My first roguelike development blog - [game programming blog ~ part 2]
My roguelike development blog ~ part 2
In my first tutorial I showed you how to write a main function for your game. This main function is the program main loop where all the action is called. So how do we define and call our own functions inside our program?
First we need to create a new file (our source code) to store our function definitions in. Select File on the drop down menu near the top of your program, then press and expand New and choose source file. You will be prompted if you want to create a new file, so click 'yes'. Save this file into your project by pressing ctrl + s and give your header definition file a name.
For simplicity lets call it GameManager.h
You can also add a new file by right-clicking your custom folder (or project root) in your Project viewer and selecting New file.
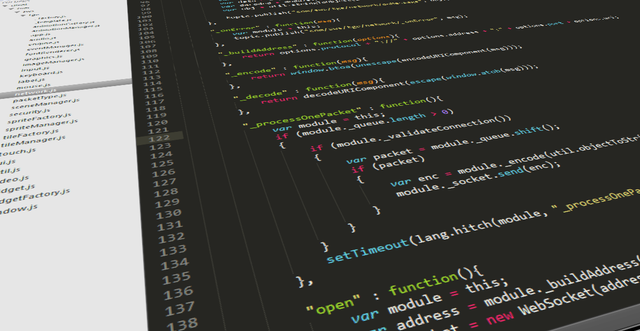
To re-select our main.cpp press the pane called main.cpp on the filepane near the top of your IDE.
Creating our own functions
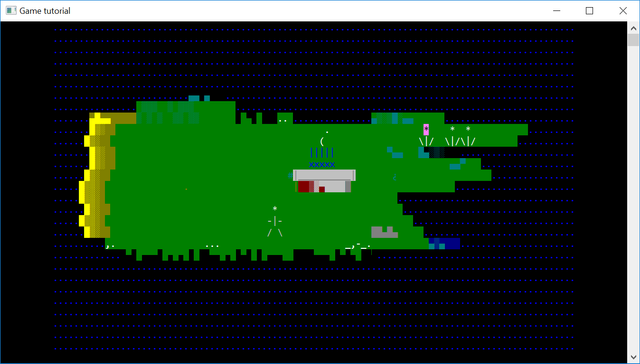
The first thing we need to do is to make sure our custom files and functions are included into our executable so they can be called inside our program.
To avaid multiple inclusions of a source code library we tell our program to include a file once by writing an inclusion guard.
Start by navigating back to your newly created file. Sometimes new files contain prewritten mockup code. We don't need this, so delete all the code in your file and, at the top of GameManager.h, write:
#ifndef GAMEMANAGER_H
#define GAMEMANAGER_H
#endif
Now we begin the process of writing our own custom defined functions. Our current file, the GameManager.h header file, is a function definiton template. This is where we predeclare any function we write so the compiler knows to look for a function implementation later on.
Place your mouse cursor between the define GAMEMANAGER_H and endif. Write the following code:
#include <iostream>
using namespace std;
class GameManager {
public:
private:
};
This is our custom class function declaration. The code private and public is the class' access specifier which restricts private functions to be called anywhere but inside this particular class.
You also need to include any libraries you are using in this class, like we did in our first tutorial.
It's time to start writing our custom functions. We need to declare an object of a class implementation in order to use it in our program. When creating a new object the compiler begin by looking for a constructor. A constructor is member function of a class that is executed whenever we create our object.
A constructor has to be called the same thing as the header file. Be aware that the constructor name is type sensitive, meaning GameManager.hs constructor needs to be called:
GameManager();
Our constructor usually is placed at the very top of our implementation, so our code should now look like this:
#ifndef GAMEMANAGER_H
#define GAMEMANAGER_H
#include <iostream>
using namespace std;
class GameManager {
public:
GameManager();
private:
}; // END GameManager
#endif
Implementing our custom function
We can now start defining our custom functions. The first thing the compiler looks for is the functions return type. This is one of C++ predeclared primitive data types that make up our program. Our new function will be called Program() and have a return type of type integer. The parentheses after Program is the functions parameter list. I will demonstrate how to use that in a later tutorial.
