Using Stylus to Build a Web Framework Like Bootstrap and Foundation
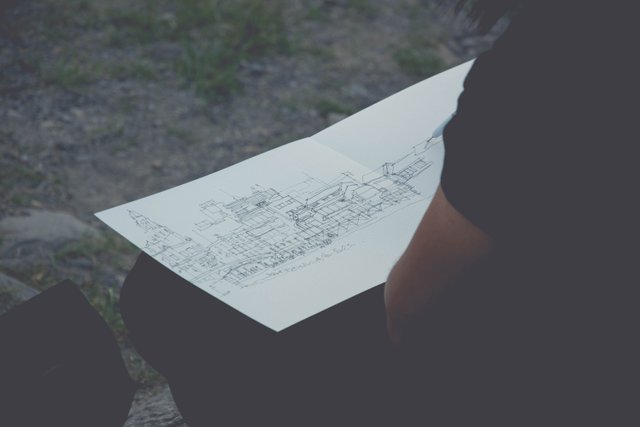
What is Stylus?
To begin with, Stylus is a CSS preprocessor like SASS or LESS with much of the same basic functionality. However, using Stylus offers a cleaner - easier to read and understand syntax.
To begin with, I believe the best way of learning, is through building. Which is why I will be walking you through the process of building a simple CSS grid framework using Stylus to generate it.
History of CSS Web Framework Design
In the past, we built CSS frameworks using lots of copy and pasting of much of the same code with slight variations. Without a doubt, this made updating and managing the frameworks repetitive and time consuming.
However, now with CSS preprocessors and the help of automation tools like NPM, Gulp and Grunt we can automate the process and rebuild them within seconds.
Blueprint (The First Web Framework)
To begin with, Blueprint was created by Olav Bjørkøy and released on August 3, 2007. Blueprint includes a 24 column “span” grid layout. Blueprint didn’t support mobile displays by default.
YAML and Cascade CSS Framework Soon Followed Behind
Comparatively, Cascade was one of the first CSS web frameworks to support responsive design. However, it required multiple different CSS files which increased page load time.
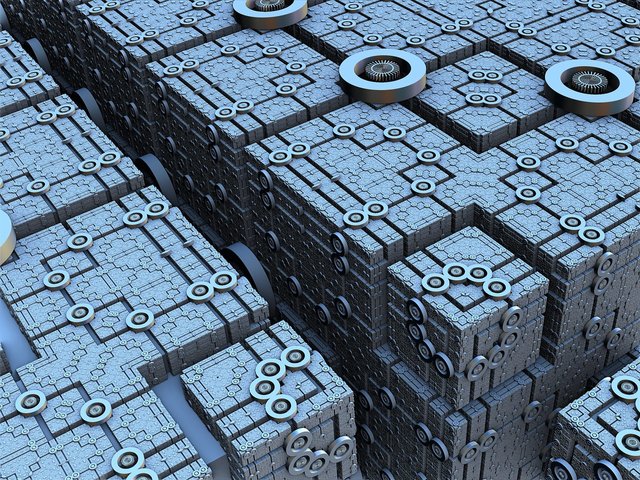
Popularity of Bootstrap and Grid Frameworks
Grid frameworks allow designers to easily layout content on web pages. In addition, grids can be resized depending on different screen sizes with the use of media queries introduced in CSS3.
Media Queries and Grid Frameworks
As you may know, Bootstrap CSS framework offers classes ranging from “col-xs-X” to “col-xl-X”. (X representing the column size.) By way of example, these column names start with “xs” and “xl” to dictate extra-small and extra-large screen sizes.
But why use these class names?
By using these descriptive class names you can easily dictate the width of elements for all different device sizes.
How to Use Stylus to Build a Grid Based Web Framework Like Bootstrap or Foundation
Creating a Basic CSS Grid Framework Using Stylus
In order to create a CSS grid using Stylus, you must first understand how the Stylus for statement works. By way of example, here’s how you would build a basic grid using the Stylus the "for" statement. Granted, due to this being just a basic grid, there is no support for responsive column sizes just yet.
