Make Pyramid Structures Using C++
Hello my dear steemians! I hope you all are well and good.
Today I am here to tell you about how you can make decreasing and increasing triangle patterns of stars or simple numbers using c++.
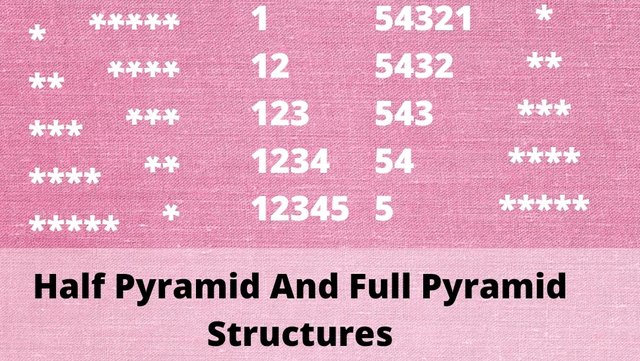
So let's start. First of all we have to use the for loop in this case in the program. We will use two for loops for these triangular structures and then three for the complete pyramid shape.
Now the question arises that why will we use two loops? So let me tell you that in this pattern there are two things. One is row and the other is coloumn. And similarly, one for loop will execute the rows and the other will execute the coloumns.
For Loop
I want to explain a little about the for loops working first because jumping to the program so that you guys can understand that how the programs will work. It is basically addition in the world of financial technology because these patterns are used to make different strategies in many ways and are useful to understand the possibilities.
- The first for loop will executes only once in an ietration.
- But the second for loop will execute completely to its limit.
How can we make increasing triangle patterns of stars using C++?
As we have to display the increasing triangle pattern. So in the first loop we will decide the number of rows and in the second loop we will give the number of coloumns.
And we will bound the second loop to the the first loop. And it will execute according to the number of rows.
For example: if the first loop prints only one row then the second will print only one coloumn. And so on if the first loop is printing out the third row now the value of the coloumns will also be three and now the three coloumns will be produced.
We can fix the number of rows in the first for loop, but I am going to give the opportunity to the user, they user will be able to put any number of rows and will be able to get the output accordingly.
#include < iostream >
using namespace std;
int main()
{
int rows;
cout << "Enter number of rows: ";
cin >> rows;
for(int i = 1; i <= 5; i++)
{
for(int j = 1; j <= i; j++)
{
cout <<j<<" " ;
}
cout<<endl;
}
return 0;
}
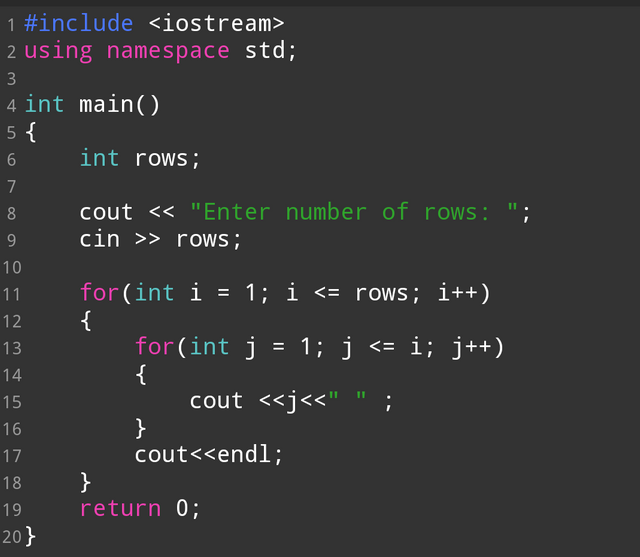
Output
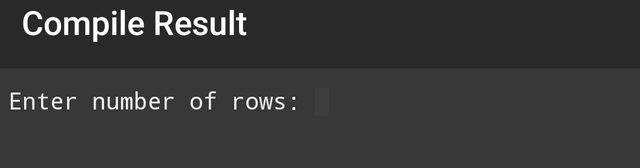
So here the user have to put the desired number of rows as I haven't fixed the number of rows.