To create our first function, add a new line after the constructor by pressing enter, indent the next line by pressing tab and write:
int Program();
For the sake of keeping the scope of this tutorial easy I will not show you how to create private data variables. Lets instead focus on creating our custom function called Program();
To implement a function definition we are going to need another file, so create a new file and call it GameManager.cpp. I prefer to save my header file declerations in a folder called header and source code implementations in a folder called source.
Once you have createed a new file we start by implementing our custom class object of function(s). As always we begin by including the libraries we are using in our program, but in our class implementation we only need to include the header file where all our included libraries are defined. To include a header file into a source implementation you use the codeword include and tell the compiler where the header file is located. GameManager.cpps decleration is stored in a folder called header, so we direct the compiler to the folder and include it like this:
#include "../header/GameManager.h"
To implement the constructor, now easly found by the compiler, we have to put it inside a scope. This is done by calling the class' namespace (GameManager) and initializing a scope resolution operator.
If you don't explicitly write a constructor C++ defines a default constructor for you, so lets jump to implementing our custom function. Remember to declare the functions return type, in this case int. Again we have to declare the class scope, GameManager, and implement our function and its parameter list. Our GameManager.cpp will now look like:
#include "../header/GameManager.h"
GameManager::GameManager () {
// Deliberately left blank!
} // END ctor
int GameManager::Program () {
} // END Program
The // and /**/ are comments. The compiler will not parse comments into the program at compile time, so use of this to make notes inside your program.
To test our new function we add a printout statement inside our Program() function. Here is what it would look like:
cout << "\nThis is printed from our Program()-function!";
Using the function in our program
If we want to use our now class library implementation in our program, we have to include it like any other library file. Navigate back to main.cpp and include GameManager.h at the top, right before or right after the other includes.
Including GameManager.h should like look:
#include "header/GameManager.h"
Notice how the include refernece doesn't begin with two [..]? This is because your main.cpp should be stored in the root directory of your project folder, and therefore only need to navigate to the folder called header where the header file is stored. Another difference between standard library inclution and custom header inclution is that our custom include are wrapped in ""-tags.
Finally we can declare an object of our custom class by calling the global name (GameManager) and instanciating an object by creating an object call name. Creating an object is done with the following decleration code:
GameManager gm;
To test our newly created Program() function, call it with:
gm.Program();
Compile and run your program and you shall see the sentence "Game programming tutorial" and "This is printed from our Program()-function!" printed to your console terminal.
That's it! Now you know how to create your own functions and calling them in your program. Next time I will expand this example and show you how to create a simple menu for your program.
To see more - upvote this post and follow me @jonrhythmic
Really enjoying reading these.
When you talk about the tags for comments, you point out that /**/ can be used, but without an example, some new programmers may think it is used in the same way as //. An example showing that it is a start and end tag to wrap a longer comment would clarify this for newcomers.
My other comment is on referencing your header file. When I was taught, we were always told to use one of the two leading pointers to clarify where they file should be. So when you're starting in the same directory as the file you're putting the #include into, you use "./header/GameManager.h ". It may just be a style thing, but it seemed a bit odd to see it without a leading indicator for me.
@ratticus Thanks for reading and commenting! Appreciate it.
I'll leave you comments on commenting here, so people reading this can get this tips straight from the source. I was not aware that you could, or should, start a custom #include with "./...". Thank you for pointing this out.
Nice post. Very informative and useful to the technical reader. Really helpful. Good work and good content. Will continue to follow.
@steppingout23 Thanks a lot! Will try to write another edition shortly.
nice post @jonrhythmic. thanks for sharing. this is a beautiful read
@outhori5ed Thanks a million :)
This is great! you have inspired me of doing the same!
@favio Thank you. Great to have inspired something creative! Hope you come through!