Create a forum application using django #6: CRUD system and URL protection,redirect system
Repository
What Will I Learn?
- CRUD system
- URL protection and redirect system
Requirements
- Basic Python
- Install Python 3
- Install Django
Resources
- Python - https://www.python.org/
- Django- https://www.djangoproject.com/
- Bootstrap 4 - https://getbootstrap.com/docs/4.0/getting-started/introduction/
Difficulty
Basic
Tutorial Content
I will continue the tutorial series on forum applications that use Django. there are some things that we have done and we have done, you can see them in the curriculum section. Until now the application that has been made already has an authentication, a slug system, and several other features. In this tutorial, we will create a CRUD(Create, read, update and delete) system. Of course, the CRUD system in each application can be different. Then how is it implemented in our application, let's start this tutorial
CRUD System
The applications that we make relate to data and information, of course, data that is made not dynamic in the meaning can be changed over time, with dynamic data and always increasing, we need to create a system that can manage data to be added, deleted and edited. In the previous section we have succeeded in creating a simple form that aims to create a forum on our application, here is the view that we can see:
- Insert data
In the previous tutorial, we created a class that will be used in the URL 'forum/add'
. There are 5 fields that we must fulfill to enter data into the forum table. They are title, desc, created_at, slug, and user.
We have got the title, desc, and created_at fields from the user input.
We have got the slug field from the generate slug we have made in the previous tutorial.
Now we have to fulfill the user field, we can get the value from the request. For that, we will create a new function that will fill that field. Here is the code:
forums/views.py
from django.shortcuts import render
from django.views.generic.edit import CreateView
from .models import Forum
class ForumCreate(CreateView):
model = Forum
fields = ['title','desc']
def form_valid(self, form):
form.instance.user = self.request.user
return super().form_valid(form)
def form_valid(self, form)
: In this function we will receive 2 parameters. The first parameter is self and the second is form. self is a parameter that we must shift so that we can access properties in the class and form parameters is the forms used in view.form.instance.user = self.request.user
: form.instance.user is used to get an instance of the form model, so we can access the user keyform.instance.use
and then fill in the value obtained from the user requestself.request.user
.And then we run the function
return super().form_valid(form)
.
Protected URL
We will create new functions that are important for URL protection systems. Of course, we will protect the URL. So that not everyone can access it. For more details, we can see the code below:
forums/views.py
from django.shortcuts import render
from django.views.generic.edit import CreateView
from .models import Forum
from django.contrib.auth.decorators import login_required // import function login_required
from django.utils.decorators import method_decorator // import utils method_decorator
@method_decorator(login_required, name='dispatch') //Protected the class
class ForumCreate(CreateView):
model = Forum
fields = ['title','desc']
def form_valid(self, form):
form.instance.user = self.request.user
return super().form_valid(form)
With this code, we will protect our class so that it cannot be accessed if we are not logged in.
from django.contrib.auth.decorators import login_required
for protection when the user is not logged in.from django.utils.decorators import method_decorator
then we have to import the module method_decorator.Now we have imported the decorator and login_required method, now we will use it like this
@method_decorator(login_required, name='dispatch')
. In @method_decorator there are two mandatory parameters. The first is the function that will be used login-required and the second is the type decorator name='dispatch'.
If there is no error then we cannot access the path 'forums/add', if the user is not logged in.
We can see in the picture above we have succeeded in creating a protection system on our URL.
- Create forum
Now we will add data with the user interface that we have created.
We can see in the picture above when we want to submit data, we get a warning. We get this not because of an error in our code. But because we don't define the routing that will be addressed after submitting the data. In Django there are two options for resolving this problem, namely Either provide a URL
or define a get_absolute_url
.
- Use
get_absolute_url
method
To solve the problem above we will use the method get_absolute_url
, Where we can use the get_absolute_url method ?. We can define models.py and in the forum class section, because the class we are accessing is forum class.
forums/models.py
from django.db import models
from django.conf import settings
from django.utils.text import slugify
from django.urls import reverse //import the reverse function
class Forum(models.Model):
user = models.ForeignKey(settings.AUTH_USER_MODEL, on_delete=models.CASCADE)
title = models.CharField(max_length=100)
slug = models.SlugField(unique=True)
desc = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
def save(self, *args, **kwargs):
self.slug = slugify(self.title)
super(Forum, self).save(*args, **kwargs)
def get_absolute_url(self): // define function get_absolute_url
return reverse('home') // redirect to home
class Comment(models.Model):
user = models.ForeignKey(settings.AUTH_USER_MODEL, on_delete=models.CASCADE)
forum = models.ForeignKey(Forum, on_delete=models.CASCADE)
desc = models.TextField()
created_at = models.DateTimeField(auto_now_add=True)
We can define the function in the forum class. We cannot change the name of the function. Because the
get_absolute_url()
function is the default function of Django.get_absolute_url()
is a function that will automatically run when the URL is successfully accessed.Redirect URL: We will run
get_absolute_url()
automatically but haven't done anything yet. For that according to the warning we have got. We need to create a redirect page when we successfully do POST data. To do the redirect we need help with thereverse ()
function. To use it we must import it as followsfrom django.urls import reverse
.reverse function ()
: We can pass a parameter that contains the Path from the URL or Alias. In this tutorial we will use an alias, after saving the data we will redirect to the homepage. We can give the alias as follows:
path(' ', TemplateView.as_view(template_name='welcome.html'), name='home')
I gave the alias the name of the path is 'home'
. If there is no error then we can see the results as follows
We can see in the picture above, we have successfully saved the data and implemented the get_absolute_url method.
Curriculum
- Class-based views
Tutorial Django - Class based view #2 : Use Class based view method and Get and Post method
- Forum app
Create a forum application using django #1 : Init projects and dependencies and Database schema
Create a forum application using django #2: Template system and Class-based view implementation
Create a forum application using django #3: Base template, Login and Register system
Create a forum application using django #4: Admin dashboard ang setting redirect
Thank you for your contribution.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @mcfarhat! Keep up the good work!
Congratulations! Your post has been selected as a daily Steemit truffle! It is listed on rank 18 of all contributions awarded today. You can find the TOP DAILY TRUFFLE PICKS HERE.
I upvoted your contribution because to my mind your post is at least 13 SBD worth and should receive 158 votes. It's now up to the lovely Steemit community to make this come true.
I am
TrufflePig
, an Artificial Intelligence Bot that helps minnows and content curators using Machine Learning. If you are curious how I select content, you can find an explanation here!Have a nice day and sincerely yours,
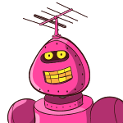
TrufflePig
Hi @duski.harahap!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @duski.harahap!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!