Basic programming course: Lesson #4 Control structures. Part 1. Conditionals.
Hello everyone, in today's post I will post the homework for @alejos7ven 's Basic programming course: Lesson #4 Control structures. Part 1. Conditionals. [ESP-ENG].
Let's get started!
Describe your understanding of conditional structures and mention 2 situations of everyday life where you could apply this concept.
Conditional structures are used for decision making, flow control, they allow you to execute code based on their results which could be True or False. In the following lines I will show you some common examples on how an IF Statement could look like.
Example 1:
if (condition) {
// Code to execute if condition is true
}
Example 2:
if (condition) {
// Code to execute if condition is true
} else {
// Code to execute if condition is false
}
Example 3:
if (condition1) {
// Code to execute if condition1 is true
} else if (condition2) {
// Code to execute if condition2 is true
} else {
// Code to execute if all conditions are false
}
And another conditional structure that could fit here is the switch statement, an if-else if-else written easier:
Example:
switch (expression) {
case value1:
// Code to execute if expression matches value1
break;
case value2:
// Code to execute if expression matches value2
break;
// More cases...
default:
// Code to execute if no case matches
}
Now let's see 2 situations from daily life where conditionals could be applied.
You are going to buy groceries, while going to the shop you prepare yourself a list of items that you need to buy, while picking items from the shelves you use conditionals to check if the item is present on your shopping list. If the item is on your shopping list you put it in the cart, if it isn't you leave it/put it back on the shelf.
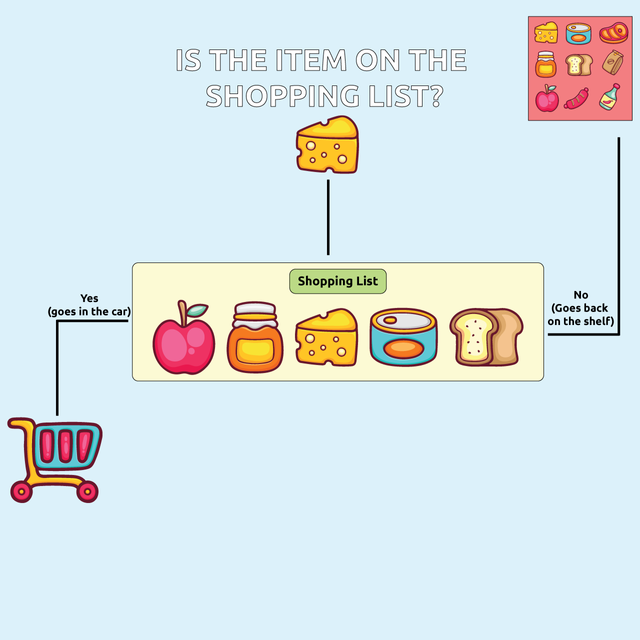
This is how it would look like in Python, the C version is taking so many lines because we need to define so many thing, in Python it's just a couple of lines.
# Define the shopping list
shopping_list = ["milk", "bread", "eggs", "butter", "cheese"]
# Get the item from the shelf
item_from_shelf = input("Enter the item picked from the shelf: ").strip()
# Check if the item is in the shopping list and print the appropriate message
if item_from_shelf in shopping_list:
print("Moving the item to the cart")
else:
print("Item goes back on the shelf")
Another example would be when you are going to travel/drive somewhere and you use google maps/waze etc as a GPS, you set your destination and usually get a few routes each one with a different arriving time or each one with an estimate time of traveling.
We compare each route with the others until we find the shortest one, in Python it would look like this.
# Define the times for each route in minutes
route1 = 45
route2 = 30
route3 = 50
# Compare using if-else to find the shortest route
if route1 <= route2 and route1 <= route3:
shortest_route = route1
elif route2 <= route1 and route2 <= route3:
shortest_route = route2
else:
shortest_route = route3
# Print the result
print(f"The shortest route takes {shortest_route} minutes.")
Create a program that tells the user "Welcome to the room What do you want to do?", if the user writes 1 then it shows a message that says "you have turned on the light", if the user writes 2 then it shows a message that says "you have left the room". Use conditionals.
For this exercise I am going to use the following C online compiler.
We first need to print the Welcoming message and the options to the user, to do this we use the printf
function like this:
printf("Welcome to the room. What do you want to do?\n");
printf("Enter 1 to turn on the light or 2 to leave the room: ");
Now to take the choice from the user we need a variable, we are going to make it an integer and declare it like this int choice;
and we use the scanf function to get the value from the user:
scanf("%d", &choice);
We are almost done, everything is prepared all we have to do now is use conditionals to check the value user entered in the choice variable.
Using IF statmenet we are checking if choice has the value 1 or 2 and print the corresponding message, if there's any other value we are going to let the user know he used an invalid choice. It would look like this.
