SEC S19W5 || Website Interactivity with JavaScript
Dear Steemians,
Welcome to week 5 of our Steemit Engagement Challenge Season 19 competition, hosted by the Dynamic Devs team! This week, we’ll dive into the fascinating website interactivity world using JavaScript.
JavaScript is a powerful tool that makes web pages come alive and interactive. From creating dynamic forms to engaging animations to responsive user interfaces, JavaScript is at the heart of the modern web experience.
Your mission this week will be to demonstrate your JavaScript skills by creating interactive elements for a website. You’ll have the opportunity to explore various aspects of the language, such as DOM manipulation, event handling, and using built-in functions.
We look forward to seeing your creativity and expertise in action. Good luck to all participants!
Why JavaScript?
- To execute specific processes to bring interactivity to web pages.
- To control the objects of a web page.
PLACEMENT OF JAVASCRIPT CODE
FIRST METHOD:
Place the JavaScript code within a <script>
tag in an HTML page in the <head>
section.
Or in the <body>
section.
SECOND METHOD:
Place the JavaScript code in a separate file.
The second method is recommended and preferred whenever possible.
JAVASCRIPT IDENTIFIER
A JavaScript identifier must start with a letter, an underscore (_), or a dollar symbol ($).
Note: JavaScript is case-sensitive. Pay attention to uppercase and lowercase letters! The following characters can be digits (0 to 9).
DECLARATIONS
Variables can be declared in 4 ways:
- Explicitly: Tell JavaScript that this is a variable. The command to declare a variable is the
var
keyword. For example:
- Implicitly: Directly write the name of the variable followed by the value assigned to it, and JavaScript will accommodate it. For example:
- Using the
let
keyword:
A variable declared with let
can be reassigned.
- Using the
const
keyword:
A variable declared with const
cannot be reassigned.
DATA TYPES USED
- String
- Number
- Boolean
EVENTS USED
Use an event handler attached to HTML tags to call predefined functions.
Event Description
onclick
: When the user clicks on a button of type="button" or an image or a hyperlink.onsubmit
: When a form is submitted.onload
: When the page is completely loaded.
INPUT/OUTPUT
INPUTS
- Using a form object:
getElementById()
is a method of the document that returns an element object representing the element whose id property matches the specified string.
- Using an assignment.
OUTPUTS
- Variable assignment:
Dialog box to display a message.
innerHTML
: It is a property of any HTML element that designates the content found between the opening and closing tags.In the example below,
innerHTML
has the value "a text":
You can get the content of the paragraph with this code:
You can dynamically replace the content of the paragraph with this code:
- Write to the console:
- Directly display in the HTML document:
PREDEFINED FUNCTIONS USED
MATH OBJECT
Object | Role | Syntax |
---|---|---|
round() | Method that returns the nearest integer. | x = Math.round(y); |
trunc() | Method that returns the integer part of a real number. | x = Math.trunc(y); |
sqrt() | Method that returns the square root of a numeric value >= 0. | x = Math.sqrt(y); |
random() | Method that returns a random real number belonging to [0, 1[. | y = Math.random(); |
To get an integer value ∈ [min, max], you can use the following formula:
STRING OBJECT
Object | Role | Syntax |
---|---|---|
length | Property that indicates the length of the string. | x = string_obj.length; |
substr(p, n) | Returns a substring based on a position p and a number of characters n passed as an argument. The parameter n can be omitted to include all characters to the end of the string. | x = string_obj.substring(p, n); |
charAt(x) | Method that returns a character c of a string at position x . | c = string_obj.charAt(x); |
indexOf(ch1, p) | Returns the position of the first occurrence of ch1 in a string, starting the search at position p (otherwise -1). | x = string_obj.indexOf(ch1, p); |
lastIndexOf(ch1, p) | Returns the position of the last occurrence of ch1 in a string, starting from position p . | x = string_obj.lastIndexOf(ch1, p); |
replace(ch1, ch2) | Replaces the first occurrence of ch1 with ch2 in a string. | x = string_obj.replace(ch1, ch2); |
toUpperCase() | Converts all characters of a string to uppercase. | x = string_obj.toUpperCase(); |
toLowerCase() | Converts all characters of a string to lowercase. | x = string_obj.toLowerCase(); |
