[Update] Voter.js v0.02: Discord Auto Upvote Bot - How to Add Registering Feature
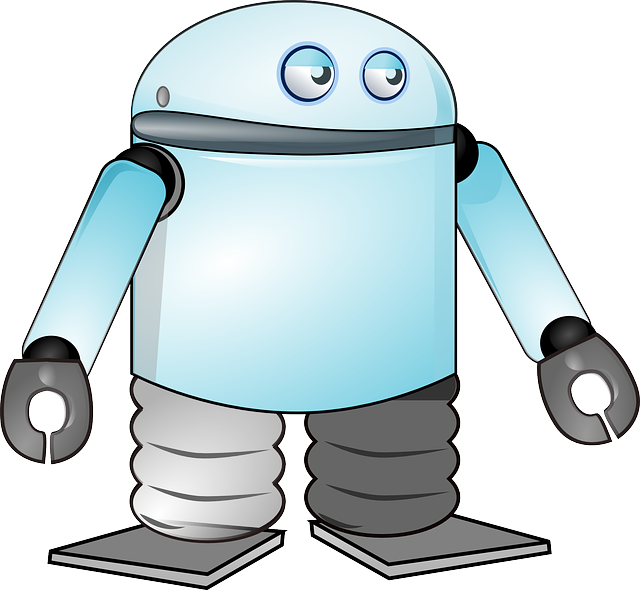
Thanks to @inertia for creating a great tool: cosgrove
ask your questions from @inertia about this tool.
This Article is about javascript. I try to show how javascript can used to communicate with steem blockchain and how you can create great tools by javascript.
Recently I published an Article, here: Complete Guide to Creating Discord Auto Upvote Bot by Javascript.
It is a complete guide for creating an auto voter bot like banjo or minnowsupport or like 'Steemfollower-voter' bot in this discord server
For running a discord bot, you need a VPS or a always online system.
minimum requirment system is 200~500 mb RAM.How it works?
this bot can work with a spicific commands like $upvote or without any command in spicific channels.
we will use Eris Discord library to connecting our bot. you can find this library here on github.
After upvoting a post, we will store that Discord User in Mysql Database with upvote time. we will need this time for checking next upvotes time.
Our bot will detect commands and post links, then it will check last upvote time for that user. if it was greater that 12 or 24 hours (you can change this) then post will get an upvote from spicific account. or User will receive an error message.
Some Fix:
After installing Mysql Database, You need to create tables by this commands:
CREATE TABLE voter (
id INT NOT NULL AUTO_INCREMENT , PRIMARY KEY (id),
user text NOT NULL,
lastvote text NOT NULL,
userid text NOT NULL);
If you already created 'voter' table by using last article, Instead, You can use this commands:
ALTER TABLE `voter` DROP PRIMARY KEY, ADD PRIMARY KEY( `id`);
ALTER TABLE `voter` CHANGE `id` `id` INT(11) NOT NULL AUTO_INCREMENT;
If you get an error in installing 'pm2' or 'steem' by npm, Try this instruction:
Enter npm -v
and check your npm version. if it was under '5', install nvm by these commands:
(it will install an stable version of npm)
sudo apt install curl
curl -o- https://raw.githubusercontent.com/creationix/nvm/v0.33.4/install.sh | bash
then:
export NVM_DIR="$HOME/.nvm"
[ -s "$NVM_DIR/nvm.sh" ] && . "$NVM_DIR/nvm.sh"
and:
source ~/.bashrc
Now try to install missed Required Libraries:
npm install steem --save
npm install --no-optional eris
npm install mysql
npm install pm2 -g
If you have not any problem with current version of voter.js file, you can add Register option too.
a few days ago, someone asked this feature:
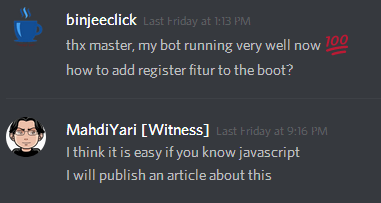
Let's Start Coding.