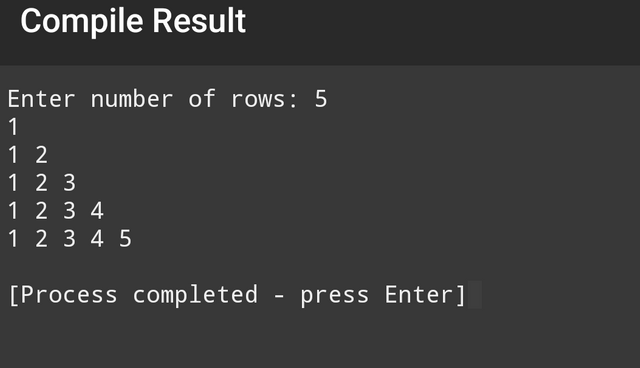
Here you can see that an increasing triangle has been produced. I have used just the numbers so that you can understand the pattern easily.
And now we have to print out the staric pattern of the increasing triangle. For this purpose we have to change the line number 15 cout <<j<<" " ;
just replace the j
with the "*"
and now the increasing triangle of stars will be produced.
#include < iostream >
using namespace std;
int main()
{
int rows;
cout << "Enter number of rows: ";
cin >> rows;
for(int i = 1; i <= 5; i++)
{
for(int j = 1; j <= i; j++)
{
cout <<"*"<<" " ;
}
cout<<endl;
}
return 0;
}
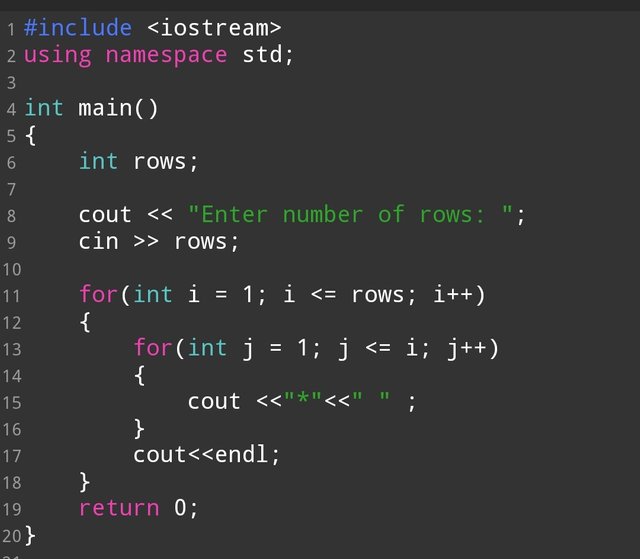
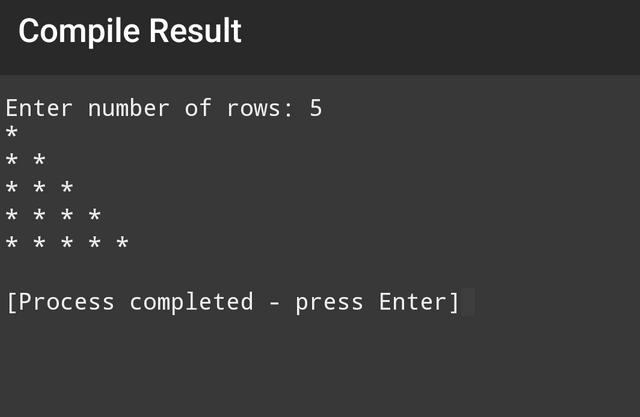
So finally the staric pattern of the increasing triangle has been produced.
How can we make decreasing triangle patterns of stars using C++?
As you guys have understood to make the increasing triangle pattern so now it is easy to make decreasing triangle pattern from that program.
