Python Programming Tutorial - How To Make Multi-Threaded Application (I.E. Background Processes/Asynchronous Processes)
Multi-Threading Applications in Python
Hi everyone, I'm writing this tutorial in hopes to get more people interested in Python, the fast scripting language that has no need for declarations for variable types and is clean and easy to learn. For reference, steemit doesn't allow multiple spaces or "indents", so Hyphens, the "----" symbol, means 4 spaces, or 1 tab in a text file, Blank Space! Very important!
This will be the simplest guide on how to make a multi-threaded program/script in Python 2.7. (This will not work in Python 3.5, they use a different module to get these same functions, so do not use this with 3+)
What is Multi-Threading?
A thread is a single process being executed that is linear but stand-alone, being called into being and occupying background processing time. This means you can run multiple functions simultaneously, or just two during the same time period whatsoever . So you can, for instance, run two timers at the same time that print different things at different intervals.
This allows you to also take advantage of multiple cores, virtual cores, and hyper-threading, which all make these "threads" run more efficiently.
So How Do We Code This?
Begin by importing into your python script threading from the Thread module, and the Time module, like so:
from threading import Thread
import time
Threading from Thread module lets you access the native python multi-threading module class threading, so we can create thread objects.
Then we create our function to implement threads & time appropriately, lets start by creating a "Timer" function that will print the time every X seconds Y times:
def timer(name, delay, repeat):
----print "Timer: " + name + " started."
----while repeat > 0:
--------time.sleep(delay)
--------print name + ": " + str(time.ctime(time.time()))
--------repeat -= 1
----print "Timer Complete"
So now we have our Timer that takes a Name, a Delay, and a Repeat number for how many times it will cycle the delay, now we just create threads to implement the Timer function we created, and they will be able to run at the same time, lets try:
def Main():
----t1 = Thread(target=timer, args=('Timer1', 10, 2))
----t2 = Thread(target=timer, args=('Timer2', 4, 5))
----t1.start()
----t2.start()
And Voila, we have our entire code to make a multi-threaded, multi-timer, application. What we did here is created a variable that stores our first Thread object, the target of which is the "timer" function, and the arguments we want it to use for the timer function are "Timer1" for the name of that timer, delay of 10s, and repeat that twice. A slightly different timer of 4s delays, repeated 5 times is set for our second Thread object, t2, named Timer2.
These variables, t1, and t2, they are storing the Thread object, but not running them. We can store them for later use to activate at any time we want, but for this tutorial's purpose, we will call them into existence right away. By calling "t1.start()", we tell Thread1 that we created earlier to Run using our specified parameters. Same with Thread2.
What will happen? Well, I would like you to try it for yourself and find out! Download python at https://python.org, once you have it installed you can run this through the command line or copy & paste to a .txt file, and rename it to a .py file. For spoilers, scroll past the next few images and output will be displayed for you.
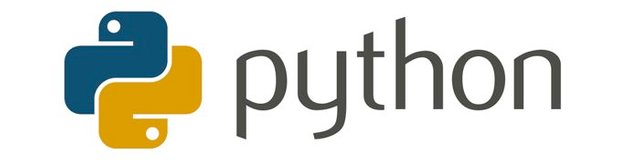
Follow Technium on Twitter!
Subscribe to Technium on YouTube for the latest video guides on FinTech and more!
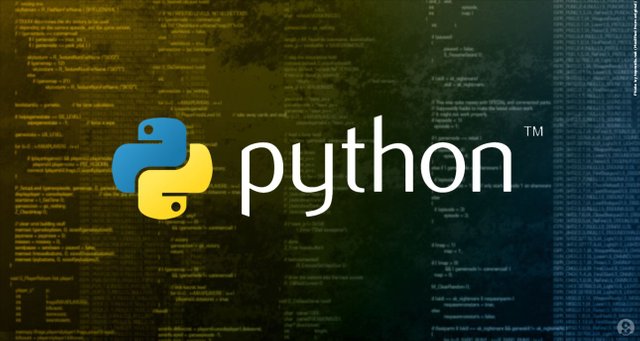
Output
Timer: Timer1 started.
Timer: Timer2 started.
Timer2: Sat Feb 04 23:11:37 2017
Timer2: Sat Feb 04 23:11:41 2017
Timer1: Sat Feb 04 23:11:43 2017
Timer2: Sat Feb 04 23:11:45 2017
Timer2: Sat Feb 04 23:11:49 2017
Timer1: Sat Feb 04 23:11:53 2017
Timer Complete
Timer2: Sat Feb 04 23:11:53 2017
Timer Complete
Thanks for the straight forward instructions, it is going to be sent over to a friend sooooooooon! All for one and one for all! Namaste :)
Thanks
To see full code on PasteBin, with syntax Highlighting & more, go here: http://pastebin.com/DDMjhgsj
Very good info @technium, thank you.
Thank You :D
Wow what a great day thank you all for the votes I appreciate it, you all helped push me to the front page of the How-To Trending Category!