Complete Guide to Creating Discord Auto Upvote Bot by Javascript.
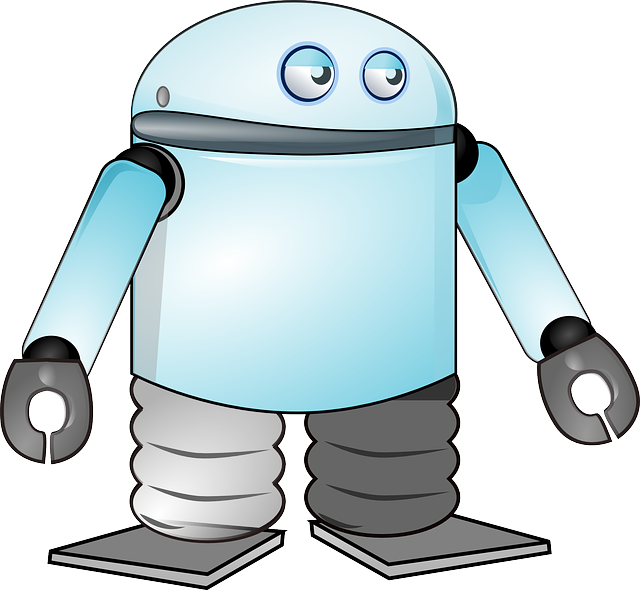
It is a complete guide for creating an auto voter bot like banjo or minnowsupport or like 'Steemfollower-voter' bot in this discord server
For running a discord bot, you need a VPS or a always online system.
minimum requirment system is 200~500 mb RAM.
How it works?
this bot can work with a spicific commands like $upvote or without any command in spicific channels.
we will user Eris Discord library to connecting our bot. you can find this library here on github.
After upvoting a post, we will store that Discord User in Mysql Database with upvote time. we will need this time for checking next upvotes time.
Our bot will detect commands and post links, then it will check last upvote time for that user. if it was greater that 12 hours (you can change this) then post will get an upvote from spicific account. or User will receive an error message.
First Step: Installing Mysql
this guide is for ubuntu 16.04
if you are using another IOS or another version, Search in Google.com
Start by entering these commands:
sudo apt-get update
sudo apt-get install mysql-server
You'll be prompted to create a root password during the installation. Choose a secure one and make sure you remember it, because you'll need it later. Next, we'll finish configuring MySQL.
Run the security script.
sudo mysql_secure_installation
This will prompt you for the root password you created in recent step. You can press Y and then ENTER to accept the defaults for all the subsequent questions, with the exception of the one that asks if you'd like to change the root password. You just set it in Step 1, so you don't have to change it now.
Finally, let's test the MySQL installation.
systemctl status mysql.service
Creating Database:
Once you have MySQL installed on your droplet, you can access the MySQL shell by typing the following command into terminal:
mysql -u root -p
Enter your mysql password and press 'Enter'.
Now we need to create a new database:
CREATE DATABASE database voter;
it will create 'voter' database. Don't forget ;
at the end of codes.
Let’s open up the database we want to use:
USE voter;
Now create a new Table:
CREATE TABLE
voter
(
id
int(11) NOT NULL,
user
text NOT NULL,
lastvote
text NOT NULL,
userid
text NOT NULL);
or If you get any error, Try this one:
CREATE TABLE `voter` (
`id` int(11) NOT NULL,
`user` text NOT NULL,
`lastvote` text NOT NULL,
`userid` text NOT NULL);
Creating a Discord Bot:
Open: https://discordapp.com/developers/applications/me
and Create New Application.
Follow this instruction and Create a new application and a new bot and add that bot to your server.
We will use bot token in our .js file.
Installing Required Libraries
Run These codes one by one:
sudo apt-get install nodejs
sudo apt-get install npm
npm install steem --save
npm install --no-optional eris
npm install mysql
npm install pm2
Creating .js File
Create a .js file in your system and add this codes.
you can do it by:
nano voter.js
we need to add some library to our file:
const Eris = require("eris");
const steem = require("steem");
var mysql = require('mysql');
Mysql Connection Details:
var con = mysql.createConnection({
host: "127.0.0.1",
user: "root",
password: "mysql",
database: "voter"
});
And connecting to Mysql:
con.connect();
Enter your Voter account name and private Posting key. and add voting period.
var wifkey = 'myprivatepostingkey';
var votey = "mahdiyari";
var per = 86400;
86400 seconds = 24 hour.
Add Voting weight. (10000 = 100% and 100 = 1%)
var weight = 2000;
Add your Bot Token here:
var bot = new Eris("Your Bot Token");
We need to know when our bot is connected and is ready:
bot.on("ready", () => {console.log('voter bot started! weight is '+weight/100+'%);});
now we must detect a spicific command and posts permlinks and Author:
var regex = /(\$)+(upvote)+.+(https:\/\/)+.+(@)+.+(\/)/;
var regex1 = /(@)+.+(\/)/;
regex will check if message includes '$upvote https://steemit.com/@-/' or not.
and regex1 will export post Author.
