[AVLE] Handling CORS issue for Release
One issue to release the AVLE dapp is CORS (Cross-Origin Resource Sharing).
The request has been blocked by CORS policy
A request to get Tron data from steemwallet.com is blocked by this CORS policy.
It simply means the browser does not allow to fetch data from different domain which is 'avle.io' in this case.
Other than Tron related APIs have no CORS issue, meaning that steemapi.com allows access from different domain. I guess Tron APIs should allow it too.
There is a workaround for this issue, which is to use a proxy server. User's request from AVLE dapp to Tron api server is proxied in the middle. The CORS issue occurs only in a browser. So requesting from not a browser does not violet CORS policy.
Firebase Functions
Firebase provides cloud functions which act as a backend server (it is called serverless computing).
The followings are functions to handle requests from the AVLE dapp:
// fetch tron reward
exports.tronRewardRequest = functions.https.onCall((async (data) => {
console.log('[tronBalanceRequest] input data', data);
const { account } = data;
const tronRewardEndpoint = `https://steemitwallet.com/api/v1/tron/tron_user?username=${account}`;
let result = null;
try {
console.log('[tronBalanceRequest] tronRewardEndpoint', tronRewardEndpoint);
await axios.get(tronRewardEndpoint).then(
(response) => {
console.log('[tronRewardRequest] res.data', response.data);
result = response.data;
})
.catch((error) => console.log('failed to get tron reward. error', error));
} catch (error) {
console.log('failed to get tron reward, error', error);
return null;
}
return result;
}));
// fetch tron account data
exports.tronAccountRequest = functions.https.onCall(async (data) => {
console.log('[tronAccountRequest] input data', data);
const { address } = data;
const options = { 'address': address };
const tronAccountEndpoint =
'https://api.trongrid.io/walletsolidity/getaccount';
let result = null;
try {
const response =
await axios.post(tronAccountEndpoint, options);
console.log('[tronAccountRequest] response', response);
result = response.data;
} catch (error) {
console.log('failed to get tron account. error', error);
return null;
}
return result;
});
Now test this with release build
$ flutter run --release -d chrome
When we check the logs in Firebase Functions, it shows correct messages.
On step closer to the Release!
--
Please Vote for @etainclub as a Witness
I am @etainclub who develops several apps for steem such as Play Steem. Recently I have started to run a witness node. Please vote for me as a witness.
You can vote here:
https://steemitwallet.com/~witnesses
(find etainclub ranked at 37)
or here:
https://steemyy.com/witness-voting/?witness=etainclub&action=approve
cc.
@zzan.witnesses
@dev365
@xeldal
@steem
@steemitblog
@misterdelegation
@ranchorelaxo
@ben
@trafalgar
Resteem / Vote / Comment / Follow / Support
This project will Make STEEM Great Again! I, the developer of PLAY STEEM mobile app, am doing my best to bring people to the STEEM ecosystem.
Thank you for your support.
@steemcurator01
Upvoted! Thank you for supporting witness @jswit.
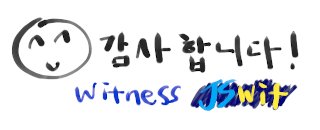
Greetings my friend @etainclub
Thank you for providing the greatest of efforts for the development of new applications, I already gave you my vote as a witness.
I hope you solve the problem soon
Your post is manually rewarded by the
World of Xpilar Community Curation Trail
STEEM AUTO OPERATED AND MAINTAINED BY XPILAR TEAM
https://steemit.com/~witnesses vote xpilar.witness
Are you sure that it's steemwallet API that's causing the CORS issue?
My tool makes use of the same API for a few calculations on the backend and I haven't faced any issues with this so far. I have faced issues with Tron APIs though as they are rate limited.
You could check out the staging instance of my app which has now been modified to use just the steemwallet API to fetch the Tron address.
https://www.steemincomereport.tech/incomecheck.
Just enter your account name and you should get the TRON address in the response.
This is the errror meesage:
Requesting in the backend has no issue. thanks.
it happens https site, too.
Oh. Damn! Not sure then.
Maybe try the tronscan APIs to get the account data?
https://apilist.tronscan.org