How To Calculate Your Engagement Rate Using php-steem-tools
You spent a lot of time networking on Steemit, commenting, upvoting and trying to create your own little community. At the end of the day, you got a few hundreds (of thousands) followers. Nice.
But how many of your readers are actually supporting you too? In other words, what's the engagement rate between the total number of your followers and the total votes you get on your posts?
In today's tutorial we're going to write a short snippet using php-steem-tools
and a little magic to get exactly that. I will describe the same approach used in the "Social Insights" section of steem.supply, which generates the following chart:
Prerequisites
You will need basic understanding of PHP and composer.
Step 1: Add php-steem-tools
to composer.php
Install composer (this is out of the scope of this tutorial, but if you need a good start, the Composer website has a very good documentation). Open composer.php and add the following lines:
{
"minimum-stability" : "dev",
"require": {
"dragos-roua/php-steem-tools": "v0.0.4-alpha"
}
}
The current version of the package is 0.0.4-alpha, and you need the "minimum-stability" setting to be at the "dev" level.
Step 2: Require the package and instantiate the Steem API
Create a file (in the same folder as composer.php) and require the package:
require __DIR__ . '/vendor/autoload.php';
Now you'll have access to all the classes you included in the composer.php file. Let's instantiate the API. Add the following lines:
$config['webservice_url'] = 'steemd.minnowsupportproject.org';
$api = new DragosRoua\PHPSteemTools\SteemApi($config);
Now, the $api
variable will contain a new API object which will query the data from the webservice_url
address. If you want to query a different node, just change this address.
Step 3: Pick an author and get the total number of the followers
If you read my previous php-steem-tools tutorial, you noticed that the first two steps are pretty much identical. Here's wehre we change things a bit.
We are going to find the total number of followers of the author. This is a recursive call, hence resource intensive, so we're going to use a little folder-based cache.
Here's the code:
$follower_count = $api->getFollowerCount($account);
$returned_follower_count = $follower_count['followers'];
$cache_folder = "./";
$file_name = $cache_folder.$author.".txt";
$cache_interval = 86400; // seconds for the cache file to live
if(file_exists($file_name)){
// if the file was modified less than $cache_interval, we do nothing
$current_time = time();
if($current_time - filemtime($file_name) > $cache_interval){
$follower_count = $api->getFollowerCount($author);
// write to the file again
$handle = fopen($file_name, 'w+') or die('Cannot open file: '.$file_name);
fwrite($handle, "followers|".$follower_count);
fclose($handle);
$returned_follower_count = $follower_count;
}
else {
// get the data from the cache file
$cache_contents = file($file_name);
$first_line = $cache_contents[0];
$content = explode("|", $first_line);
$returned_follower_count = $content[1];
}
}
else {
$follower_count = $api->getFollowerCount($author);
// write the data to cache file
$handle = fopen($file_name, 'w+') or die('Cannot open file: '.$file_name);
fwrite($handle, "followers|".$follower_count);
fclose($handle);
$returned_follower_count = $follower_count;
}
It's pretty much self-explanatory, not much going on here. We make a call to the getFollowerCount()
of the API and save the result in txt file. The variable $follower_count
will hold the returned value from the API call.
Step 4: Get the total number of posts and the votes
The total number of posts is not really required for our goal, but it's a nice addition. Again, if you looked at my previous tutorial, you'll notice some similarities.
