Lesson #5 Control structures. Part 2. Cycles.
Hi friends,
I am Jyoti from India. here I am going to participate in the SEC S20 W5 contest:https://steemit.com/devwithseven/@alejos7ven/basic-programming-course-lesson-5-control-structures-part-2-cycles-esp-eng organised by @alejos7ven
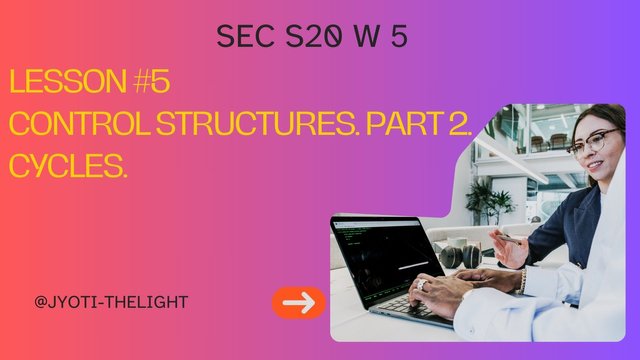.jpg)
Lesson #5 Control structures. Part 2. Cycles. |
---|
Explain the cycles in detail. What are they and how are they used? What is the difference between the While and Do-While cycle?
Loop or Cycle
A loop or cycle is a loop that continues to perform a given task until a certain condition is reached i.e. n number of times.
There are three variations in the Loop:
- For Loop
- While Loop
- Do while Loop
For Loop
A for loop is a control flow statement that causes a set of codes to be run repeatedly. It has three types of expressions: initialization, condition, and increment/decrement. The loop's processes are based on these three, and all three are defined at the same place.
Initialization - where to start,
The loop will run only if condition - is true.
Increment/Decrement - Used to indicate how much value should be continuously incremented or decremented each time.
for(initialization;condition;increment/decrement){
statement;
.....
.....
}
Example: A simple program to print a sentence a number of times using increment and decrement method in the for loop.
#include<stdio.h>
#include<conio.h>
int main(){
char sentence[30]="I love Steemit";
int i, n;
printf ("Enter how many times want to print: ");
scanf("%d",&n);
for(i=1;i<=n;i++){
printf("\n%s",sentence);
}
printf("\n");
for(i=n;i>=1;i--){
printf("\n%s",sentence);
}
return 0;
}
Output:
Enter how many times want to print: 3
I love Steemit
I love Steemit
I love Steemit

While Loop
A while loop is a control flow statement that causes a set of codes to run repeatedly. In which initialization, condition, increment/decrement these three are defined at different place.
- initialization - where to start,
- The loop will run only if condition - is true.
- increment/decrement - Used to indicate how much value should be continuously incremented or decremented each time.
initialization;
while(condition){
statement;
.....
.....
increment/decrement;
}
Example: A simple program for print mathematical table
#include<stdio.h>
#include<conio.h>
int main(){
int table,terms,sno;
printf("\n Enter which table you want: ");
scanf("%d",&table);
printf("\n How many terms want to print: ");
scanf("%d",&terms);
sno=1; //initialization
while(sno<=terms) // condition
{
printf("\n %d * %d = %d",sno,table,(sno*table));
sno++; //increment
}
return 0;
}
Output:
Enter which table you want: 6
How many terms want to print: 4
1 * 6 = 6
2 * 6 = 12
3 * 6 = 18
4 * 6 = 24

Do-While Loop
Do-while loop is similar to the while loop. But there is one important difference. The do-while loop statements will run before the condition is checked. Only after that, the condition will be checked. So in the do-while loop, the statements will run once whether the condition is true or false.
do{
// body of do while loop
statement 1;
statement 2;
}while(condition);
Where should I use the do-while loop?
If you enter a wrong number instead of entering a correct number in a program. You can enter the correct number only if you close the program and run it again. But if you use do-while loop you can enter the number again without closing the program. In such a context, a do-while loop is used.
Example: A simple program for keep asking enter a valid 4 digit number until get valid number using do-while loop
#include<stdio.h>
#include<conio.h>
int main(){
int number;
do
{
printf("\n Enter a 4 digit positive number: ");
scanf("%d", &number);
if(number<0 || number<1000 || number>9999){
printf("\n Given number is invalid");
}else{
printf("\n Success!..");
}
}while(number<0 || number<1000 || number>9999); // keep asking for numbers until get valid
return 0;
}
Output:
Enter a 4 digit positive number: 362
Given number is invalid
Enter a 4 digit positive number: 2527
Success!..