Full Code:
const Eris = require("eris");
const steem = require("steem");
var mysql = require('mysql');
var con = mysql.createConnection({
host: "127.0.0.1",
user: "root",
password: "mysql",
database: "voter"
});
con.connect();
var bot = new Eris("your bot token");
var regex = /(\$)+(upvote)+.+(https:\/\/)+.+(@)+.+(\/)/;
var regex1 = /(@)+.+(\/)/;
var wifkey = 'private posting key';
var votey = "mahdiyari";
var weight = 2000; // 10000 = 100%
var per = 86400; // 86400 seconds = 24hour
bot.on("ready", () => {console.log('voter bot started! weight '+weight+' %');}); //when it is ready
bot.on("messageCreate", (msg) => { // when a message is created
if(msg.content.match(regex)){
var permlink= msg.content.replace(msg.content.match(regex)[0],"");
var au = msg.content.match(regex1)[0];
var aut = au.replace("@","");
var author = aut.replace("/","");
var channel = msg.channel.id;
var uid = msg.author.id;
var x = '0';
con.query('SELECT EXISTS(SELECT * FROM `voter` WHERE `userid` = "'+uid+'")', function (error, results, fields) {
for(i in results){
for(j in results[i]){
x = results[i][j];
if(x == '1'){
var last;
con.query('SELECT `lastvote` FROM `voter` WHERE `userid`="'+uid+'"', function (error, results, fields) {
for(i in results){
for(j in results[i]){
last = results[i][j];
}
}
var time = Math.floor(new Date().getTime() / 1000);
if((time - last) > per){
con.query('UPDATE `voter` SET `lastvote`="'+time+'" WHERE `userid`="'+uid+'"', function (error, results, fields) {
steem.broadcast.vote(wifkey,votey,author,permlink,weight,function(downerr, result){
if(downerr){
setTimeout(function(){bot.createMessage(channel,'Already Upvoted!');},1000);
con.query('UPDATE `voter` SET `lastvote`="'+last+'" WHERE `userid`="'+uid+'"', function (error, results, fields) {
});
}
if(result) {
setTimeout(function(){bot.createMessage(channel,'Done! Your Post Upvoted By @mahdiyari');},1000);
}
});
});
}else{
var come = per - (time - last);
setTimeout(function(){bot.createMessage(channel,'Sorry! Come back after '+come+' seconds.');},1000);
}
});
}else{
var time = Math.floor(new Date().getTime() / 1000);
con.query('INSERT INTO `voter`(`user`, `lastvote`, `userid`) VALUES ("'+author+'","'+time+'","'+uid+'")', function (error, results, fields) {
steem.broadcast.vote(wifkey,votey,author,permlink,weight,function(downerr, result){
if(downerr){
setTimeout(function(){bot.createMessage(channel,'Already Upvoted!');},1000);
con.query('UPDATE `voter` SET `lastvote`="10" WHERE `userid`="'+uid+'"', function (error, results, fields) {
});
}
if(result) {
setTimeout(function(){bot.createMessage(channel,'Done! Your Post Upvoted By @mahdiyari');},1000);
}
});
});
}
}
}
});
}
});
bot.connect();
Running Bot:
You must Add account username, posting private key, voting weight and Bot Token before running this file.
for running your bot, enter:
pm2 start voter.js
if you edited your file anytime, you must restart it by:
pm2 restart voter.js
and for stopping:
pm2 stop voter
'voter' is my .js file name. change it if your file is different.
You can add a channel filter fo your bot, so your bot will work only in spicific channels.
Also, in this case, my Mysql password for root user was 'mysql'. so change it in above codes.
Ask your questions in comments or join Steemdevs discord server.
if you think I can be helpful for steem community, please vote me as a witness. Thanks.
1- open https://steemit.com/~witnesses
2- scroll down.
3- type mahdiyari
and once click on the vote button.
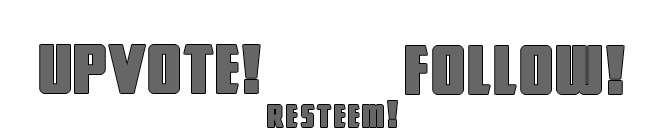
first image source: pixabay.com
Regards,
2017-09-06
Does a hosting server work for this?
you need a linux system. a VPS or server.
use heroku to desploy the script
thanks @mahdiyari , realy i need it
hi @hdmed, are you success make that bot running? because i am traying on my own computer running kali linux don't work,.. i don't know why..
hi i made one without mysql i will put it in my , now server, now i made some change in my bot @morwhale for more options in the future, thanks for asking @binjeeclick
Awesome @hdmed . thanks
What is a bot token? I am going to try to set one of these up tomorrow.
you can find bot token after creating a bot for your application
I think information in article is enough.
Hi again. Im trying to set this up and i have followed all the instructions. I copied your full code and edited it. Set up the bot on discord. But when i go to turn it on with pm2 start voter.js it says the command is not found. I have restarted the whole process and still getting the same error that pm2 is not a command.
you must install all requirments
check again this article.
you must install pm2 before starting it.
npm install pm2
i tried this. however i am getting an error when trying to install npm install steem --save. But either way npm install pm2 does not fix that there is no pm2 command. Should i wipe the server and try to start over?
I used this bot on 4 ubuntu system. and all of them works fine.
I am doing this on a VPS i got from AWS for free. I will try to set it up on a VM on my main machine. Also thank you for your replys. To mention i did get one error when following your steps. The database part "CREATE DATABASE database voter;" i removed the database part to get it to work.
Oh, I used 2 'database'. Thanks.
I think you missed something. try this codes and then install requirments.
I tried on a vm and get the same exact thing. It says when i install pm2 that 'npm WARN optional Skipping failed optional dependency /chokidar/fsevents:'
'npm WARN Not compatible with your operating system or architecture : [email protected]'
also that there is no file or directory package.json
i don't know if this is my problem or not. I followed your directions to a Tee. Can you please help me.
really awesome stuff. Bookmarked for one day.
I should have stayed in school...
Nice method for auto upvote. Resteemed and upvoted.
Upvoted And Resteemed...
thx for good article, this article I am looking for, i want to build my own upvote bot on discord.. very helpful,..
upvote and resteem
great
This is the best article ever. I have decided to take a boot-camp coding course for 6 months so I can understand the terminology and script better.