Using Steem-API with Ruby Part 15 — Print Steem Engine Token values
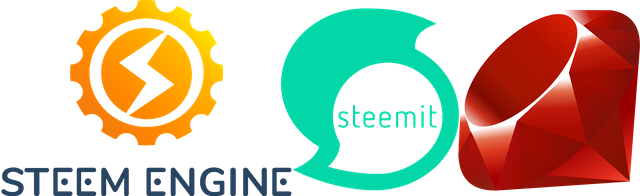
Repositories
SteemRubyTutorial
All examples from this tutorial can be found as fully functional scripts on GitHub:
- SteemRubyTutorial
- radiator sample code: Steem-Print-SCC-Metrics.rb.
radiator
- Project Name: Radiator
- Repository: https://github.com/inertia186/radiator
- Official Documentation: https://www.rubydoc.info/gems/radiator
- Official Tutorial: https://developers.steem.io/tutorials-ruby/getting_started
Steem Engine

- Project Name: Steem Engine
- Home Page: https://steem-engine.com
- Repository: https://github.com/harpagon210/steem-engine
- Official Documentation: https://github.com/harpagon210/sscjs (JavaScript only)
- Official Tutorial: N/A
What Will I Learn?
This tutorial shows how to interact with the Steem blockchain, Steem database and Steem Engine using Ruby. When accessing Steem Engine using Ruby their only one APIs available to chose: radiator.
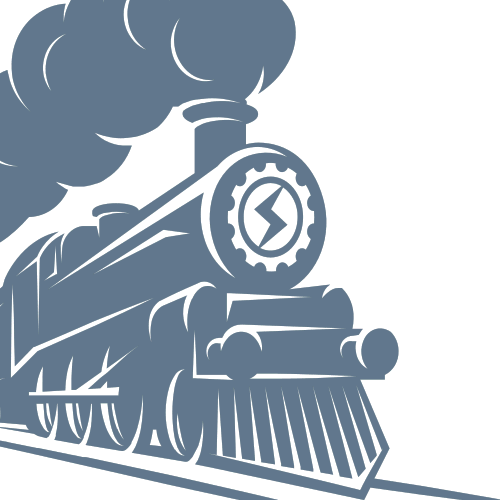
In this particular chapter you learn how to read the current exchange rates for steem engine token.
Requirements
Basic knowledge of Ruby programming is needed. It is necessary to install at least Ruby 2.5 as well as the following ruby gems:
gem install bundler
gem install colorize
gem install contracts
gem install radiator
Difficulty
For reader with programming experience this tutorial is basic level.
Tutorial Contents
Implementation using radiator
SCC/Steem_Engine.rb
Base class for all steem engine classes. This class holds general constants and a lazy initialization to the contracts API.
module SCC
class Steem_Engine < Radiator::Type::Serializer
include Contracts::Core
include Contracts::Builtin
attr_reader :key, :value
Amount of rows read from database in a single query. If the overall results exceeds this limit then make multiple queries. 1000 seem to be the standard for Steem queries.
QUERY_LIMIT = 1000
To query all rows of an table an empty query and not a nil
must be provided.
QUERY_ALL = {}
Provide an instance to access the Steem Engine API. The attribute is lazy initialized when first used and the script will abort when this fails. Note that this is only ok for simple scripts. If you write a complex web app you spould implement a more elaborate error handler.
class << self
attr_reader :QUERY_ALL, :QUERY_LIMIT
@api = nil
##
# Access to contracts interface
#
# @return [Radiator::SSC::Contracts]
# the contracts API.
#
Contract None => Radiator::SSC::Contracts
def contracts_api
if @api == nil then
@api = Radiator::SSC::Contracts.new
end
return @api
rescue => error
# I am using Kernel::abort so the code snipped
# including error handler can be copy pasted into other
# scripts
Kernel::abort("Error creating contracts API :\n".red + error.to_s)
end #contracts
end # self
end # Token
end # SCC
Hint: Follow this link to Github for the complete script with comments and syntax highlighting : SCC/Steem_Engine.rb.
SCC/Metric.rb
Most of the actual functionality is part of a new SCC:Metric
class so it can be reused in further parts of the tutorial.
require 'pp'
require 'colorize'
require 'contracts'
require 'radiator'
require 'json'
require_relative 'Steem_Engine'
module SCC
##
# Holds the metrics of Steem Engine Token that is the
# recent prices a steem engine token was traded.
#
class Metric < SCC::Steem_Engine
include Contracts::Core
include Contracts::Builtin
attr_reader :key, :value,
:symbol,
:volume,
:volume_expiration,
:last_price,
:lowestAsk,
:highest_bid,
:last_day_price,
:last_day_price_expiration,
:price_change_steem,
:price_change_percent,
:loki
public
The class contructor create instance form Steem Engine JSON object. Numeric data in strings are converted floating point values.
Contract Any => nil
def initialize(metric)
super(:symbol, metric.symbol)
@symbol = metric.symbol
@volume = metric.volume.to_f
@volume_expiration = metric.volumeExpiration
@last_price = metric.lastPrice.to_f
@lowest_ask = metric.lowestAsk.to_f
@highest_bid = metric.highestBid.to_f
@last_day_price = metric.lastDayPrice.to_f
@last_day_price_expiration = metric.lastDayPriceExpiration
@price_change_steem = metric.priceChangeSteem.to_f
@price_change_percent = metric.priceChangePercent
@loki = metric["$loki"]
return
end
Create a colorized string showing the current STEEMP value of the token. STEEMP us a steem engie token that can be exchanged 1:1, minus a 1% transaction fee, to STEEM. It's STEEMP which gives the token on Steem Engine it's value.
