and add default colors.
$amp=new AmpGeneratorOne($canonical,$base,"#ECE9C7","white","text-black");
$amp->setDefault("#FC9D9A","text-white");
Where
- #ECE9C7 the background color of the sidebar
- white Is the color of the title, burger and X close slider
- text-black is the class of text of the slider
- #FC9D9A is the default background color
- text-white is the default class color of the text
without the slider
with the slider open
E- First section
$amp->sectionFirst(new SectionModel("Cupcakes"));
Yep, it’s fine but the color is the same than the head. Let’s add a modifier
$amp->setBackgroundColor("#F9CDAD") // change background
->sectionFirst(new SectionModel("Cupcakes"));
$amp->setBgImage("resources/pexels-photo-585581.jpeg") // change background image
->setClassTextColor("text-black") // change text color
->sectionFirst(new SectionModel("Cupcakes"));
F- More sections
Let’s add more stuff
- a section with two buttons
- a section with a single text and description
- a section with images
//second buttons
$sec2=new SectionModel();
$sec2->buttons[]=new ButtonModel("Button1","#");
$sec2->buttons[]=new ButtonModel("Button2","#","danger");
$amp->setBackgroundColor("white")
->sectionButtons($sec2);
// section text
$sec3=new SectionModel("Cupcakes","The earliest extant description of what is now often called a cupcake was in 1796");
$amp->setBackgroundColor("white")
->setClassTextColor("text-black")
->sectionText($sec3);
// section images
$images=[];
$images[]=new SectionModel("Cupcake","The earliest extant description of what is now often called a cupcake was in 1796","/resources/cupcake_square.jpg");
$images[]=new SectionModel("Cupcake","The earliest extant description of what is now often called a cupcake was in 1796","/resources/cupcake_square.jpg");
$images[]=new SectionModel("Cupcake","The earliest extant description of what is now often called a cupcake was in 1796","/resources/cupcake_square.jpg");
$amp->setBackgroundColor("white")
->setClassTextColor("text-black")
->sectionColImage($images,400,400,3); // 3 indicates the number of columns.
There are several section to play, including google analytics, images with text, google maps and such
G-Navigation and footer
// # navigation
$sbnav=new SectionModel("Cupcakes","The earliest extant description of what is now often called a cupcake was in 1796");
$sbnav->buttons[]=new ButtonModel("primary","#","primary");
$navCol1=[];
$navCol1[]=new LinkModel("Cupcakes","#","",true);
$navCol1[]=new LinkModel("Easy Little Pandas","#");
$navCol1[]=new LinkModel("Apple Pie Cupcakes","#");
$navCol1[]=new LinkModel("Chocolate","#");
$navCol2=[];
$navCol2[]=new LinkModel("Muffins","#","",true);
$navCol2[]=new LinkModel("Apple-Cinnamon","#");
$navCol2[]=new LinkModel("Banana","#");
$amp->setBackgroundColor("black")->setClassTextColor("text-white")->sectionNavigation($sbnav,"",0,0,$navCol1,$navCol2);
// #5 footer
$amp->setPadding(0,0)
->sectionFooter(new FooterModel("Copyright something(c)","See as desktop"));
H-Closing and packing
You should add a link to your amp site. It must be added in the header of the canonical (non amp) site.
