Coding challenge #1 - Winners!
Last week I posted my first contest with a coding problem to solve.
Now it's time to acknowledge and reward the winners!
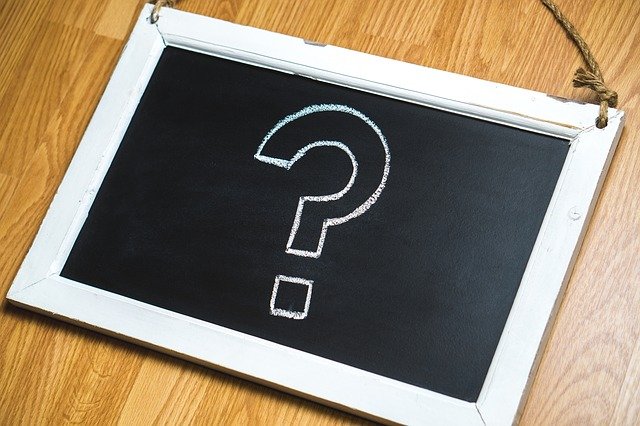
Contest #1
- Write a function that take as argument a char array and modify it so that the order of the letters is reversed.
- For instance: 'abc def' ---> 'fed cba'
- Write a function that take as argument a char array and modify it so that the order of the letters in the words are reversed.
- For instance: 'abc def' ---> 'cba fed'
You can assume the separator between words is always the space (no comma, dot, ...)
The preferred language to use is Java, but you can also use other languages as well. You will need to translate the starting code to the language of your choice. Don't use languages where you can solve the problem natively in one line ;)
Winners
There were 3 participants and all of them solved the problems well!
The first place goes to @hendrikcrause: he did a very good job and the code is very well written.
The second and third prizes will be divided equally between @chasmic-cosm and @pps because I couldn't choose a winner between them.
@chasmic-cosm did a great job, in javascript, but his code was not working in a special case where there were words separated by two spaces.
@pps solved the problem in C, it works, but his solution was a bit overcomplicated. @pps have a look at the Best solution here to see how it could have been done.
Anyway, great job guys! Hope to see your solutions to my future contests too!
Best solution
class Main {
/*
* Example: 'abc def' --> 'fed cba'
*/
public static void reverseAll(char[] text) {
reversePartial(text, 0, text.length - 1);
}
/*
* Example: 'abc def' --> 'cba fed'
*/
public static void reverseWords(char[] text) {
int i = 0, j = 0;
while (j < text.length) {
while (j < text.length && text[j] != ' ') {
j++;
}
reversePartial(text, i, j - 1);
i = ++j;
}
}
private static void reversePartial(char[] text, int start, int end) {
int i = start, j = end;
while (i < j) {
swap(text, i++, j--);
}
}
private static void swap(char[] text, int i, int j) {
char temp = text[i];
text[i] = text[j];
text[j] = temp;
}
/***************************/
/***************************/
/* DO NOT CHANGE DOWN HERE */
/***************************/
/***************************/
public static void solve(String text) {
System.out.println("Reversing string: " + text);
char [] reversedAll = text.toCharArray();
reverseAll(reversedAll);
System.out.println(String.format("Result after reversing all: %s", new String(reversedAll)));
char [] reversedWords = text.toCharArray();
reverseWords(reversedWords);
System.out.println(String.format("Result after reversing the words: %s", new String(reversedWords)));
System.out.println();
}
public static void main(String[] args) {
solve("abc def");
solve("This is a sentence I want to reverse");
}
}
Rewards
The contest post total liquid reward is: 7.210 SBD
- 1st price: 50% ---> 3.605 SBD
- @hendrikcrause --- 3.605 SBD
- 2nd-3rd prize: 25% + 12.5% = 37.5% ---> 2.703 SBD
- @chasmic-cosm --- 1.351 SBD
- @pps --- 1.351 SBD
Thanks for taking part of the challenge!
Armando 🐾
Thanks @armandocat,
For anyone interested in knowing how it works, here's a - hopefully - quick description.
So the two public methods were what needed to be implemented, for my implementation I needed two private helper methods:
swap
andreversePartial
.swap
should hopefully be easy to understand, it simply takes an array and two indexes and then "swaps" the array's characters at the index positions.reversePartial
is a bit more complicated, but simple enough to grasp I believe. It takes in an array and two index as well and then reverses the order of all the characters in the array between the two indexes. The tricky / clever part about it is that you can reverse an array more efficiently by swapping the first and last character and then the second and second to last, etc. I made this handy gif below to demonstrate.So now the stars of the show...
reverseAll
becomes trivial to solve, since it is simply areversePartial
with the first and last index of the array.reverseWords
is a bit more involved. Here we have to loop through the array until we find a space character. Then do areversePartial
between the index before the space and the index after the last space found. I keep track of the last space found with integeri
and the current place I'm checking with the integerj
.Thanks @armandocat for hosting the competition, was a fun little exercise.
Thanks @hendrikcrause, very nice and detailed explanation!
Thanks for explanation
Thanks for the perfect solution.
Thanks for the challenge!