How do you Unit Test a Simple Getter and Setter Interface in Java?
Usually, we unit tests the logics. An interface is without implementation details. A interface is just a binding a contract, but still, we can use Mockito to mock the interface, and test it.
For example, given the following simple Setter and Getter Interface:
interface GetAndSet {
void setValue(String name);
String getValue();
}
We can test it like this - thanks to the Mockito mocking framework in Java. We use doAnswer method to intercept the invokation of a interface method i.e. setter, then at the time, we mock the getter.
package com.helloacm;
import lombok.val;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.mockito.Mockito.*;
interface GetAndSet {
void setValue(String name);
String getValue();
}
public class ExampleTest {
@Test
public void test_simple_getter_setter_interface() {
val instance = mock(GetAndSet.class);
doAnswer(invocation ->; {
// or use invocation.getArgument(0);
val name = (String)invocation.getArguments()[0];
when(instance.getValue()).thenReturn(name);
return null;
}).when(instance).setValue(anyString());
instance.setValue("HelloACM.com");
assertEquals("HelloACM.com", instance.getValue());
}
}
If the method we are mocking is not void - we can use when. For example:
when(instance.getValue()).thenAnswer(innocation -> {
return "Hello";
});
Every little helps! I hope this helps!
Steem On!~
Reposted to The Blog of Computing
If you like my work, please consider voting for me, thanks!
https://steemit.com/~witnesses type in justyy and click VOTE
Alternatively, you could proxy to me if you are too lazy to vote!
Also: you can vote me at the tool I made: https://steemyy.com/witness-voting/?witness=justyy
Done
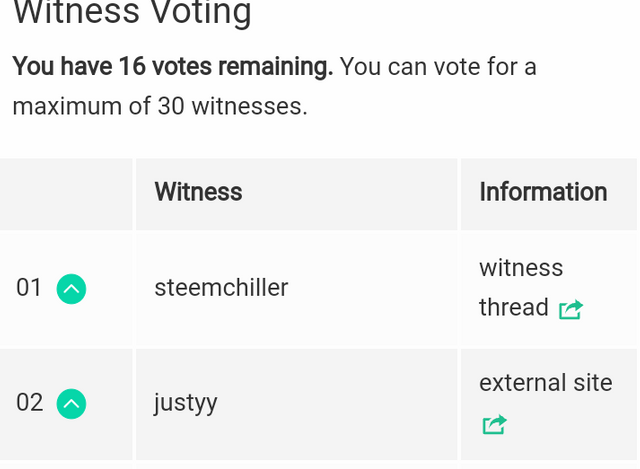
Thank you very much!
You are welcome, sir!