Altcoin Portfolio The Pythonic Way - Have FUN & LEARN a New Skill!
I thought I would share a simple method of querying CoinMarketCap for altcoin prices via their simple API using Python.
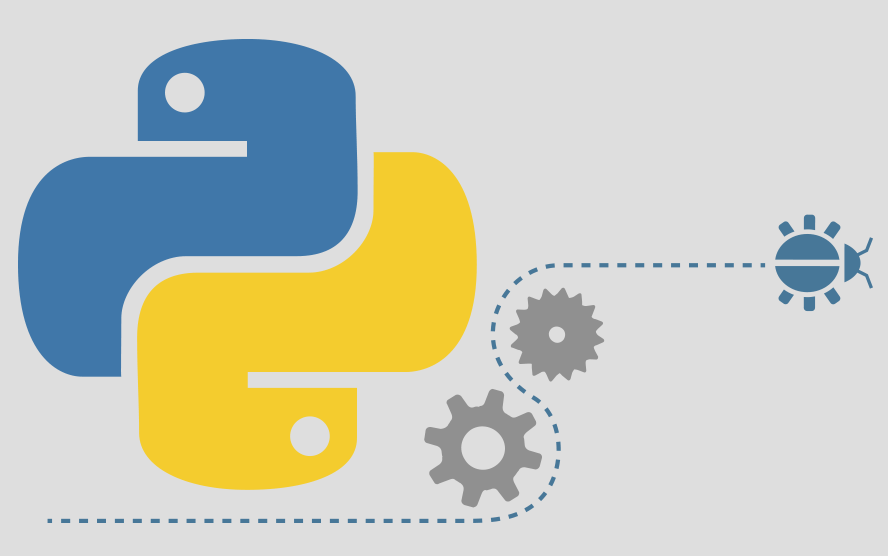
If you have never used Python before then this is for you! Python is a powerful programming language which you all should take the time to learn the basics. Once you understand the basics, you can do so many things!
Learning Python is a great way to advance your knowledge using computers and to become more sophisticated. This simple altcoin portfolio introduction will help you start your own projects.
Lets get everything setup
Firstly, lets get the environment all setup on your computer. These steps should be about the same if you are using Windows or Mac.
- Head over to Anaconda Python and install it. Windows or Mac. Make sure to choose Python 3.x and complete the install.
- Anaconda has the Jupyter Notebook built in. The Jupyter notebook provides a simple and clean interface for writing and testing out Python code. The notebook makes it easy to share with others as well.
- If you are using Windows then click on "start" and type "cmd". Click the cmd application. If you are using Mac then search for "terminal" and click the application. When either the cmd or terminal windows is open, type "jupyter notebook" and press enter to to launch it.
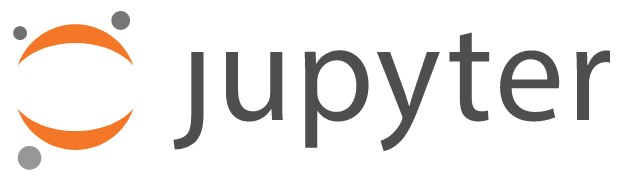
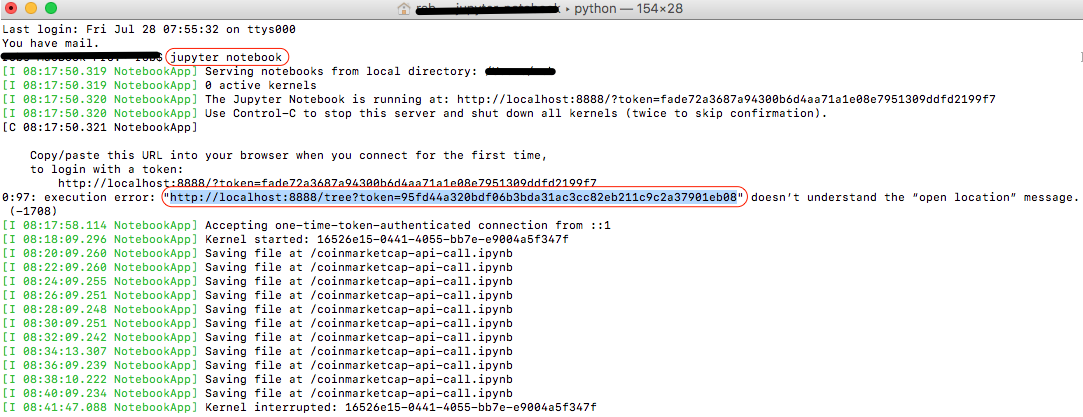
The notebook should automatically launch itself in your default browser (chrome for me). If it doesn't launch, then you will have to manually copy the link provided and paste into your browser. The link is shown after you press enter, see above picture for highlighted link.
