[Python #4] Learning Python with Selenium - Function [KR]
함수를 알아 본다. 반복적으로 사용하는 코드를 함수로 묶어서 생성해 놓으면 필요할 때마다 그 함수를 호출하기만 하면 된다.
반복적으로 사용하는 코드를 계속 복사붙여넣기로 사용할 수는 없는 노릇이다. 유지보수가 힘들뿐더러 코드가 더러워진다.
파이썬의 함수는 java 의 메서드와 비슷한 개념이지만 java 에 비해 작성 방식이 훨씬 자유롭다.
type을 지정하지 않아도 된다는게 가장 큰 장점인 것 같다. 코드를 짧게 작성할 수 있기 때문이다.
아래, 리스트를 인자로 받아 Object 타입으로 리턴하는 함수를 java와 비교해 본다.
java:
public Object a(List<Object> name){
... 블라블라 ...
return object
}
python:
def a(name):
... 블라블라 ...
return object
이거슨... 너무 간단하지 아니한가?
파이썬이 훨씬 자유도가 높은 것 같다. java 가 아이폰라면, 파이썬은 안드로이드 느낌이랄까~
다만 이런 작성 방법은 권장하지 않는다고 공식적으로 언급된 것 같다. 함수가 동작은 할지라도 가독성이 떨어지기 때문이다.
또한 함수를 호출하기전까지는 그 함수의 인자 유형이나, 리턴 유형을 알기 어렵다.
다행히 파이썬의 어노테이션이라는 개념으로 유형을 문서화 할수 있다. 어노테이션을 추가하는 방법도 매우 간단하다.
함수 첫행에 추가하면 되는데 파라미터 타입은 파라미터 뒤에 ': 타입'을 추가하면 되고,
리턴 유형은 괄호 뒤에 '-> 타입'을 추가하면 된다. 리턴 함수가 아닐 경우 '-> None'을 추가한다.
위 파이썬 예를 수정하면 아래와 같다.
def a(name: list) -> object:
... 블라블라 ...
return object
그리고 또 하나는 java 의 오버로딩과 같은 개념이 파이썬에는 없다. (다른 개념의 오버로딩은 있는 듯 하나 거기까지는 아직이다.)
동일 이름의 메서드이지만 파라미터 개수가 다를 경우 Java에서는 동일 이름의 메서드를 여러개 만들어야 한다.
파이썬은 그럴 필요가 없다. 하나의 함수에 여러개 파라미터를 정의한 후 호출할 때 인자만 조절하면 된다.
Source
from selenium import webdriver
import time
from selenium.common.exceptions import NoSuchElementException
import pprint
def login():
"""
log in to steemit
"""
# Click the login button
chrome.find_element_by_xpath('//Header/descendant::a[text()="로그인"]').click()
# Enter username
chrome.find_element_by_xpath('//input[contains(@name,"username")]').send_keys('june0620')
# Get posting key from file
with open('C:/Users/USER/Desktop/python/june0620.txt') as pw:
for postingKey in pw:
# Enter posting key
chrome.find_element_by_xpath('//input[contains(@name,"password")]').send_keys(postingKey)
# Click the login button
chrome.find_element_by_xpath('//button[text()="로그인"]').click()
# Waiting for N seconds
time.sleep(3)
def vote():
pass
def is_nsfw(post: webdriver) -> bool:
"""
Check if it is nsfw.
:param post: Post element
"""
try:
post.find_element_by_xpath('descendant::span[text()="nsfw"]')
return True
except NoSuchElementException as err:
return False
def posts_info_list(posts: webdriver) -> list:
"""
Return the information in the posts.