[code lang="text"]
//Default grid settings
columns = 12
gutter = 15px
//Set all elements to border-box
*, *:before, *:after
box-sizing: border-box
.container
float: left
padding: 0 gutter
width: 100%
.row
float: left
margin-left: -+gutter
margin-right: -+gutter
width: 'calc(%s + %s)' % (100% gutter*2)
[class^='col-'], [class*=' col-']
border: solid rgba(0,0,0,0.15) 1px
float: left
padding-left: gutter
padding-right: gutter
for i in (1..columns) {
.col-{i} {
col-width = 100%/(12/i)
width: col-width
}
}
[/code]
This type of CSS grid utilizes a row to negate the left padding of the first column and right padding of the last column. Coupled with, the [class*=col-] part of this is saying all class names that begin with “col-“ will be affected with the internal styles.
We’re ensuring the box-sizing is set to border-box to keep the border, margin and padding within the inside of the columns. Float is necessary to keep elements in a row to fill the 12 column space. I set padding on the left and right of columns to the gutter-size. It’s important to keep these values matching the negative margin set in the .row class.
As for the “for i in (1..columns)” part… this is saying for every iteration from 1 until the defined column count (12), create that new column size.
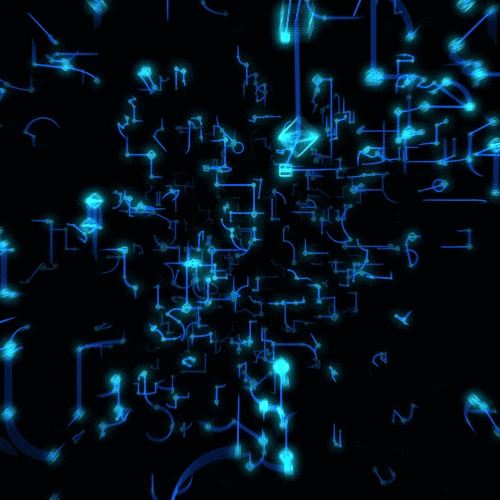
A Deeper Understanding of the Stylus For Loop
In the for statement near the bottom, we have a loop running from 1 to 12 as dictated by the “(1..12)” part. Equally important, there is a class using that iteration “i” for the column size “.col-{i}” - Which will be cycled through from 1 to 12 as “col-1” to “col-12” representing each column’s width.
Stylus allows for variables and logic, so we create a variable called “col-width” to equal the fraction of the 100% total each column should be. Being that, we simply set the width to that variable’s value.
Building a Responsive CSS Grid Framework Using Stylus
Furthermore, now that we have a basic understanding of how a CSS grid is built, we can move on to advanced grid development.
As you may know already, Bootstrap uses: col-sm-, col-md- and col-lg- class prefixes to control column sizes across devices.
col-sm-(size) will be set to the column size specified as long as the screen size is above 768px.
col—md-(size) will be set to the column size as long as the screen size is above 992px.
col—lg-(size) will be set to the column size as long as the screen size is above 1200px.
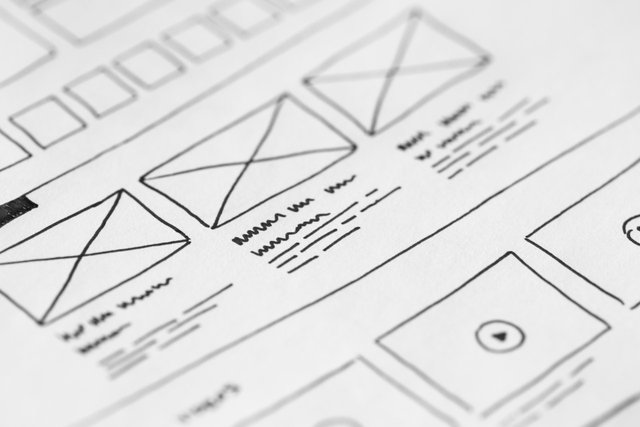
Building Media Queries for Responsive Column Sizing
For instance, this is the foundation of any mobile responsive CSS framework - Media Queries. By the same token, this code can be added to the basic framework code to give a fully functional CSS framework much like Bootstrap.
[code lang="text"]
//Setup screen sizes
screen-sizes = (sm md lg)
sm-min-size = 768px
md-min-size = 992px
lg-min-size = 1200px
//Cycle through screen sizes
for screen-size in screen-sizes
@media screen and (min-width: screen-size+'-min-size')
for i in (1..columns) {
.col-{screen-size}-{i} {
col-width = 100%/(12/i)
width: col-width
}
}
[/code]
In addition to cycling through column sizes, we are now cycling through the screen-sizes as well. Nonetheless, this will be the basis of our responsive media queries.