You should now have the Jupyter notebook up and running! You should be looking at a browser window like below.
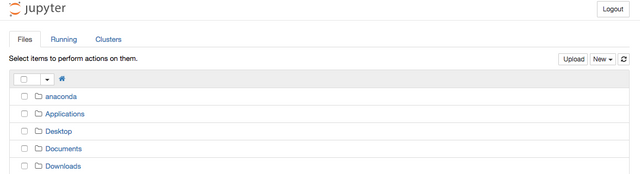
Start a new project and lets go!
To start a new project, simply click "new" in the upper right hand corner and make sure to click "python 3".
Now we have a new Jupyter project, lets start coding Python!
We will be referencing Coinmarketcaps API for altcoin ticker prices.
Specifically we will be calling https://api.coinmarketcap.com/v1/ticker/ for our script to parse.
In Python we need to import some basic libraries and call the API and get the response.
import requests
import json
url = 'https://api.coinmarketcap.com/v1/ticker/'
r = requests.get(url)
data = json.loads(r.text)
It should look like this. Press shift + enter to execute the current cell.
In the next cell we will let Python know what tickers we are interested in looking at as well as how many coins owned. I will be using made up numbers for this and is not my portfolio :)
ids = ['ethereum', 'gambit', 'decred', 'digibyte', 'nimiq', 'iota', 'mobilego', 'neo', 'monero', 'edgeless']
quantities = [20, 400, 101, 100000, 300, 766, 300, 1000, 44, 50]
Finally in the third cell we will parse the data and calculate prices then print them to the screen.
i = 0
balance = 0
print("SYM \t Price \t\t Amount \t Total BTC")
for d in data:
sym = d['symbol']
name = d['id']
if name in ids:
price = float(d['price_btc'])
amount = quantities[ids.index(d['id'])]
total = float(d['price_btc'])*amount
print("%s \t %0.8f \t %i \t\t %0.2f" % (sym, price, amount, total))
i += 1
balance += total
print("\nBTC Total: %0.3f" % balance)
The final result!
The final project is shown below. You can see the Jupyter notebook printed out the prices and totals for each coins. It also printed the total balance in BTC!
Please take your time to look over each line of this code and familiarize yourself on what each one does. Learning this simple script will make your Python skill powerful!
Personal challenge
Can you add a fancy pie chart to this script to add a graphical view? Hint, look at matplotlib.
🚀🚀🚀 Thanks for Reading 🚀🚀🚀
Follow, Resteem and VOTE UP Crypto & Tech Lovers |
![]() |
Its been recommended by a friend the Pandas might work well for this project.
https://pandas.pydata.org/pandas-docs/stable/index.html
I suggest to also add python tag
Just did! Thank you sir.
Thank you (from Python beginner) for your example and I hope more will be coming :-) This one was very useful for me.
I was just wondering how to get certain data from coinmarketcap. I knew I can loop through the dictionaries inside that json and print out data like this: (example is for STEEM)
print(data[19]['symbol'], data[19]['price_usd'])
But I was wondering, how to make sure to always get data for say STEEM, because when STEEM changes rank and ends on say 18th place, upper line of code will return data for different crypto currency.
Well, you just showed me, how to elegantly solve this by creating list of id's I want data for and then get the info with:
if name in ids:
Cool, solves the problem currencies changing their 'rank', their position.
Thanks, very appreciated!
Glad the example was helpful to you. Full Steem ahead :)
Did you figure out how to add the pie chart?
Sorry, haven't touched computer since. I'm not ready for matplotlib yet anyway, maybe in a year, :-)
I'm old & slow learner with not enough free time...