"""
info_list = []
for post in posts:
post_info = {
"title": post.find_element_by_xpath('descendant::h2').text,
'url': post.find_element_by_xpath('descendant::h2/a').get_attribute('href'),
'username': post.find_element_by_xpath('descendant::span[@class="author"]/strong').text,
'reputation': post.find_element_by_xpath('descendant::span[@class="Reputation"]').text,
'post_date': post.find_element_by_xpath('descendant::span[@class="updated"]').text,
'upvoting_status': post.find_element_by_xpath('descendant::span[@class="Voting__inner"]/span[1]').get_attribute('class'),
'rewards': post.find_element_by_xpath('descendant::span[@class="FormattedAsset "]').text
}
info_list.append(post_info)
return info_list
오늘의 내용 정리
Python:
- 함수는 def로 시작하고 :로 마무리한다. 함수 안의 코드를 스위트라고 하는데 스위트는 꼭 들여쓰기 해야 한다.
- 파이썬 함수는 간단하게 작성 가능하지만, 가급적이면 어노테이션과 docstring(설명)을 추가하도록 한다. docstring은 스위트 첫행에 ''' ''' 주석처리 후 안에 설명을 기입하면 된다. (캡쳐 이미지 녹색 부분)
- 공함수는 pass 키워드로 만들 수 있다. 공함수는 임시용이고 공함수가 있어서는 당연히 안되겠다. (캡쳐 이미지 vote 함수)
pycharm:
- 함수의 어노테이션과 docstring 조회 단축키는 ctrl + Q 이다.
Reference:
[Python #3] Learning Python with Selenium - Dictionary [KR]
Thank you so much for participating in the Partiko Delegation Plan Round 1! We really appreciate your support! As part of the delegation benefits, we just gave you a 3.00% upvote! Together, let’s change the world!
!shop
@tipu nominate
@tipu curate
항상 행복한 💙 오늘 보내셔용~^^
Posted using Partiko Android
감사합니다^^
행복한 저녁 보내셔요^^
!shop
Posted using Partiko Android
Hi~ bluengel!
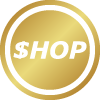
@june0620 has gifted you 1 SHOP!
Currently you have: 42 SHOP
View or Exchange
Are you bored? Play Rock,Paper,Scissors game with me!SHOP
Please go to steem-engine.com.Rock
Posted using Partiko Android
You win!!!! 你赢了! 给你1枚SHOP币!
你好鸭,june0620!
@bluengel给您叫了一份外卖!
由 @honoru 米高 迎着台风 跑着步 给您送来
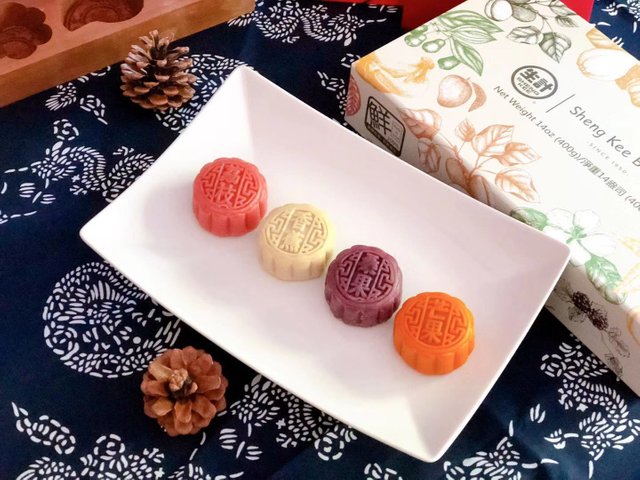
水果月饼
吃饱了吗?跟我猜拳吧! 石头,剪刀,布~
如果您对我的服务满意,请不要吝啬您的点赞~
@onepagex
没有tipu么
@tipu curate 2
Posted using Partiko Android
Upvoted 👌
非常感谢 😃😀
Posted using Partiko Android
不用谢呀☺️
几日不见帅锅正好看到呢😁
!shop
Posted using Partiko Android
Hi~ june0620!
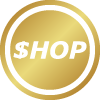
@annepink has gifted you 1 SHOP!
Currently you have: 45 SHOP
View or Exchange
Are you bored? Play Rock,Paper,Scissors game with me!SHOP
Please go to steem-engine.com.