if (choice == 1) {
printf("You have turned on the light.\n");
} else if (choice == 2) {
printf("You have left the room.\n");
} else {
printf("Invalid choice. Please enter 1 or 2.\n");
}
And the whole code in a block looks like this:
#include <stdio.h>
int main() {
int choice;
printf("Welcome to the room. What do you want to do?\n");
printf("Enter 1 to turn on the light or 2 to leave the room: ");
scanf("%d", &choice);
if (choice == 1) {
printf("You have turned on the light.\n");
} else if (choice == 2) {
printf("You have left the room.\n");
} else {
printf("Invalid choice. Please enter 1 or 2.\n");
}
return 0;
}
Let's check some outputs for the 3 printf's in our IF statement:
When we run the code, we are prompted with the following messages:
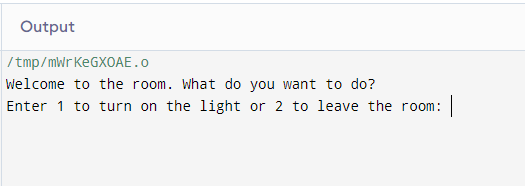
If we use the first choice
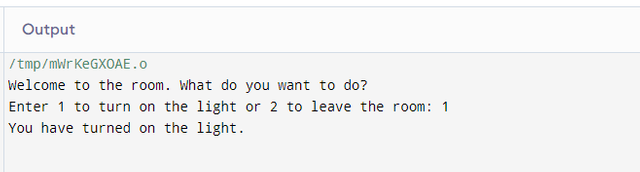
If we use the second choice
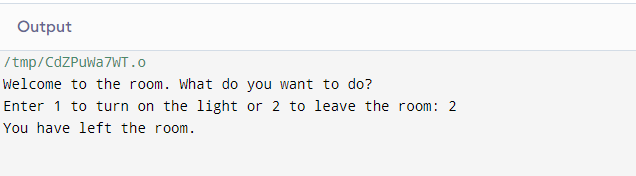
If we use an invalid choice
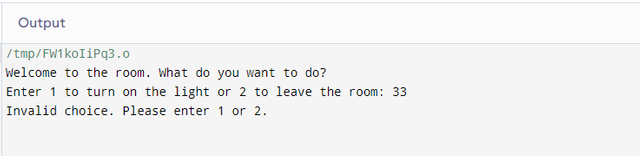
Create a program that asks the user for 4 different ratings, calculate the average and if it is greater than 70 it shows a message saying that the section passed, if not, it shows a message saying that the section can improve.
For this problem we need to declare our variables, the 4 ratings and the average value for these:
float rating1, rating2, rating3, rating4, average;
Once we defined the variables it's time to get the input from the user, each rating will hold it's own value, to get the value we are going to use scanf again like this:
printf("Enter rating 1: ");
scanf("%f", &rating1);
We are going to apply the above on all 4 ratings getting these scanfs:
printf("Enter rating 1: ");
scanf("%f", &rating1);
printf("Enter rating 2: ");
scanf("%f", &rating2);
printf("Enter rating 3: ");
scanf("%f", &rating3);
printf("Enter rating 4: ");
scanf("%f", &rating4);
Let's use the average variable now and give it a value, the average is the sum of the ratings above the number of ratings, so its (rating1+rating2+rating3+rating4)/4 and it would look like this:
average = (rating1 + rating2 + rating3 + rating4) / 4;
Now let's print the average value so we can see it, we are going to print only 2 decimals for aestetics:
printf("The average rating is: %.2f\n", average);
Now let's compare our average using an IF statement and see if it's above 70 and passed or can improve:
if (average > 70) {
printf("The section passed.\n");
} else {
printf("The section can improve.\n");
}
The whole code block would look like this:
#include <stdio.h>
int main() {
float rating1, rating2, rating3, rating4, average;
// Asking the user to input 4 ratings
printf("Enter rating 1: ");
scanf("%f", &rating1);
printf("Enter rating 2: ");
scanf("%f", &rating2);
printf("Enter rating 3: ");
scanf("%f", &rating3);
printf("Enter rating 4: ");
scanf("%f", &rating4);
// Calculating the average of the 4 ratings
average = (rating1 + rating2 + rating3 + rating4) / 4;
// Display the average
printf("The average rating is: %.2f\n", average);
// Conditional to check if the average is greater than 70
if (average > 70) {
printf("The section passed.\n");
} else {
printf("The section can improve.\n");
}
return 0;
}
Now let's see some example when we run the code:
Here we are going to give 4 ratings that in the end will have an average above 70 resulting in section passed:
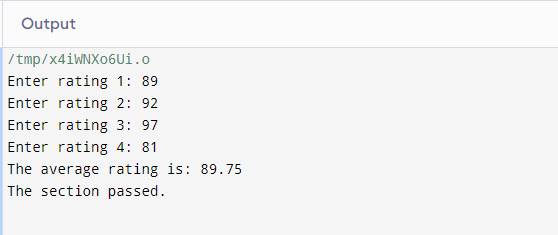
And now an example where we fall below 70 and we get the section can improve message:
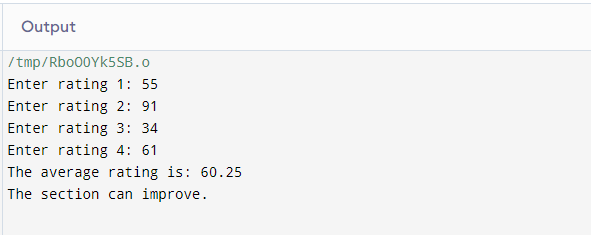
That was it for today's homework, thank you @alejos7ven for another great class.
In the end I would like to invite some friends to join @r0ssi, @titans, @mojociocio!
Until next time, I am wishing you a great day!
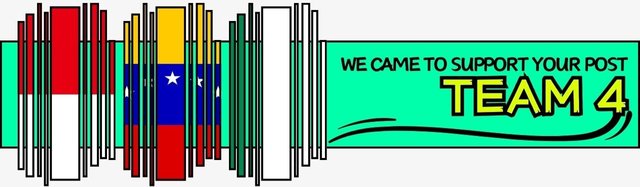