trim() | Removes all spaces existing at the beginning and the end of a string. | x = string_obj.trim(); |
TYPE CONVERSION FUNCTIONS
Object | Role | Syntax |
---|---|---|
Number() | Converts a string to a number. | x = Number(str); |
String() | Converts a numeric value to a string. | x = String(num); |
TYPE VERIFICATION FUNCTIONS
Object | Role | Syntax |
---|---|---|
isNaN() | Returns true if the argument passed is not a number; otherwise returns false. | x = isNaN(value); |
Homework :
We propose to create a website to help students solve some mathematical problems, specifically:
- Solving a quadratic equation
- Decomposing an integer into a product of prime factors
To do this, you are asked to:
- Create the "index.htm" page containing the following sections:
Here is the English translation of the provided document:
TP N°1 - HTML5 & CSS3 & JS23/24
We propose to create a website to help students solve some mathematical problems, specifically:
- Solving a quadratic equation
- Decomposing an integer into a product of prime factors
To do this, you are asked to:
- Create the "index.htm" page containing the following sections:
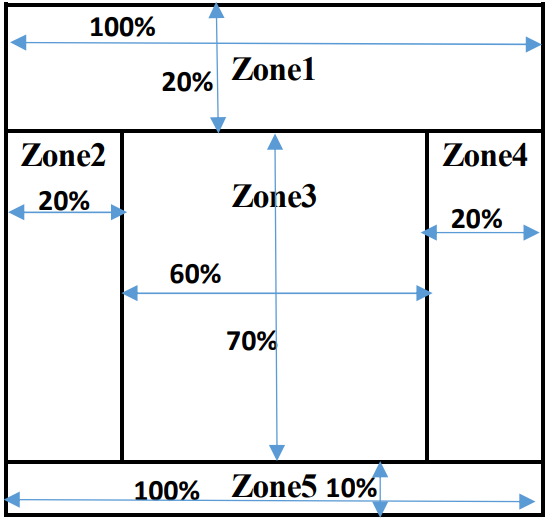
Knowing that:
Zone 1: An element representing the page header containing the text "Math Tutoring".
Zone 2: An element that represents the navigation panel containing the following hyperlinks:
- Identify: Links to the "identification.html" page.
- Equation Resolution: Links to the "resequation.html" page.
- Prime Factor: Links to the "FactPrem.html" page.
Zone 3: An element representing the display area of the different pages of the site, which will by default contain the "identification.html" page.
"Teaching: learning to know, to know how to do, to make known. Education: learning to be." - Louis Pauwels, Artist, Writer, Journalist (1920 - 1997)
Zone 4: An element representing the area for displaying additional information, containing a division with the following quote:
Zone 5: An element representing the footer of the page with the text "Contact us at [email protected]".
Task: Structure the "index.html" page using mainly semantic tags for layout. Link all created HTML pages to a stylesheet named "messtyles.css". Add CSS style rules to apply:
- Dimensions for each zone as indicated in the figure.
- Zone 1 background is a full-screen, no-repeat image "banniere.jpg".
- Transform the text in Zone 1 to uppercase, center it with a font named "impact" size 48px, color #87D7FF, bold style, and a shadow value of 2px 3px 5px rgba(127, 127, 127, 0.6).
- Background of Zone 2 and Zone 4 is color #DBFEC0.
- Background color of links is #69C130.
- Space the links and remove underlines.
- Transform link text to uppercase with white color and size 15px.
- On hover, the link background color changes to rgba(89, 225, 202, 0.8).
Zone 3: Background color #C0FCDE.
Zone 4 quote division background: Color #BC0104, centered in Zone 4 with a shadow value of 2px 5px 3px rgba(0, 0, 0, 0.8).
Quote text: Justified, white color, size 0.9rem, respecting the formatting indicated in the figure.
Zone 5 background: Color #87D7FF.
Zone 5 text: Centered with a font named "Arial", size 0.9rem, dark blue color, bold style, and a shadow value of 2px 3px 5px rgba(127, 127, 127, 0.8).
Identification Page:
Create the "identification.html" page containing the following form:
Task:
The "Captcha" zone is used to secure the website against bots. It will be automatically filled on page load by a 4-digit integer returned by a JavaScript function named "GenerateCaptcha()".
Clicking on the image

will call the "GenerateCaptcha()" function to regenerate a new Captcha value.
Clicking on the "Login" button will call a JavaScript function named "Verify()" to validate the form fields based on the following checks:
- The client's identifier is a required field, exactly 8 characters long, starting with two uppercase letters followed by six digits.
- The value entered in the "Confirmation" field must match the generated Captcha code.
Add CSS style rules to apply:
- Form field formatting using a table centered and width = 95% of its container.
- All input fields have width = 100%, height = 20px, font size = 14px, and a 2px green border.
- All form buttons have width = 100px, height = 30px, right-aligned, spaced, green background color, white text, bold, centered.
- All labels have color #515050, font size = 0.9em, bold.
- All forms are centered on the page, width = 40%, bordered with 4px #69676c, shadow value of 0 0 20px 0 rgba(0, 0, 0, 0.2), 0 5px 5px 0 rgba(0, 0, 0, 0.24).
- Captcha zone font size = 2rem.
- Refresh image height = 18px; width = 18px.
