Project Inception Progress Report: Finished Key Generator
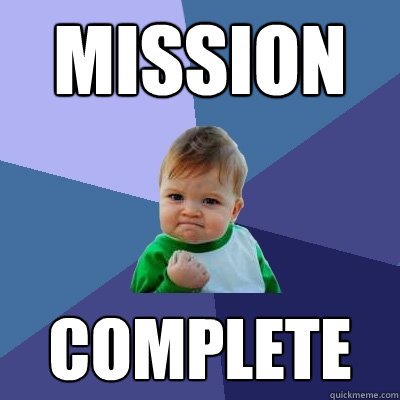
I
have completed the static html page for generating a set of public keys from a valid formatted password and WIF private key.
http://projectinception.lt/static/keygen.html
It automatically removes extraneous whitespace from the password field whenever the 'onchange' (press enter or click on another part of the page) event happens, checks if the input is a valid Steem username, and if not, puts an error message in the yellow box.
If the username is valid and the password is valid, then it generates the public keys and generates a block of text you can copy into a form I will make to buy an account.
The password field is read-only and automatically generates a random one on page load, or when the generate button is pressed, and generates it from a randomly generated hexadecimal string, which it feeds into the toWif()
function, puts a P in front (same as Steemit.com).
There is a random username generator you can also use if you intend to make the account 'nameless' and obviously never intended to be used with the forum, but only the money system. I will be generating such keys for the tumbler I will build after I finish building the Sybil Workshop Steem account brokerage.
Here is the code from the page, of course this is free for anyone to reuse or modify:
<html>
<head>
<title>Sybil Workshop Key Generator Static Page</title>
<style>
input {
font-family:Consolas,Monaco,Lucida Console,Liberation Mono,DejaVu Sans Mono,Bitstream Vera Sans Mono,Courier New, monospace;
}
</style>
<script src="steem.min.js"></script>
<script>
function randomHexString(length) {
mask = '0123456789abcdef'; // the set of characters used in hexadecimal
var result = '';
// generate 'length' number of characters
for (var i = length; i > 0; --i) result += mask[Math.floor(Math.random() * mask.length)];
return result;
}
function randomusername() {
maskFirst = 'abcdefghijklmnopqrstuvwxyz'; // first is only lowercase letter
maskLast = maskFirst + '01234567890'; // last is lowercase letter or number
maskMiddle = maskLast + '-'; // all between same as end plus hyphen
var result = '';
// generate first character from maskFrst set
result = maskFirst[Math.floor(Math.random() * maskFirst.length)];
// generate 14 characters in between from maskMiddle set, append
for (var i = 14; i > 0; --i) result += maskMiddle[Math.floor(Math.random() * maskMiddle.length)];
// generate last character from maskEnd set, append
result += maskLast[Math.floor(Math.random() * maskLast.length)];
// place generated username into username input field
document.getElementById("username").value = result;
// refresh the output of the form
keygen();
}
function checkusername() {
var password = document.getElementById("username").value;
// strip all whitespace at beginning, end and in the middle
var stripped = password.replace(/\s+/g,'');
if (stripped != password) {
// if whitespace was stripped, replace password automatically
password = document.getElementById("username").value = stripped;
}
// check that username conforms with constraints
var re = /^([a-z]{1})([a-z0-9\-]{1,14})([a-z0-9]{1})$/;
if (!re.test(password)) { // username is not valid
document.getElementById("output").innerHTML =
"<h3>Error: Invalid Username</h3>\
First character must be a lower case letter<br />\
Last character must be a lower case letter or number<br />\
There must be one or more character in between which can be<br />\
a string of lower case letters, numbers and hyphen (-)";
} else keygen(); // update generated output if any
}
function keygen() {
var username = document.getElementById("username").value;
var password = document.getElementById("password").value;
// if either field is entry, show error in output
if (username == "" || password == "") {
document.getElementById("output").innerHTML =
"All fields must be filled in";
}
else {
// generate parameters for account
var outputstring = "<pre>username: " + username; //+ "<br />password: " + password;
keys = steem.auth.generateKeys(username, password,
["posting", "active", "owner", "memo"]);
outputstring += "<br />posting: " + keys['posting'];
outputstring += "<br />active: " + keys['active'];
outputstring += "<br />owner: " + keys['owner'];
outputstring += "<br />memo: " + keys['memo'];
outputstring += "</pre>";
// display constructed output in output container
