Smart contracts - Part 3 - How it's done
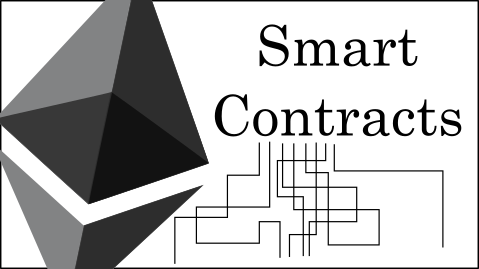
Hi Steemians, how are you? The contest has already ended... unfortunately it did not have much reception despite the fact that the deadline was one week. Surely the prize of 0.03 ETH was not so tentative as to take the time to participate and it is also true that you have to pay gas to participate, even if it is little!
To end this cycle on ethereum, I want to show you the smart contract code, which I have tried to make it as simple as possible to explain it and that anyone can understand it even if they do not have programming knowledge.
Again I explain the main idea of the contract: Anyone can participate and to do so send 0 ETH so that their address can be registered. The winner will be the 50th participant, or the last one who participated before a deadline (which I defined as September 9).
Here goes the code:
pragma solidity ^0.4.0;
contract countGame {
address public best_gamer;
uint public count = 0;
uint public endTime = 1504969200;
function fund() payable {
require(now <= endTime);
}
function (){
require(now<=endTime && count<50);
best_gamer = msg.sender;
count++;
}
function endGame(){
require(now>endTime || count == 50);
best_gamer.transfer(this.balance);
}
}
I will explain it step by step. First we have:
pragma solidity ^ 0.4.0;
This line of code should always be in all contracts and refers to the version of solidity that we are using so that the compiler understands the format in which it is written.
Then comes contract countGame
which defines the name of our contract and inside the brackets {}
we write its content.
Now we define 3 variables, which are the ones that will help us store the relevant information. We need an address (address public
) that we will call best_gamer
, and that is where we will store the address of the last participant, which will be the winner candidate, since the previous participants no longer interest us. Then we need a positive integer uint public
that we will call count
and we use it to count how many participants there have been so far. Finally we need an integer number uint public
that we will call endTime
that defines the game's deadline. Thus we have:
address public best_gamer;
uint public count = 0;
uint public endTime = 1504969200;
It is important to note that the dates are defined in unix time, which is a counter of seconds to facilitate computer calculations. To calculate any date we can use this tool, from which I obtained that September 9 at 3pm GMT corresponded to the second 1504969200.
After this we define 3 functions that will allow us to completely define the game. We go with the first, called fund
function fund() payable {
require(now <= endTime);
}
Every time this function is called, what we want is that the contract receives money, it is the way to receive funds. That is why it is necessary the word payable
in it. Within it, we only have to say that money is received only if the contest is still ongoing, that is, if now
(current moment), it is less than endTime
, the date that we define as the limit. If this is not fulfilled money is never transferred. This was the function that I used to send 0.03 ETH to the contract.
The second function has no name and we define it like this:
function (){
require(now<=endTime && count<50);
best_gamer = msg.sender;
count++;
}
This is the function with which the participants interact. Every time someone interacts with a contract they must choose the function they want to interact with, however, if no function is defined or their name does not match any of the list then the unnamed function is executed. Since participants usually have wallets that do not support interaction with smart contracts, it is best to define it here and run it simply by sending 0 ETH to the contract.
What does this function contain? first we make sure that the time limit has not passed now <= endTime
, and that the counter of participants is less than 50. The way to concatenate these two conditionals is done with &&
, which means "AND".
require(now<=endTime && count<50);
After this condition we change our variables: The winner candidate will be the address of who is participating, in ethereum it is defined as msg.sender
, and means "from the message msg
and take the address of the sender
". And finally we increase the counter by 1, and it is done using ++
.
best_gamer = msg.sender;
count++;
In this way, each time someone participates, their address is stored and the counter increases. Let's go with the last function.
function endGame(){
require(now>endTime || count == 50);
best_gamer.transfer(this.balance);
}
This function called endGame
we use to deliver the prize to the winner. First we make sure that the game is over, this happens when the time limit has already passed now> endTime
, or when the counter has reached 50, count == 50
, the way to join these conditions is done with ||
, which means "OR".
require(now>endTime || count == 50);
and finally if this has been fulfilled, we transfer the total amount of money that the contract has (this.balance
) to the last participant (best_gamer
).
best_gamer.transfer(this.balance);
With this we have finished defining our smart contract.
How to compile and publish it?
To compile the contract we can enter to https://remix.ethereum.org/, an online tool that is very useful for simple contracts, and it is not necessary to install anything. There we can compile and at the same time make tests to see that it works correctly.
Once we have it ready and compiled, the system will throw us a series of data and information, from there we must take the content of BYTECODE, which will serve us to publish.
To publish the contract there are also several tools, the simplest in my view is https://www.myetherwallet.com/. There we go to Contracts and then Deploy Contract. We wrote the ByteCode there, we paid the gas for publishing, which in my case was $2.
Good Post.
thanks
A good contest for sure