Adding Registering Option:
First we must install 'xmlhttprequest' by npm:
npm install xmlhttprequest
then open voter.js file and add this line to your voter.js file:
var XMLHttpRequest = require("xmlhttprequest").XMLHttpRequest;
You must add these codes after last '}':
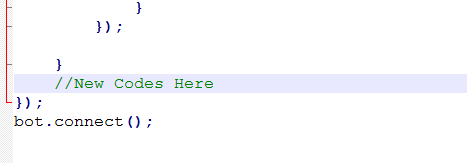
and a Regex for detecting '$register' Command:
var regex2 = /(\$)+(register)+(\ )/;
an 'if' for checking message:
if(msg.content.match(regex2)){
}
First we must check if user already registered or not,
then we will check voter account transactions for Spicific Memo and Username:
var sender = msg.content.replace(msg.content.match(regex2)[0],"");
var memo = 'register';
var transaction = 'Receive 0.001 SBD from '+sender;
var r = 0;
var channel = msg.channel.id;
con.query('SELECT EXISTS(SELECT * FROM `voter` WHERE `userid` = "'+msg.author.id+'" OR `user`="'+sender+'")', function (error, results, fields) {
for(i in results){
for(j in results[i]){
x = results[i][j];
if(x == 1){
setTimeout(function(){bot.createMessage(channel,'Already Registered!');},1000);
}else if(x == 0){
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
var response = JSON.parse(this.responseText);
for(i in response){
if(response[i].transaction == transaction && response[i].memo == memo){
con.query('INSERT INTO `voter`(`user`, `lastvote`, `userid`) VALUES ("'+sender+'","0","'+msg.author.id+'")', function (error, results, fields) {
r = 1;
setTimeout(function(){bot.createMessage(channel,'User '+sender+' Registered by <@'+msg.author.id+'>');},1000);
});
break;
}
}
setTimeout(function(){if(r == 0){
setTimeout(function(){bot.createMessage(channel,'Please Send 0.001 SBD to '+votey+' with memo:`'+memo+'` and Try again.');},1000);
}},2000);
}
};
xmlhttp.open("GET", "https://steemfollower.com/voter-tx.php?user=" + votey , true);
xmlhttp.send();
}
}
}
});
Now we must Change Some codes in last version:
find this part in your codes:
(line ~60)
}else{
var time = Math.floor(new Date().getTime() / 1000);
con.query('INSERT INTO `voter`(`user`, `lastvote`, `userid`) VALUES ("'+author+'","'+time+'","'+uid+'")', function (error, results, fields) {
steem.broadcast.vote(wifkey,votey,author,permlink,weight,function(downerr, result){
if(downerr){
setTimeout(function(){bot.createMessage(channel,'Already Upvoted!');},1000);
con.query('UPDATE `voter` SET `lastvote`="10" WHERE `userid`="'+uid+'"', function (error, results, fields) {
});
}
if(result) {
setTimeout(function(){bot.createMessage(channel,'Done! Your Post Upvoted By @mahdiyari');},1000);
}
});
});
}
and change it to:
}else{
setTimeout(function(){bot.createMessage(channel,'You Are not Registered.');},1000);
}
Now, you can run it by:
pm2 start voter.js
or restart it by:
pm2 restart voter.js
Full Code:
const Eris = require("eris");
var steem = require("steem");
var mysql = require('mysql');
var XMLHttpRequest = require("xmlhttprequest").XMLHttpRequest;
var con = mysql.createConnection({
host: "127.0.0.1",
user: "root",
password: "mysql password",
database: "voter"
});
con.connect();
var bot = new Eris("Your Bot Token");
var regex = /(\$)+(upvote)+.+(https:\/\/)+.+(@)+.+(\/)/;
var regex1 = /(@)+.+(\/)/;
var wifkey = 'Private Posting Key';
var votey = "mahdiyari";
var weight = 5000; // 10000 = 100%
bot.on("ready", () => {console.log('voter bot started!');}); //when it is ready
bot.on("messageCreate", (msg) => { // when a message is created
if(msg.content.match(regex)){
var permlink= msg.content.replace(msg.content.match(regex)[0],"");
var au = msg.content.match(regex1)[0];
var aut = au.replace("@","");
var author = aut.replace("/","");
var channel = msg.channel.id;
var uid = msg.author.id;
var x = '0';
con.query('SELECT EXISTS(SELECT * FROM `voter` WHERE `userid` = "'+uid+'")', function (error, results, fields) {
for(i in results){
for(j in results[i]){
x = results[i][j];
if(x == '1'){
var last;
con.query('SELECT `lastvote` FROM `voter` WHERE `userid`="'+uid+'"', function (error, results, fields) {
for(i in results){
for(j in results[i]){
last = results[i][j];
}
}
var time = Math.floor(new Date().getTime() / 1000);
if((time - last) > 86400){
