Let's Build a Game! #3 - Progression
Ready to expand upon the game we built last time?
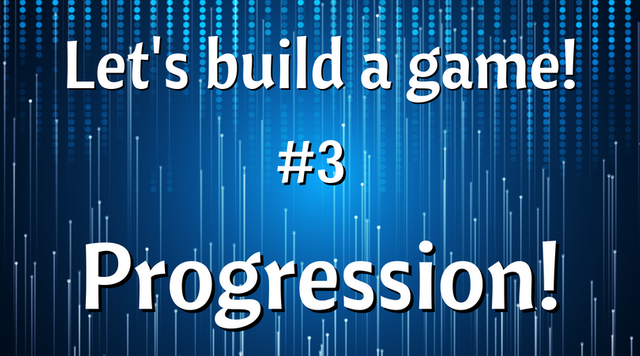
If you have followed the tutorial in part #2 of this series, you'll have a simple game in which you can walk around a grassy area, kill bugs, and collect any gold coins they drop. In that tutorial, we covered:
- Commands - A "mob" can use Verbs, and Procs instruct the game to make something happen.
- Creating Icons - Icons are graphics. They are used to display everything. Some can have multiple "states"
- Creating Maps - You gotta have somewhere for the player to be, right?
- Defining new mobs - It's boring if everyone is the same!
- Basic Troubleshooting - Sometimes, your code reads clearly, but doesn't exactly do what you wanted it to.
This next part of the tutorial will explain how to add progression to your game, so that players feel like they're actually growing or advancing in some way.

What's the most common element in RPGs across genres?
Leveling up!
Gaining experience levels has been a staple in RPGs from the beginning. It gives the player a sense of accomplishment each time they reach a new milestone. If we're going to build an RPG, we want our player to be able to earn some experience, and level up, for killing the bugs of the world.
That being the case, we'll need to define a few variables to keep track of the various parts of that process. One to track the mob's level, one for the amount of experience they currently have, another for how much experience is needed to reach the next level, and one to determine how much experience a mob will give when it's killed. That looks a little like this:
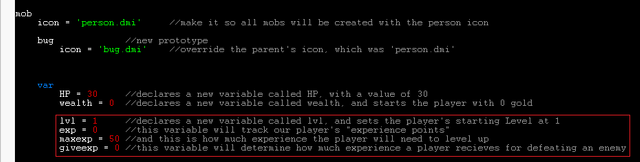
That last one says that all mobs in the world by default won't give any experience. We don't want that to be the case with our bugs, so you could either alter the default amount of experience given for all mobs, or simply override the "giveexp" variable for the bugs alone:

Now that we've defined how much experience our enemy should give, we need to implement a way to actually give that experience to the player. We'll do this inside our attack() verb, but along the way, I'm going to edit some of the code from the previous tutorial to fit a bit better with what we want to accomplish here.
We're going to edit the DeathCheck() procedure to require a variable when it gets called. We'll name this variable "attacker" for the sake of simplicity, and tell the procedure that the attacker must be a mob.

Now, whenever we call the DeathCheck() procedure, we'll need to tell it who the attacker was. We do this simply by adding a note inside the "( )" when we make the call:
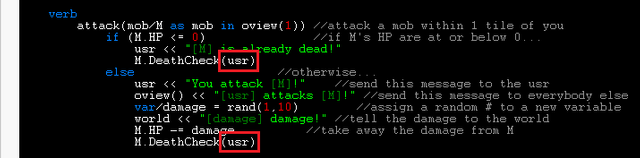
In this instance, we're telling the game that the "user" of the attack variable should be passed along to the DeathCheck(). All that's left is to use the information that gets passed along.

After we've established the mob is dead, we want to increase the attacker's exp variable by the dead mob's giveexp variable, then tell the attacker they received it. By having the attacker referenced, we can also take this opportunity to include information about who killed the mob in the message that gets broadcast.
If you compile the code and run the game, you will see that each time you kill an enemy, a message states that you received experience. This is great and all, but as the code stands, you will just continue to gain experience indefinitely, and never reach your next level...
We need a new procedure!
Just like how we check whether a mob has any health left, we need to check whether the player has enough experience to reach the next level. The procedure shown below is one of the simplest methods of doing this:
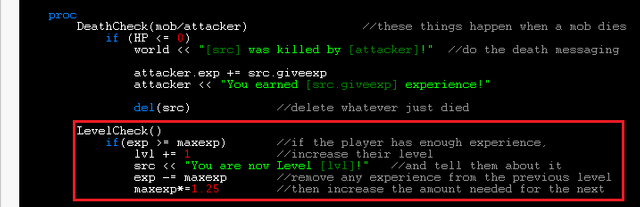
The comments explain how everything works, but basically, all we're doing is checking to see if the mob's exp is greater than or equal to the amount determined by their maxexp. If it is, we add 1 to the mob's level variable, and let them know they've leveled up.
The next two lines can be handled in a number of ways, but here, the procedure subtracts the amount of experience the player needed for the previous level so that it doesn't get counted as progress toward the next, then increases the amount of experience needed to reach the next level.
In action, if the player gains an amount of experience over what they need to level up, the remainder will be saved and pushed over. (If the player needs 50 experience to level up, already has 45, and gains 10, the extra 5 experience will be saved, and the player will then need 62.5 experience, minus the extra 5, to reach level 3.) Again, this can be handled a number of ways, and depends completely on how you want the run-over experience to be handled.
Any time we award the player experience, we'll need this procedure to run, so I'm going to add a call to the DeathCheck() procedure, right after the experience is awarded and announced to the player. I only put it after the announcement because in case we do have enough to level up, I want the experience message to come right before the level up message.

With all of this in place, we now gain experience from killing bugs, and level up for it!
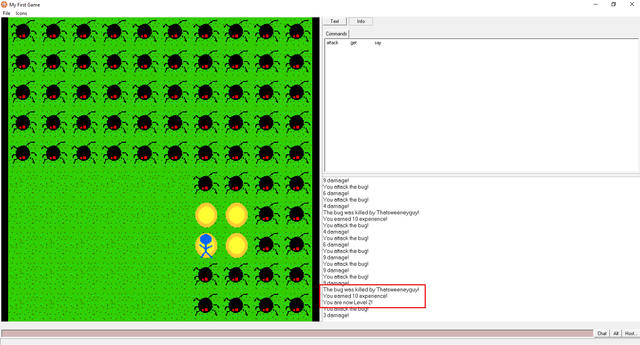
Games like this will usually increase the player's stats in some way when they level up, such as increasing the amount of HP the player has, or the amount of damage they deal with attacking. These can all be done simply by defining any wanted variable changes at the same time we increase the level stat. How you want the player's character to progress is completely up to you, the game's developer.

Challenge Time!
Let's see if you can take what we've learned so far, and create a "combat skill", allowing the player to become stronger the more they fight. Here's a rough idea of what you'll need to do. Some of the steps are left out on purpose, because I believe in your ability to fill in the blanks!
- Define your variables
You'll need a way to store information about the level of the skill, and a way to track experience for it.
- The more a player fights, the better they should get
Each time the player attacks a mob, award some experience toward the new skill, and be sure to check whether the player has enough.
- When they're better at fighting, they can kill things faster
Edit the code so that the new Combat skill affects the amount of damage a player deals when attacking.
I'll show you the whole procedure in the next part of this series so you can see how well you did.

Now we're getting somewhere!
In the next part of this series, we'll see our bugs come to life, giving our game difficulty!

Thanks for reading! Let me know what you think in the comments!