So we just have to rearrange the elements of the second for loop. As we have to make the decreasing pattern so we have to initialise the second loop from the maximum value and then we have to decrease the value after each iteration.
So firstly I am going to make the decreasing pattern of the numbers.
#include < iostream >
using namespace std;
int main()
{
int rows;
cout << "Enter number of rows: ";
cin >> rows;
for(int i = 1; i <= rows; i++)
{
for(int j = rows; j >= i; j--)
{
cout <<j<<" " ;
}
cout<<endl;
}
return 0;
}
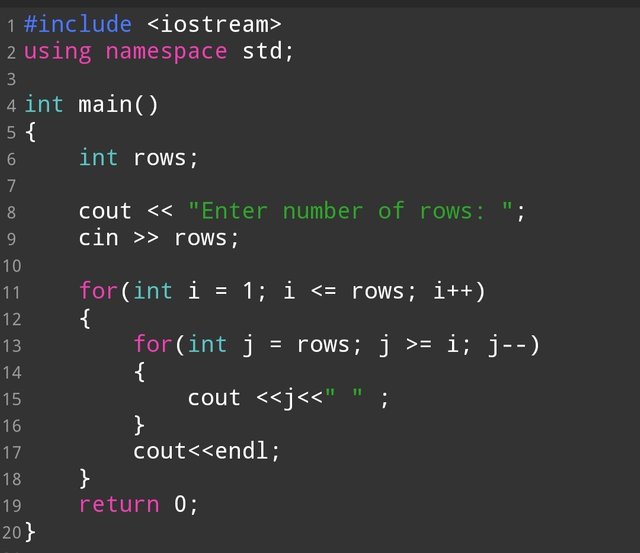
Output
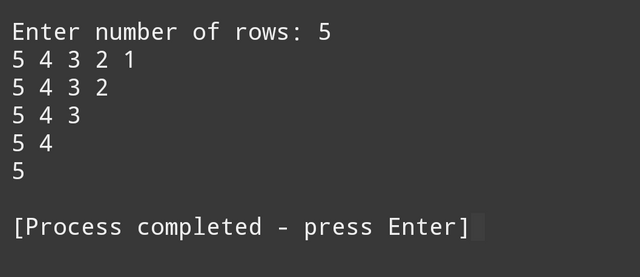
So here you can see that it is the decreasing pattern of the triangle. You can print out anything in this pattern using the above code. But you have to change the code in the input and you have to out your desired thing which you want to print out.
#include < iostream >
using namespace std;
int main()
{
int rows;
cout << "Enter number of rows: ";
cin >> rows;
for(int i = 1; i <= rows; i++)
{
for(int j = rows; j >= i; j--)
{
cout <<"*"<<" " ;
}
cout<<endl;
}
return 0;
}
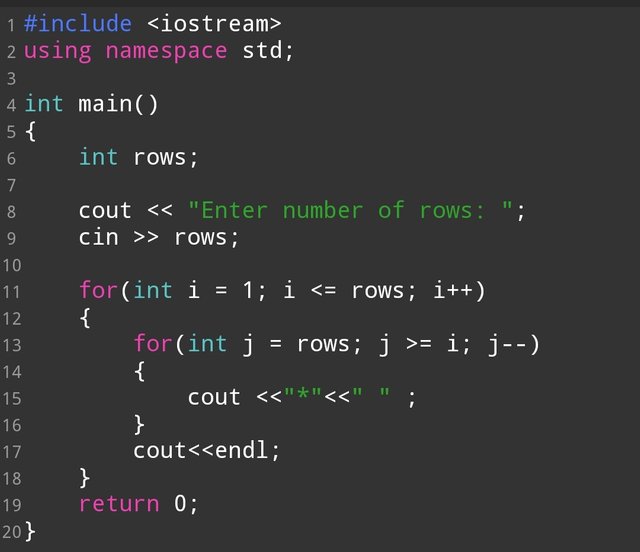
Output
So here you can see that just by changing the second for loop we have made the decreasing pattern of the triangle.