<link rel="amphtml" href="https://www.mysite.com/amp/" />
Final project (landing.php)
<?php
use eftec\AmpGeneratorOne\AmpGeneratorOne;
use eftec\AmpGeneratorOne\ButtonModel;
use eftec\AmpGeneratorOne\FooterModel;
use eftec\AmpGeneratorOne\HeaderModel;
use eftec\AmpGeneratorOne\HeadModel;
use eftec\AmpGeneratorOne\LinkModel;
use eftec\AmpGeneratorOne\SectionModel;
use eftec\AmpGeneratorOne\StructureModel;
include "../../lib/AmpGeneratorOne.php";
$canonical="https://www.canonical.com";
$base='http://localhost/currentproject/ampgeneratorone/example/MediumExample';
$amp=new AmpGeneratorOne($canonical,$base,"#ECE9C7","white","text-black");
$amp->setDefault("#FC9D9A","text-white");
$amp->startAmp(new HeaderModel("Example","AMP Generator One"));
// sidebar menu
$menu=array();
$menu[]=new LinkModel("Cupcakes", $base."/detail.php?id=cupcake", "");
$menu[]=new LinkModel("Muffins", $base."/detail.php?id=muffin", "");
$amp->setClassTextColor("text-white")
->sidebar($menu);
$amp->head(new HeadModel("Cupcakes","/resources/logo/logo.png"),60,60);
// first section
$amp->setBgImage("resources/pexels-photo-585581.jpeg")
->setClassTextColor("text-black")
->sectionFirst(new SectionModel("Cupcakes"));
//second buttons
$sec2=new SectionModel();
$sec2->buttons[]=new ButtonModel("Button1","#");
$sec2->buttons[]=new ButtonModel("Button2","#","danger");
$amp->setBackgroundColor("white")
->sectionButtons($sec2);
// section text
$sec3=new SectionModel("Cupcakes","The earliest extant description of what is now often called a cupcake was in 1796");
$amp->setBackgroundColor("white")
->setClassTextColor("text-black")
->sectionText($sec3);
// section images
$images=[];
$images[]=new SectionModel("Cupcake","The earliest extant description of what is now often called a cupcake was in 1796","/resources/cupcake_square.jpg");
$images[]=new SectionModel("Cupcake","The earliest extant description of what is now often called a cupcake was in 1796","/resources/cupcake_square.jpg");
$images[]=new SectionModel("Cupcake","The earliest extant description of what is now often called a cupcake was in 1796","/resources/cupcake_square.jpg");
$amp->setBackgroundColor("white")
->setClassTextColor("text-black")
->sectionColImage($images,400,400,3);
// # navigation
$sbnav=new SectionModel("Cupcakes","The earliest extant description of what is now often called a cupcake was in 1796");
$sbnav->buttons[]=new ButtonModel("primary","#","primary");
$navCol1=[];
$navCol1[]=new LinkModel("Cupcakes","#","",true);
$navCol1[]=new LinkModel("Easy Little Pandas","#");
$navCol1[]=new LinkModel("Apple Pie Cupcakes","#");
$navCol1[]=new LinkModel("Chocolate","#");
$navCol2=[];
$navCol2[]=new LinkModel("Muffins","#","",true);
$navCol2[]=new LinkModel("Apple-Cinnamon","#");
$navCol2[]=new LinkModel("Banana","#");
$amp->setBackgroundColor("black")->setClassTextColor("text-white")->sectionNavigation($sbnav,"",0,0,$navCol1,$navCol2);
// #5 footer
$amp->setPadding(0,0)
->sectionFooter(new FooterModel("Copyright something(c)","See as desktop"));
$amp->render();
Nutritional Facts
Why AMP Site?.The AMP Project is an open-source initiative aiming to make the web better for all. The project enables the creation of websites and ads that are consistently fast, beautiful and high-performing across devices and distribution platforms.
Hello! Your post has been resteemed and upvoted by @ilovecoding because we love coding! Keep up good work! Consider upvoting this comment to support the @ilovecoding and increase your future rewards! ^_^ Steem On!
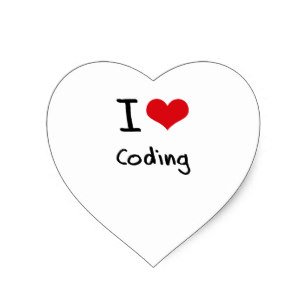
Reply !stop to disable the comment. Thanks!
Congratulations @jorgecastro! You received a personal award!
You can view your badges on your Steem Board and compare to others on the Steem Ranking
Vote for @Steemitboard as a witness to get one more award and increased upvotes!