Investigate how the Switch-case structure is used and give us an example.
A switch statement is a long if statement, i.e. it is used instead of many if statements. In this, using the keyword case, it allows to check each condition and run the program statements accordingly.
Example: A program for finding weekdays using the switch statement
#include<stdio.h>
#include<conio.h>
int main(){
int number;
printf("Enter a number between (1 to 7): ");
scanf("%d",&number);
switch(number){
case 1:
printf("Sunday\n");
break;
case 2:
printf("Monday\n");
break;
case 3:
printf("Tuesday\n");
break;
case 4:
printf("Wednesday\n");
break;
case 5:
printf("Thursday\n");
break;
case 6:
printf("Friday\n");
break;
case 7:
printf("Saturday\n");
break;
default:
printf("This is invalid number to find week days");
break;
}
return 0;
}
Output 1:
Enter a number between (1 to 7): 4
Wednesday
Output 2:
Enter a number between (1 to 7): -5
This is invalid number to find week days

Explain what the following code does:
Algorithm switchcase
Define op, n, ns, a As Integer;
Define exit As Logic;
exit = False;
a=0;
Repeat
Print "Select an option:";
Print "1. Sum numbers.";
Print "2. Show results."
Print "3. End program.";
Read op;
Switch op Do
case 1:
Print "How much nums do you want to sum?";
Read n;
For i from 1 to n Do
Print "Enter a number: ";
Read ns;
a = a + ns;
EndFor
Print "Completed! Press any button to continue";
Wait Key;
Clear Screen;
case 2:
Print "This is the current result: " a;
Print "Press any button to continue.";
Wait Key;
Clear Screen;
caso 3:
Clear Screen;
Print "Bye =)";
exit = True;
Default:
Print "Invalid option.";
Print "Press any button to continue";
Wait Key;
Clear Screen;
EndSwitch
Until exit
EndAlgorithm
The above code is an implementation of a menu-driven program that allows the user to perform three different operations: summing numbers, showing the current result, and ending the program.
The program starts by defining several variables, including the operator , the number of values to sum, the input number, and a variable to store the sum. It also sets a flag variable to False and initializes the sum variable to 0.
The program then enters a loop that displays a menu of options to the user and reads the user's choice. Depending on the user's choice, the program performs one of three operations:
If the user chooses to sum numbers, the program prompts the user to enter the number of values to sum and then enters a loop that reads n values from the user and adds them to the sum variable. Once all values have been read and added, the program displays a message indicating that the operation is complete and waits for the user to press a key before clearing the screen and returning to the menu.
If the user chooses to show the current result, the program simply displays the current value of the sum variable (a) and waits for the user to press a key before clearing the screen and returning to the menu.
If the user chooses to end the program, the program clears the screen, displays a goodbye message, and sets the exit flag to True, which causes the loop to terminate.
If the user enters an invalid option, the program displays an error message and waits for the user to press a key before clearing the screen and returning to the menu.
The loop continues until the user chooses to end the program by selecting option 3.
Write a program in pseudo-code that asks the user for a number greater than 10 (Do-While), then add all the numbers with an accumulator (While) Example: The user enters 15. The program adds up to 1+2+3+4+5+6+7+8+9+10+11+12+13+14+15.
Here's an example program in C that asks the user for a number greater than 10, and then adds all the numbers up to that number using an accumulator:
#include <stdio.h>
int main() {
int num, sum = 0, i = 1;
do {
printf("Enter a number greater than 10: ");
scanf("%d", &num);
} while (num <= 10);
while (i <= num) {
sum += i;
i++;
}
printf("The sum of all numbers up to %d is %d\n", num, sum);
return 0;
}
Let us compile this code using an online C compiler
Now Let us check its output
I would like to invite
to take part in this contest.
Discord : @jyoti-thelight#6650 Telegram :- https://telegram.org/dl
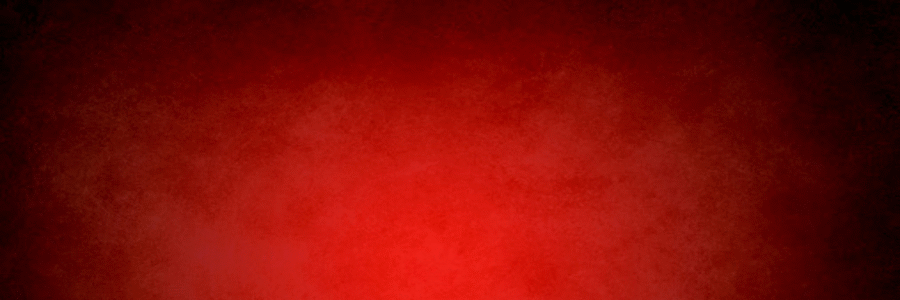
Congratulations, your post has been upvoted by @scilwa, which is a curating account for @R2cornell's Discord Community. We can also be found on our hive community & peakd as well as on my Discord Server
Felicitaciones, su publication ha sido votado por @scilwa. También puedo ser encontrado en nuestra comunidad de colmena y Peakd así como en mi servidor de discordia
0.00 SBD,
0.25 STEEM,
0.25 SP
Thank you very much
Upvoted. Thank You for sending some of your rewards to @null. It will make Steem stronger.
Thank you @malikusman1 for the great support
Oh! Wow you had explained all the things so good 😊. I believe that you had be a well trained person in coding. Thank for inviting me. I try to take participate in this steemit contest