Using Steem-API with Ruby Part 11 — Using Mechanize to improve performance
Using Steem-API with Ruby Part 11
Repositories
SteemRubyTutorial
All examples from this tutorial can be found as fully functional scripts on GitHub:
- SteemRubyTutorial
- steem-mechanize sample code: Steem-Dump-Vesting.rb
steem-mechanize
- Project Name: Steem Mechanize
- Repository: https://github.com/steemit/steem-mechanize
- Official Documentation: https://www.rubydoc.info/gems/steem-mechanize
- Official Tutorial: N/A
What Will I Learn?
This tutorial shows how to interact with the Steem blockchain and Steem database using Ruby. When using Ruby you have three APIs available to chose: steem-api, steem-mechanize and radiator which differentiates in how return values and errors are handled:
- steem-api uses closures and exceptions and provides low level computer readable data.
- steem-mechanize drop in replacement for steem-api with more performat network I/O
- radiator uses classic function return values and provides high level human readable data.
This tutorial takes a special look at the steem-mechanize API.
Requirements
Basic knowledge of Ruby programming is needed. It is necessary to install at least Ruby 2.5 as well as the following ruby gems:
gem install bundler
gem install colorize
gem install contracts
gem install steem-mechanize
Note: All APIs steem-ruby, steem-mechanize and radiator provide a file called steem.rb
. This means that:
- When more then one APIs is installed ruby must be told which one to use.
- The three APIs can't be used in the same script.
If there is anything not clear you can ask in the comments.
Difficulty
For reader with programming experience this tutorial is basic level.
Tutorial Contents
Printing all delegation from and to a list of accounts, as shown in the last tutorial, hast proven to be a time consuming operation — easily lasting over an hour.
On way to improve performance is to skip over the delegations of the “steem” account who delegates to most accounts below a steem power of ≈30000 VEST / 15 STEEM. This brings the run time down to about half an hour. Which is still a lot.
Another way to improve performance is the use of steem-mechanize a combination of steem-ruby and mechanize. Mechanize improved performance by using Persistent HTTP connections.
Implementation using steem-mechanize
steem-mechanize is a drop in replacement for steem-ruby and theoretically the only change to the code needed is the use of require 'steem-mechanize'
instead require 'steem-ruby'
.
# use the "steem.rb" file from the steem-ruby gem. This is
# only needed if you have both steem-api and radiator
# installed.
gem "steem-ruby", :require => "steem"
require 'pp'
require 'colorize'
require 'steem-mechanize'
# The Amount class is used in most Scripts so it was moved
# into a separate file.
require_relative 'Steem/Amount'
However in praxis there is one more Problem: steem-mechanize is to fast for https://api.steemit.com and you will be get an “Error 403” / “HTTP Forbidden” if you try.
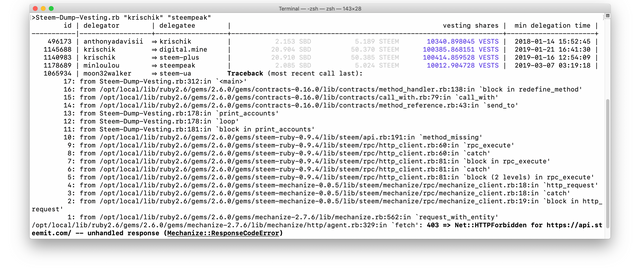
This suggest that Steemit has an upper limit on how many the accesses per seconds it allows which makes it necessary to add additional error handling and a little sleep
. To avoid duplication only the differences to the original version is shown.
At the beginning of the file a constant indicating the maximum retries to be done.
##
# Maximum retries to be done when a
#
Max_Retry_Count = 3
At the beginning of the main loop a retry counter is added.
# empty strings denotes start of list
_previous_end = ["", ""]
# counter keep track of the amount of retries left
_retry_count = Max_Retry_Count
loop do
At then end of the loop a sleep
is added to throttle the loop to 20 http requests per second. Test have shown that this is usually enough to make the loop run though.
For the few cases where throttling is not sufficient an error handler is added to allow up to three (Max_Retry_Count
) retries.
# Throttle to 20 http requests per second. That
# seem to be the acceptable upper limit for
# https://api.steemit.com
sleep 0.05
# resets the counter that keeps track of the
# retries.
_retry_count = Max_Retry_Count
rescue => error
if _retry_count == 0 then
# We made Max_Retry_Count repeats ⇒ giving up.
print Delete_Line
Kernel::abort ("\nCould not read %1$s with %2$d retrys :\n%3$s".red) % [_previous_end, Max_Retry_Count, error.to_s]
end
# wait one second before making the next retry
_retry_count = _retry_count - 1
sleep 1.0
end
Hint: Follow this link to Github for the complete script with comments and syntax highlighting: Steem-Dump-Vesting.rb.
The output of the command (for my own and the steem account) looks just like before:
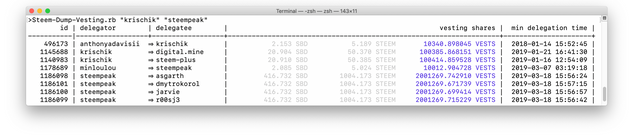
However it steem-mechanize version is just about 2½ faster:
API used | Time elapsed |
---|---|
steem-ruby | 27'58" |
steem-mechanize | 11'49" |
Curriculum
First tutorial
Previous tutorial
Next tutorial
Proof of Work
- GitHub: SteemRubyTutorial Issue #13
Image Source
- Ruby symbol: Wikimedia, CC BY-SA 2.5.
- Steemit logo Wikimedia, CC BY-SA 4.0.
- Screenshots: @krischik, CC BY-NC-SA 4.0
Beneficiary
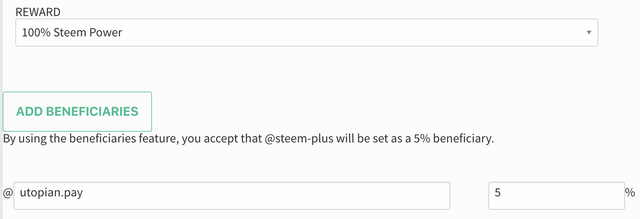







Thank you for your contribution @krischik.
After analyzing your tutorial we suggest the following points below:
Excellent tutorial as it has always presented. The contribution is easy to read and very pleasant. The structure of the tutorial is fine. Good job!
In the next tutorial put more images of the results of what you are explaining.
Thank you for your work in developing this tutorial.
Looking forward to your upcoming tutorials.
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin! Keep up the good work!
Hi @krischik!
Your post was upvoted by @steem-ua, new Steem dApp, using UserAuthority for algorithmic post curation!
Your post is eligible for our upvote, thanks to our collaboration with @utopian-io!
Feel free to join our @steem-ua Discord server
Hi, @krischik!
You just got a 5.13% upvote from SteemPlus!
To get higher upvotes, earn more SteemPlus Points (SPP). On your Steemit wallet, check your SPP balance and click on "How to earn SPP?" to find out all the ways to earn.
If you're not using SteemPlus yet, please check our last posts in here to see the many ways in which SteemPlus can improve your Steem experience on Steemit and Busy.
Hey, @krischik!
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Get higher incentives and support Utopian.io!
Simply set @utopian.pay as a 5% (or higher) payout beneficiary on your contribution post (via SteemPlus or Steeditor).
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Congratulations @krischik! You have completed the following achievement on the Steem blockchain and have been rewarded with new badge(s) :
You can view your badges on your Steem Board and compare to others on the Steem Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP