Building the blockchain using JavaScript
The blockchain is a data structure that comprises blocks of data that are chained together using a cryptographic hash. In this article, we shall attempt to build a blockchain prototype using JavaScript. This is a bit technical for non-technical people but should be a piece of cake for computer nerds.
First of all, let's examine the content of a block. A block consists mainly of the block header containing metadata and a list of transactions appended to the block header. The metadata includes the hash of the previous block, the hash of the block, timestamp, nonce, difficulty and block height. For more information, please refer to my earlier article Blockchain in a Nutshell.
Prior to writing the code, you need to install the following software:
- Chocolatey
- Visual Studio Code
- node.js
The installations are based on Windows 10, but you can do the the same thing easily in Ubuntu. Chocolatey is a software management solution for Windows, Visual Studio Code is a streamlined code editor with support for development operations like debugging, task running and version control and Node.js is an open source server environment.
Let's start writing the code using Visual Studio Code. The first line is
const SHA256 = require("crypto-js/sha256");
This code meas we are using the JavaScript library of crypto standards. We require the crypto-js library because the sha256 hash function is not available in JavaScript.The crypto module provides cryptographic functionality.
SHA-256 is a cryptographic hash algorithm. A cryptographic hash is a kind of 'signature' for a text or a data file. SHA-256 generates an unique 256-bit signature for a text. SHA256 is always 256 bits long, equivalent to 32 bytes, or 64 bytes in an hexadecimal string format. In blockchain hash, we use hexadecimal string format, so it is 64 characters in length.
Next, we create the block using the following scripts:
class Block {
constructor(index, timestamp, data, previousHash = '') {
this.index = index;
this.previousHash = previousHash;
this.timestamp = timestamp;
this.data = data;
this.hash = this.calculateHash();
}
The class Block comprises a constructor that initializes the properties of the block. Each block is given an index that tells us at what position the block sits on the chain. We also include a timestamp, some data to store in the block, the hash of the block and the hash of the previous block.
We also need to write a function calculateHash() that creates the hash, as follows:
calculateHash() {
return SHA256(this.index + this.previousHash + this.timestamp + JSON.stringify(this.data)).toString();
}
}
Finally, we write the script that creates the blockchain, as follows:
class Blockchain{
constructor() {
this.chain = [this.createGenesisBlock()];
}
createGenesisBlock() {
return new Block(0, "01/01/2017", "Genesis block", "0");
}
getLatestBlock() {
return this.chain[this.chain.length - 1];
}
addBlock(newBlock) {
newBlock.previousHash = this.getLatestBlock().hash;
newBlock.hash = newBlock.calculateHash();
this.chain.push(newBlock);
}
Notice that the class blockchain comprises a constructor that consists of a few functions, createGenesisBlock(), getLatestBlock() and addBlock(newBlock).
Save the file as myblkchain.js or any name you like.
Now, execute the file in the VS terminal using the following command
node myblkchain.js
The output is as follows:
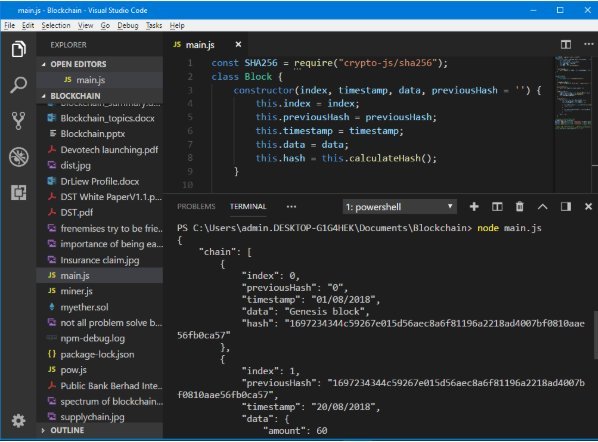
Let’ examine the output. Notice that each block comprises the properties index, previous hash, data and hash.
The index for the Genesis is always 0 . Notice that the hash of the genesis block and the previous hash of the second block are the same. It is the same for other blocks.
References
- https://www.savjee.be/2017/07/Writing-tiny-blockchain-in-JavaScript/
- https://nodejs.org/api/crypto.html
- https://www.savjee.be/2017/07/Writing-tiny-blockchain-in-JavaScript/
Posted from my blog with SteemPress : http://www.blockchainguide.biz/building-a-blockchain-using-javascript/
好高深,但我看完了,作者辛苦了