Python and Network Automation HOW TO - Part 2 Configuration Commands
Moving from simple show commands to config commands with Python
In the first post on using Python to automate network show commands we got a base script going where we took precautions such as trying to make it agnostic to running in Python2 and Python3. We also got to the point where we ran a show single command against a single switch to receive some basic output. Before we get into running against multiple devices, I wanted to jump back into the steps of using Python and Netmiko to automate network tasks by doing some simple examples of configuration commands.
Once again, I'll be performing this against my lab HP2530 switch, but check out the first post for a list of supported devices in the Netmiko library.
Recap of what we have so far
Just to recap, this is what we have in our script so far:
#!/usr/bin/env python
from __future__ import print_function
import netmiko
from getpass import getpass
connection = netmiko.ConnectHandler(ip='172.31.1.251',
device_type='hp_procurve',
username='admin',
password=getpass('Enter a password for the switch:\n'))
print(connection)
result = connection.find_prompt()
result += connection.send_command('show version')
print(result)
connection.disconnect()
We're going to take what we have and modify it a bit to make it more modular and easier should we want to incorporate this into something in real life. First before getting into the config section let's turn this setup into a Python class. A class will let us follow an object oriented type approach where we can create an instance of the class we make and then call the methods we define to do our work. We'll change our file to look something like the output below:
#!/usr/bin/env python
from __future__ import print_function
import netmiko
from getpass import getpass
class SteemitAutomate():
def __init__(self):
self.connection = netmiko.ConnectHandler(ip='172.31.1.251',
device_type='hp_procurve',
username='admin',
password=getpass('Enter a password for the switch:\n'))
def printConnection(self):
print(self.connection)
def showCommand(self):
result = self.connection.find_prompt()
result += self.connection.send_command('show version')
print(result)
sa = SteemitAutomate()
sa.printConnection()
sa.showCommand()
sa.connection.disconnect()
Some of the sections we changed are described here
- class SteemitAutomate() <- defines our class
- def init <- the constructor of the class, basically what creates the class when called. This class also creates the connection to the switch
- the 'self' declaration <- this is to help define that variables you refer to belong to self, or the class itself
- def printConnection(self) <- a method to just print out connection info
- def showCommand(self) <- we moved the functionality of our show commands here, the connection object is inherited from the init class
- sa = SteemitAutomate() <- outside the class definition, we create an instance of the class that has all the methods of the class too
We then call the methods by calling the object by name, sa in our case then following with a period "." then the name of the function. Above is an example sa.printConnection() which will call the printConnection function of the SteemitAutomate class from the sa object. Also at the end notice how we access the disconnect property of the Netmiko connection object through sa. It's important to disconnect when complete.
So now we have a good framework down for our class, let's add a method to handle configuration commands. We'll keep the config commands pretty static and next time we will add the ability to input a command after calling the script as well as select show or config command.
Adding the configuration Class Method
Add the following to the script, each field I will elaborate on below:
def configCommand(self):
changes = ['vlan 777 name Lucky7s']
output = self.connection.send_config_set(changes)
print(output)
- def configCommand(self): <- defining the Method
- changes = ['vlan 777 name Lucky7s'] <- this is a List of the changes to make, I'm adding a vlan and naming it
- output = self.connection.send_config_set(changes) <- we're calling the send_config_set of the connection object from Netmiko we made here, we assign it to a variable because we're going to print the output of what commands were sent too
- print(output) <- this will show you the commands that Netmiko ran against the switch
So now that we have that, lets use the new method and verify it adds the VLAN by changing our show command from show version to show vlan and then call the showCommand method before and after the change. Our code in it's entirety is now below:
#!/usr/bin/env python
from __future__ import print_function
import netmiko
from getpass import getpass
class SteemitAutomate():
def __init__(self):
self.connection = netmiko.ConnectHandler(ip='172.31.1.251',
device_type='hp_procurve',
username='admin',
password=getpass('Enter a password for the switch:\n'))
def printConnection(self):
print(self.connection)
def showCommand(self):
result = self.connection.find_prompt()
result += self.connection.send_command('show vlan')
print(result)
def configCommand(self):
changes = ['vlan 777 name Lucky7s']
output = self.connection.send_config_set(changes)
print(output)
sa = SteemitAutomate()
sa.printConnection()
sa.showCommand()
sa.configCommand()
sa.showCommand()
sa.connection.disconnect()
Moment of Truth!
Time to run this thing and see what happens! The output of me running it is below:
python steemit.py
Enter a password for the switch:
<netmiko.hp.hp_procurve_ssh.HPProcurveSSH object at 0x000002A2CE8E9908>
CH-2530#
Status and Counters - VLAN Information
Maximum VLANs to support : 256
Primary VLAN : DEFAULT_VLAN
Management VLAN :
VLAN ID Name | Status Voice Jumbo
------- -------------------------------- + ---------- ----- -----
1 DEFAULT_VLAN | Port-based No No
config term
CH-2530(config)# vlan 777 name Lucky7s
CH-2530(config)# end
CH-2530#
CH-2530#
Status and Counters - VLAN Information
Maximum VLANs to support : 256
Primary VLAN : DEFAULT_VLAN
Management VLAN :
VLAN ID Name | Status Voice Jumbo
------- -------------------------------- + ---------- ----- -----
1 DEFAULT_VLAN | Port-based No No
777 Lucky7s | Port-based No No
Success!! Now this may not seem like much, but wait until we get to running multiple commands against multiple devices at the same time!
Wrapping Up
So let's finish this up for now with a quick recap of what we did and accomplished today:
- Changed our Python file over to a Python class to make it more reusable in the future
- Added a configuration class to take a single command and apply to our device
- Created an instance of our class and tested our methods
Next time we will expand upon this to do more and more. If you have anything you'd like to see let me know and I appreciate you reading this and the support.
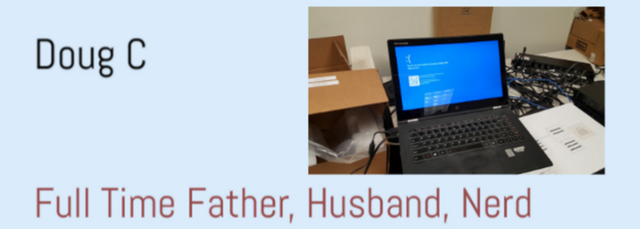