con.query('UPDATE `voter` SET `lastvote`="'+time+'" WHERE `userid`="'+uid+'"', function (error, results, fields) {
steem.broadcast.vote(wifkey,votey,author,permlink,weight,function(downerr, result){
if(downerr){
setTimeout(function(){bot.createMessage(channel,'Already Upvoted!');},1000);
con.query('UPDATE `voter` SET `lastvote`="'+last+'" WHERE `userid`="'+uid+'"', function (error, results, fields) {
});
}
if(result) {
setTimeout(function(){bot.createMessage(channel,'Done! Your Post Upvoted By @mahdiyari');},1000);
}
});
});
}else{
var come = 86400 - (time - last);
setTimeout(function(){bot.createMessage(channel,'Sorry! Come back after '+come+' seconds.');},1000);
}
});
}else{
setTimeout(function(){bot.createMessage(channel,'You Are not Registered.');},1000);
}
}
}
});
}
var regex2 = /(\$)+(register)+(\ )/;
if(msg.content.match(regex2)){
var sender = msg.content.replace(msg.content.match(regex2)[0],"");
var memo = 'register';
var transaction = 'Receive 0.001 SBD from '+sender;
var r = 0;
var channel = msg.channel.id;
con.query('SELECT EXISTS(SELECT * FROM `voter` WHERE `userid` = "'+msg.author.id+'" OR `user`="'+sender+'")', function (error, results, fields) {
for(i in results){
for(j in results[i]){
x = results[i][j];
if(x == 1){
setTimeout(function(){bot.createMessage(channel,'Already Registered!');},1000);
}else if(x == 0){
var xmlhttp = new XMLHttpRequest();
xmlhttp.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
var response = JSON.parse(this.responseText);
for(i in response){
if(response[i].transaction == transaction && response[i].memo == memo){
con.query('INSERT INTO `voter`(`user`, `lastvote`, `userid`) VALUES ("'+sender+'","0","'+msg.author.id+'")', function (error, results, fields) {
r = 1;
setTimeout(function(){bot.createMessage(channel,'User '+sender+' Registered by <@'+msg.author.id+'>');},1000);
});
break;
}
}
setTimeout(function(){if(r == 0){
setTimeout(function(){bot.createMessage(channel,'Please Send 0.001 SBD to '+votey+' with memo:`'+memo+'` and Try again.');},1000);
}},2000);
}
};
xmlhttp.open("GET", "https://steemfollower.com/voter-tx.php?user=" + votey , true);
xmlhttp.send();
}
}
}
});
}
});
bot.connect();
Please Report if you get any error or any bug.
If you have any question, please ask in comments.
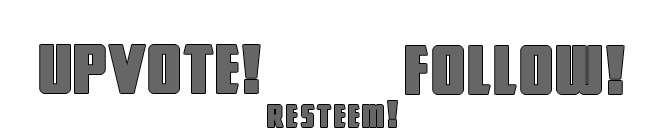
if you think I can be helpful for steem community, please vote me as a witness. Thanks.
1- open https://steemit.com/~witnesses
2- scroll down.
3- type mahdiyari
and once click on the vote button.
first image source: pixabay.com
Regards,
2017-09-19
Nice new post! Will add this feature to the discord bot. Can't wait for the multi bot post :D
Edit:
Added it to the discord channel. I guess i don't understand how the register works. when i put $register nothing comes up, unsure if anything is suppose to come up. I added the full code editing the details such as posting key, user, and bot token. Can you please explain how to register a user with the new code?
var xmlhttp = new XMLHttpRequest();
ending with error xmlhttprequest is not defined.
I think miss this in the beginning :
var XMLHttpRequest = require("xmlhttprequest").XMLHttpRequest;
yes, Thanks.
Inspiring. Love this. I am just starting to learn code.. :) @mahdiyari
thx master, for a very helpful article, I will apply this feature in my discord bot
its like the banjo bot
Thanks for this post! It helps a lot. Upvoted and Followed ^_^.
Thanks a lot for this updates
Great post about the technical aspects of a bot.
I haven't tried coding anything on the Steem blockchain.
I guess it is time to start XD
Thanks for sharing :)
Thank you @mahdiyari .This guide will help us.
This post has been ranked within the top 50 most undervalued posts in the second half of Sep 19. We estimate that this post is undervalued by $31.04 as compared to a scenario in which every voter had an equal say.
See the full rankings and details in The Daily Tribune: Sep 19 - Part II. You can also read about some of our methodology, data analysis and technical details in our initial post.
If you are the author and would prefer not to receive these comments, simply reply "Stop" to this comment.