document.getElementById("output").innerHTML = outputstring;
}
}
function newkey() {
// generates a 32 byte random hex value then turns it into WIF format
document.getElementById("password").value = "P" + steem.auth.toWif("", "" ,randomHexString( 64 ));
keygen();
}
</script>
</head>
<body style="max-width:800px; margin: auto;" onload="newkey()">
<h1>Sybil Workshop Key Generator</h1>
<p>This script includes <a href="steem.min.js"><code>steem.min.js</code></a>
which was taken from
<a href="https://github.com/steemit/steem-js/blob/dev/dist/steem.min.js">
https://github.com/steemit/steem-js/blob/dev/dist/steem.min.js</a>
for the necessary cryptographic functions for you to generate the role keys
for ordering a new account here.</p>
<p>The parts of it that are necessary for this page will be refactored into
a single script within this page in the future.</p>
<h2>How to use:</h2>
<p>Save this web page to your local hard drive, and open the file from the
location you saved it in. This will gather the necessary script mentioned
above into a folder and this script will run offline on more or less any
web browser.</p>
<p><b>It is advised that you make doubly sure by turning off your
network connection before generating a key.</b></p>
<p>When the script is
integrated into this, the code will be quite easy to read and follow and
someone with reasonable skills in logic should be able to see that the
script does not attempt to write anything to disk or connect the network.
If you are uncertain, or just want to be doubly sure, just disable your
network connection while you are using this script.</p>
<h2>Make me new keys!</h2>
<p>A randomly generated master password will be created each time this page
loads.</p>
<p>Username: <input type="text" id="username" maxlength="16" size="16"
onchange="checkusername()" />
<button onclick="randomusername()">Generate Random Username</button><br />
<sub>(16 characters, first from [a-zA-z], last from [a-zA-Z0-9],<br />
minimum one character between first and last from [a-z0-9\-]<br />
(lower case letters, numbers and hyphens),<br />
and minimum 3, maximum 16 characters total length, all lower case.</sub></p>
<p>Password: <input type="text" id="password" size="51" maxlength="52" readonly />
<button onclick="newkey()">Generate New Password</button></p>
<p>Copy the password somewhere safe, it cannot be recovered!</p>
<p><button onclick="keygen()">Generate Role Keys</button></p>
<h3>Copy and paste the below list for the account purchase form:</h3>
<p id="output" style="background-color:yellow; padding:1em;">The generated set of keys will appear here.</p>
<h2>What this script does:</h2>
<p>In simple language, it takes your desired password, generates the 4 public
keys by which the network can authenticate that you are the controller of
the secret keys. The master key you input can also be used for login and
other types of transactions, but Steem network clients like steemit.com,
they only generate the secret keys according to the role (post/vote, transfer,
memo, etc) from it to create a signature on your transaction.</p>
<p>This script will generate the secret keys for your account
roles, and then their matching public keys. The secret keys you can keep
as well as the master password you generate/create for your own use, <i>but
for the purpose of buying a new account, you must only paste in the set of
<b>public role keys</b> generated.</i></p>
<p>This way you know you didn't give away the master password to me in the
process. I don't want to know it and the less people who know it, the
more secure your account is.</p>
</body>
</html>
Postscript
I think I have completely finished this, except for my future project to pull all of the stuff out of the script include that is not depended on by the scripts on the page. This will be enough for now, it does not take advanced skills to audit the executing code. I have corrected the script and more comprehensively commented it, it is intended to be easily grasped and modified by someone else, even with low level skills.
We can't code here! This is Whale country!
Vote #1 l0k1
Go to steemit.com/~witnesses to cast your vote by typing l0k1
into the text entry at the bottom of the leaderboard.
nice, message the ciccadia people to get on here and host their contest :D
here are the songs we can "expect" :D , coded crypto jumbos and nice guitars , not the best , but great compositions
thanks