The current highest bid is printed green, the current lowest asking price us printed in red and the last price of an actual transaction is printed on blue.
Unless the value is close to 0, then the value is greyed out. Reminder: Never use equality for floating point values to 0. Always use some ε value.
Contract None => String
def to_ansi_s
begin
_retval = (
"%1$-12s" +
" | " +
"%2$18.6f STEEM".colorize(
if @highest_bid > 0.000001 then
:green
else
:white
end
) +
" | " +
"%3$18.6f STEEM".colorize(
if @last_price > 0.000001 then
:blue
else
:white
end
) +
" | " +
"%4$18.6f STEEM".colorize(
if @lowest_ask > 0.000001 then
:red
else
:white
end) +
" | "
) % [
@symbol,
@highest_bid,
@last_price,
@lowest_ask
]
rescue
_retval = ""
end
return _retval
end
Read the metric of a single token form the Steem Engine database and convert it into a SCC::Metric
instance. The method Steem_Engine.contracts_api.find_one
method is described in Part 13.
class << self
##
#
# @param [String] name
# name of symbol
# @return [Array<SCC::Metric>]
# metric found
#
Contract String => Or[SCC::Metric, nil]
def symbol (name)
_metric = Steem_Engine.contracts_api.find_one(
contract: "market",
table: "metrics",
query: {
"symbol": name
})
raise KeyError, "Symbol «" + name + "» not found" if _metric == nil
return SCC::Metric.new _metric
end
Read the metrics of all tokens form the Steem Engine database and convert it into a SCC::Metric
instance. The method Steem_Engine.contracts_api.find_one
method is described in Part 14.
##
# Get all token
#
# @return [SCC::Metric]
# metric found
#
Contract String => ArrayOf[SCC::Metric]
def all
_retval = []
_current = 0
loop do
_metric = Steem_Engine.contracts_api.find(
contract: "market",
table: "metrics",
query: Steem_Engine::QUERY_ALL,
limit: Steem_Engine::QUERY_LIMIT,
offset: _current,
descending: false)
# Exit loop when no result set is returned.
#
break if (not _metric) || (_metric.length == 0)
_retval += _metric.map do |_token|
SCC::Metric.new _token
end
# Move current by the actual amount of rows returned
#
_current = _current + _metric.length
end
return _retval
end # all
end # self
end # Metric
end # SCC
Hint: Follow this link to Github for the complete script with comments and syntax highlighting : SCC/Metric.rb.
Steem-Print-SCC-Metrics.rb
With all the functionality in classes the actual implementation is just a few lines:
require_relative 'SCC/Metric'
_metrics = SCC::Metric.all
puts("Token | highes Bid | last price | lowest asking |")
puts("-------------+--------------------------+--------------------------+--------------------------+")
_metrics.each do |_metric|
puts _metric.to_ansi_s
end
Hint: Follow this link to Github for the complete script with comments and syntax highlighting : Steem-Print-SCC-Metrics.rb.
The output of the command looks like this:
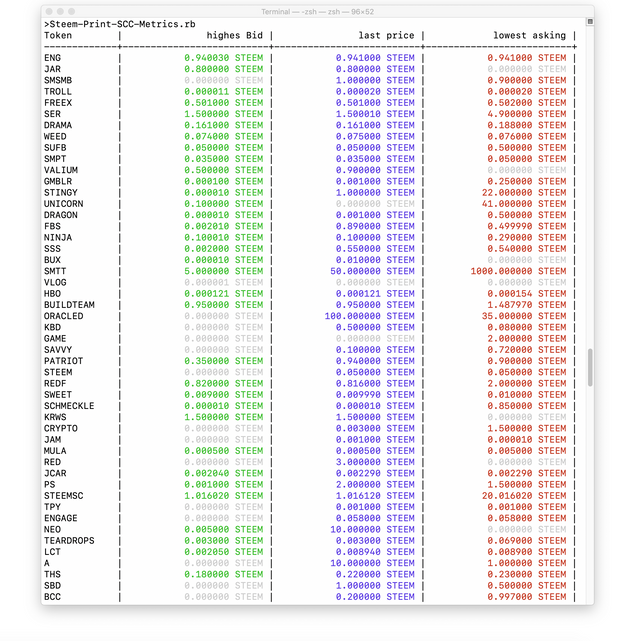
Curriculum
First tutorial
Previous tutorial
Next tutorial
Proof of Work
- GitHub: SteemRubyTutorial Issue #17
Image Source
- Ruby symbol: Wikimedia, CC BY-SA 2.5.
- Steemit logo Wikimedia, CC BY-SA 4.0.
- Steem Engine logo Steem Engine
- Screenshots: @krischik, CC BY-NC-SA 4.0
Beneficiary
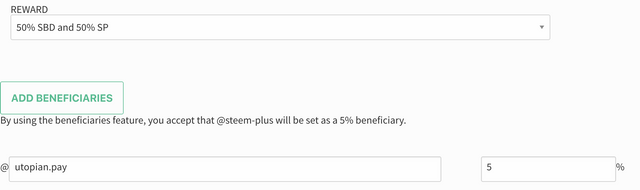







Thank you for your contribution @krischik.
After analyzing your tutorial we suggest the following points below:
Your tutorial is well organized and explains very well all the concepts and development.
It would be interesting at the end of your tutorial to have the result visible in GIF.
Thank you for following our suggestions in your previous tutorial.
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin! Keep up the good work!
Hi @krischik!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hey, @krischik!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!