How to make half pyramid increasing pattern with decreasing spaces?
So now in order to make the increasing half pyramid structure structure with the decreasing spaces on the right side, we use three fir loops. We use one extra for loop because in this we have to print out the spaces on the right side and then the other loop will print the next visible pattern.
#include< iostream >
using namespace std;
int main()
{
int rows,i,j;
cout << "Enter number of rows: ";
cin >> rows;
for(i = 1; i <= rows; i++)
{
for(j = rows; j >= i; j--)
{
cout<<" ";
}
for(j=1;j<=i;j++)
{
cout<<j;
}
cout<<endl;
}
return 0;
}
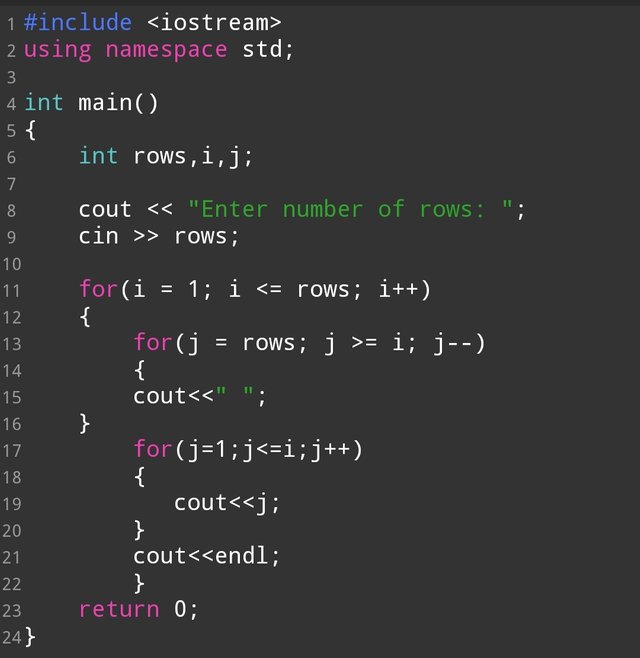
Output
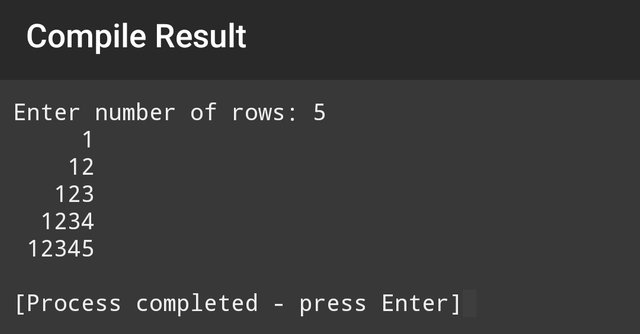
So finally the half pyramid increasing pattern of numbers with the decreasing spaces on the left side has been produced.
Now we can make the half pyramid increasing pattern with decreasing spaces on the left side just by changing the output line. As you can see in the code below that I have changed the output code in the line 19.