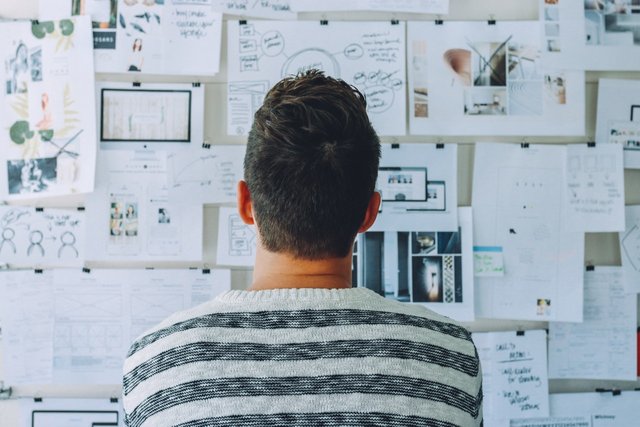
This can be tricky to understand, but it makes adding screen sizes to your framework easier than ever. For every screen-size array value, simply add the same screen size value + “-min-size”.
In addition, each media query is using the screen-name array value to retrieve the defined {screen-size}-min-size value.
Building CSS Stylesheets From Stylus Using Gulp
If you are a beginner in Gulp and using NPM packages, I recommend first reading Automate Your Tasks Easily With Gulp and Gulp for Beginners. These two articles will give you the basic understanding of the technology to follow through this next section.
Installing the Necessary Gulp Plugins
npm init
will generate our package.json file.On a mac or Linux machine, simply open up a terminal and change directory to your working folder. Then, paste
npm i -S gulp-stylus gulp-postcss gulp-autoprefixer gulp-sourcemaps
to install all necessary Gulp plugins.Setting Up the Gulp File for Automation
[code lang="text"]
'use strict';
let gulp = require('gulp');
let stylus = require('gulp-stylus');
let postcss = require('gulp-postcss');
let autoprefixer = require('autoprefixer');
let sourcemaps = require('gulp-sourcemaps');
gulp.task('default', ['slate']);
gulp.task('slate', function () {
return gulp.src('./styl/slate.styl')
.pipe(sourcemaps.init())
.pipe(stylus())
.pipe(postcss([require('autoprefixer')]))
.pipe(sourcemaps.write('./'))
.pipe(gulp.dest('./css'));
});
[/code]
What These Gulp Tasks Do:
pipe(sourcemaps.init())
is telling the Sourcemaps package to intiate.pipe(stylus())
will convert the target styl file to a CSS file. Read more about gulp-stylus.pipe(postcss([require('autoprefixer')]))
is responsible for adding piping other CSS generating Gulp plugins to a single CSS file. Notice, the autoprefixer plugin is piped through. Specifically, this plugin simplifies the process of adding the ‘-moz-‘ and ‘-webkit-‘ prefix to stylesheet attributes. Read more about gulp-postcss.pipe(sourcemaps.write('./'))
is telling the Sourcemaps package to create a source map for the stylesheet. Consequently, since we are compiling stylus files to CSS there is no way for us to find the error in our code easily using a browser inspector. For this reason, Sourcemaps builds a link to the styl files and instead pinpoints the line of code in the styl file when inspecting instead. Read more about gulp-sourcemaps.pipe(gulp.dest('./css'))
is telling Gulp where to place the compiled CSS file. In this case, we are placing the stylesheet in a folder called “css”.
How to Begin Using Our New CSS Framework
Just like linking to the Bootstrap CSS file, we will link to the outputted CSS file saved in the “css” folder. Further, using our generated class names like “col-xs-12 col-md-6” we can begin creating our mobile responsive columns.Now that you have a basic understanding of how CSS frameworks are made and how to start using Stylus, you can begin adding new features. Make your new framework as simple or advanced as you’d like!
Most importantly, share your new creation so that other web designers may use it and have fun
Feel free to leave a comment and share this article with your friends so that they can learn how to start using Stylus to build their very own CSS framework too!
Posted from my blog with SteemPress : https://divto.com/using-stylus-building-css-web-framework/