What we are going to do is to call the getDiscussionsByAuthorBeforeDate($params, $transport)
function of the API, which will return the total number of posts during the last 8 days. If we have a result, we then cycle through the returned JSON object and the following:
- increment the value of the variable
$total_posts
- add to the array
$votes_number
the voter nickname as key, and total number of his votes as the value, because we are going to use the total number of users who are actually voting - at the end of the loop, we just print the calculated ratio
Here's the code:
date_default_timezone_set('UTC');
$dateNow = (new \DateTime())->format('Y-m-d\TH:i:s');
$date_8days_ago = date('Y-m-d\TH:i:s', strtotime('-8 days', strtotime($dateNow)));
$params = [$author, '', $date_8days_ago, 100];
$remote_content = $api->getDiscussionsByAuthorBeforeDate($params, 'websocket');
//print_r($remote_content);
if(isset($remote_content)){
if (array_key_exists('error', $remote_content)){
echo "Something is not ok. You should investigate more.\n";
}
else
{
$votes_number = array();
$total_posts = 0;
foreach($remote_content as $rkey => $rvalue){
if($rvalue['pending_payout_value'] !== '0.000 SBD' && $rvalue['max_accepted_payout'] != 0){
foreach($rvalue['active_votes'] as $idx => $voter){
$voter_nickname = $voter['voter'];
if($voter_nickname != $author){
if(array_key_exists($voter['voter'], $votes_number)){
$votes_number[$voter['voter']] += 1;
}
else {
$votes_number[$voter['voter']] = 1;
}
}
}
}
$total_posts++;
}
echo "\n**************\n";
echo "Total number of posts during the last 7 days: ".$total_posts."\n";
echo "Total number of voters: ".count($votes_number)." \n";
echo "Total followers: ".$returned_follower_count."\n";
echo "**************\n";
echo "Conversion rate: ".number_format((count($votes_number) * 100)/$returned_follower_count, 2)."%\n**************\n";
}
}
That's pretty much it!
Now you can invoke the script (in the same folder) by issuing this command: php conversionRate.php
.
And here's how it looks for the author utopian-io
:
utopian-io
has a 10.69% engagement rate.
You can get the full source of this tutorial from the 'examples' folder of the php-steem-tools repository on GitHub.
Note: this snippet is calculating the ratio between your followers and the number of votes you get, resulting in an overview of your popularity. It doesn't calculate how many of your followers are voting you. I leave this as a homework for you. Hint: there is a function in the api called getFollowers($account, $transport)
, which returns an array. You may want to intersect that array with the $votes_number
array created in the script above, and get the percentage of the voting followers.
I'm a serial entrepreneur, blogger and ultrarunner. You can find me mainly on my blog at Dragos Roua where I write about productivity, business, relationships and running. Here on Steemit you may stay updated by following me @dragosroua.
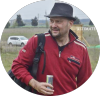
If you're new to Steemit, you may find these articles relevant (that's also part of my witness activity to support new members of the platform):
- Rewards: Were Does The Money Come From?
- Netiquette: How To Avoid Being A Steem Douche?
- Witnesses: What Are They And Why Should You Care?
- How Can I Mine Steem And What CPU / GPU Power Do I Need For That?
- The Beginner Guide To Not Getting Hacked On Steemit
Posted on Utopian.io - Rewarding Open Source Contributors
"Social Insights" is an amazing tool of steem.supply ...Thanks @dragosroua for sharing these programming details....
very useful tool for steemit, thanks for sharing
Very useful, but I use minnowbooster sometimes for an upvote and because they use other peoples account to vote it is difficult to see whats a real vote or a paid one.
@dragosroua I love when you bring this to the table and show us this Great Stuff..........
That's pretty neat. Can come in handy, gonna check it out asap,
Thank you so much for this tool. i love the features of steem
super decent code man. a debt of gratitude is in order for including this element.Upvoted and resteemed
It's very much important to know one's daily engagement. However, it's more important to know which chunk of the audience is proactively participating in social media so that you could device your next SM strategy accordingly. Having that being said, php-steem-tools is very good tool one should have for Steemit. Also, this seems to be the first one on Steem engagement, if I'm not wrong.
Thanks @dragosroua. Definitely going to share with my audience and I hope they love it.
Steem On!
Nice! I suspect 10% is actually pretty strong given the dead followers.
Yes, anything over 10% is very good, especially if the number of followers is beyond a few thousands.
you always focus our attention on simple things that have a big impact..we always fail to notice them unless someone like you brings it for the discussion :)