#include < iostream >
using namespace std;
int main()
{
int rows,i,j;
cout << "Enter number of rows: ";
cin >> rows;
for(i = 1; i <= rows; i++)
{
for(j = rows; j >= i; j--)
{
cout<<" ";
}
for(j=1;j<=i;j++)
{
cout<<"*";
}
cout<<endl;
}
return 0;
}
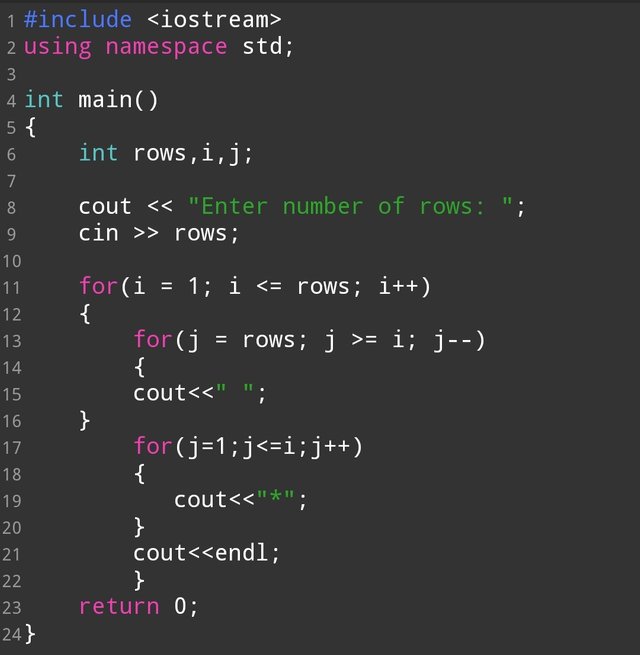
Output
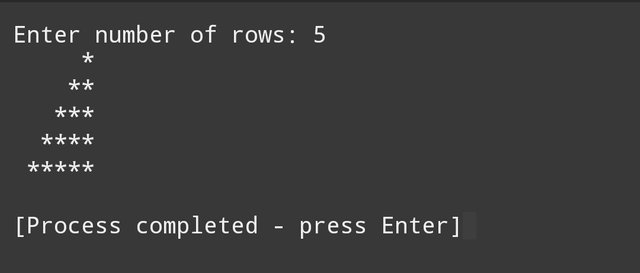
So you can see the output and our half pyramid stars increasing pattern with decreasing spaces on the left side has been produced.
Complete Pyramid Shape Structures
Complete pyramid structures are also easy to make so let me show you a sample of that and I hope you guys will understand it.
#include < iostream >
using namespace std;
int main()
{
int rows,i,j;
cout << "Enter number of rows: ";
cin >> rows;
for(i = 1; i <= rows; i++)
{
for(j = rows; j >= i; j--)
{
cout<<" ";
}
for(j=1;j<=i;j++)
{
cout<<"* ";
}
cout<<endl;
}
return 0;
}
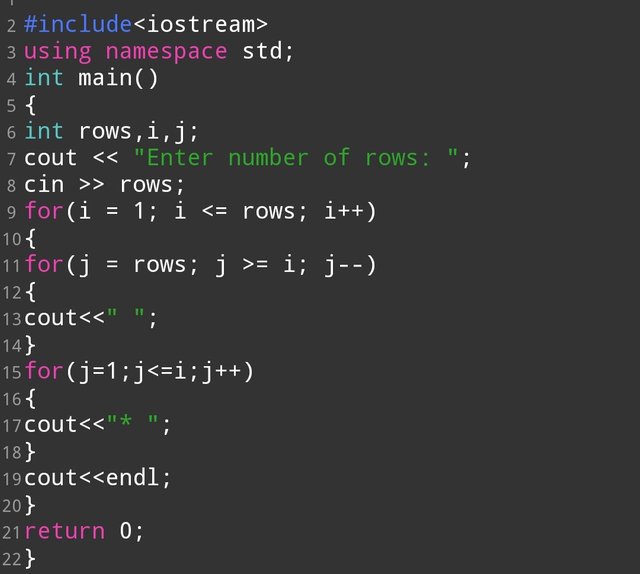
Output
So guys finally a complete pyramid structure is here which we have made using the c++ language. I have made it of 8 rows. And you can see it has complete symmetry. So dear steemians in this way you can also make these structures and if you have any confusion then you can ask I'll guide you.
Yours: @mohammadfaisal
Thank you for contributing to #LearnWithSteem theme. This post has been upvoted by @Reminiscence01 using @steemcurator09 account. We encourage you to keep publishing quality and original content in the Steemit ecosystem to earn support for your content.
Regards,
Team #Sevengers
Please your club status on the tags.
I have put....thank you 😊