Equation Resolution Page:
Create the "resequation.html" page containing the following form:
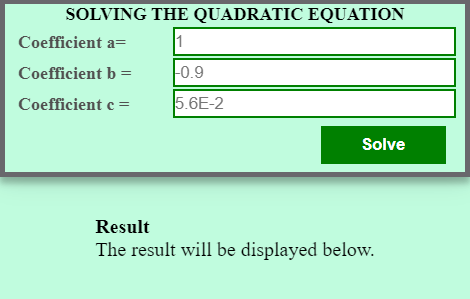
Task: Clicking the "Solve" button will call a JavaScript function named "calculate()" to:
- Validate that each coefficient (a, b, c) is numeric. If not, display "Please enter a numeric value".
- Solve the equation and display its roots.
Prime Factor Page:
Create the "FactPrem.html" page containing the following form:
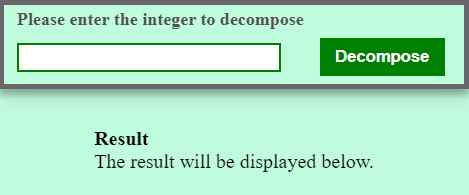
Task: Clicking the "Decompose" button will call a JavaScript function named "decompose()" to:
- Validate that the entered value is an integer between 2 and 99999999.
- Display the decomposition of the entered integer into prime factors.
Note: Decomposing an integer ( n ) into a product of prime factors involves writing the integer as a product of prime numbers. This can be done by:
- Dividing ( n ) by the first prime number ( p_1 ) (the smallest prime number is 2).
- If the remainder is zero, replace ( n ) with ( n / p_1 ) and add ( p_1 ) to the list of obtained factors.
- If the remainder is not zero, replace ( p_1 ) with the next prime number and repeat the previous step until ( n ) equals 1.
After integrating HTML5 semantic tags and CSS style rules, you should achieve the "index.html" page illustrated in the following figure:
What is required for both practice exercises:
- Open VSCode to create files.
- Take a screenshot of your completed pages in the browser.
- Share a video that demonstrates all the requested features in the site.
Image resources:
Contest Guidelines
Post can be written in any community or in your own blog.
Post must be #steemexclusive.
Use the following title: SEC S19W5 || Website Interactivity with JavaScript
Participants must be verified and active users on the platform.
Post must be more than 350 words. (350 to 500 words)
The images used must be the author's own or free of copyright. (Don't forget to include the source.)
Participants should not use any bot voting services, do not engage in vote buying.
The participation schedule is between Monday, August 5 , 2024 at 00:00 UTC to Sunday, - August 11, 2024 at 23:59 UTC.
Community moderators would leave quality ratings of your articles and likely upvotes.
The publication can be in any language.
Plagiarism and use of AI is prohibited.
Participants must appropriately follow #club5050 or #club75 or #club100.
Use the tags #dynamicdevs-s19w5 , #country (example- #tunisia, #Nigeria) #steemexclusive.
Use the #burnsteem25 tag only if you have set the 25% payee to @null.
Post the link to your entry in the comments section of this contest post. (very important).
Invite at least 3 friends to participate in this contest.
Strive to leave valuable feedback on other people's entries.
Share your post on Twitter and drop the link as a comment on your post.
Your article must get at least 10 upvotes and 5 valid comments to count as valid in the contest, so be sure to interact with other users' entries
Rewards
SC01 would be checking on the entire 7 participating communities and upvoting outstanding content. Upvote is not guaranteed for all articles. Kindly take note.
At the end of the week, we would nominate the top 3 users who had performed well in the contest and would be eligible for votes from SC01/SC02.
Important Notice: The nomination of the top 3 users in our community is not based on good grades alone, it includes their general engagements (quality and quantity of their interactions with other users' articles) to measure the overall performance. Also note that generally, you can only make one post per day in the Steemit Engagement Challenge.
Best Regards,
Dynamic Devs Team
Thank you, friend!


I'm @steem.history, who is steem witness.
Thank you for witnessvoting for me.
please click it!
(Go to https://steemit.com/~witnesses and type fbslo at the bottom of the page)
The weight is reduced because of the lack of Voting Power. If you vote for me as a witness, you can get my little vote.
This is an interesting and difficult task for us. But we enjoy to solve this task. Java script is the task where we handle our objects on the webpage layout.
Please add the new title. It relates to the previous task thank you.
Thank you, Yes, it's a bit different, especially with the integration of the JavaScript part. I'm confident that you're able to handle it, and I'm here for any explanations you might need.
Thanks for your cooperation. I try my Best to perform this task.
Wow. Amazing. Can't wait to put my entry.
Here is my participation:
https://steemit.com/hive-109435/@ahsansharif/sec-s19w5-or-or-website-interactivity-with-javascript