Java Programming for Beginners from Development Kit Installation to Exceptions
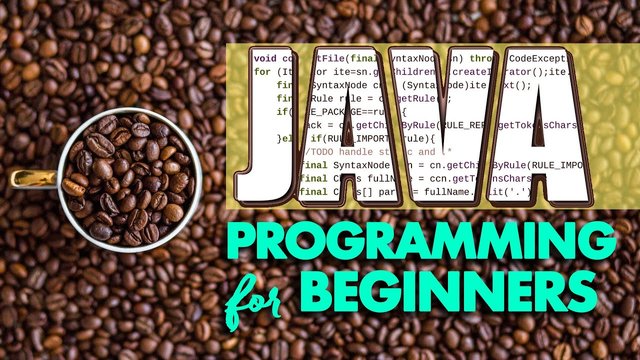
Hello and welcome to this new Java programming language course. In this course, we will learn all about the Java programming language.
If you will enjoy reading and contributing to the discussion for this post, will you please join us on the YouTube video above and leave a comment there because I read and respond to most comments on YouTube?
If you find anything helpful in this video or funny, will you please leave a like because you will feel great helping other people find it?
To start with the course, you would first have to be able to compile Java code, and to have a text editor. You have to install the Java JDK, so the Java Development Kit. It’s really easy. It depends on the operating system you’re using. You go to Google and search for download of the JDK and then you will select your operating system whether it is Windows, Mac OS, Linux or whatever. With Java it doesn’t really matter in which operating system you’re using it because it runs in a virtual machine. It’s the same for every operating system. Once you have installed it in this case, I will be using Visual Studio Code in a Linux operating system.
I’m using it on Debian but it will work the same for Ubuntu or whatever other Linux distribution. This is Visual Studio. This is a free code editor for almost any platform out there. You can also download it in Windows, Mac OS, or Linux.
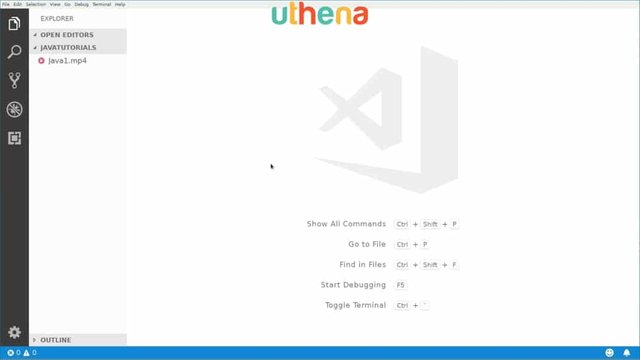
After you’ve installed the Java Development Kit, you should be able to open a terminal and type java and if you type enter, these options should appear.
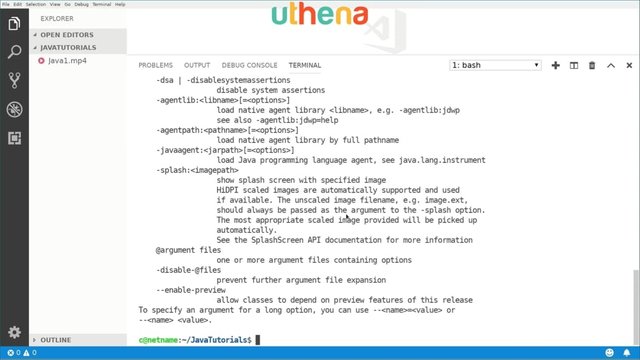
If you type in javac which is the Java compiler these options should appear.
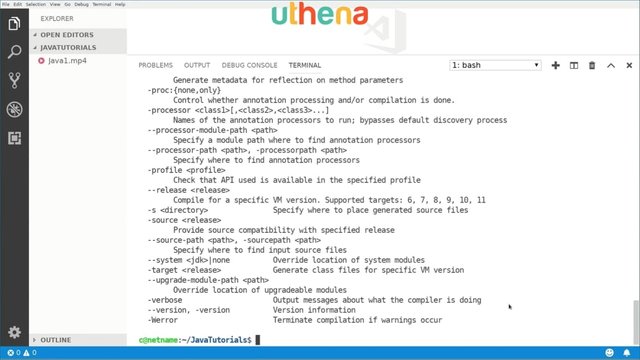
Before moving forward with the tutorial make sure you have Java and Java C installed.
Java is a high-level object oriented programming language that runs on a virtual machine. This means you don’t need to recompile your code if you run it on a different architecture.
Java basics are kind of about objects. Objects are kind of pieces of code that have certain states and behaviors like a person has a name, and has the weight and has something. Characteristics of an object.
We also have classes which is a template that describes the behavior of the object and the type of it.
We also have methods that are procedures that we can call of the behavior of an object .A class can contain many methods.
We also have instance variables. Each object has its own set of instance variables and object state is created by the values assigned to these variables.
We will start coding right now. We will open a visual studio code and we will open a new folder. In this case my folder here is JAVA TUTORIALS. If you don’t know how to open a new folder you simply go to file, and here you go to open folder, and you basically create a new folder. I have created this JAVA TUTORIALS folder here.
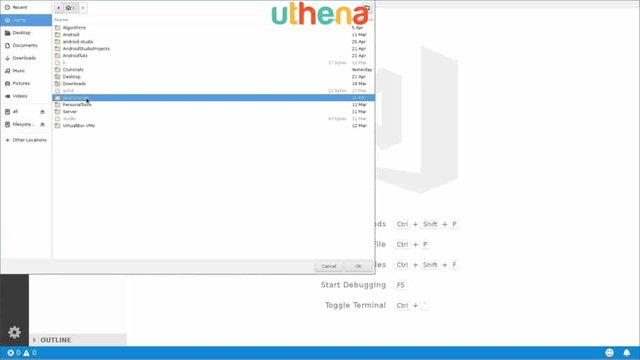
If I open it you can maybe create another one. Maybe I will create one that is Javacode.class. I will press ENTER and here we can see that our first source code file was created. First of all, every Java source code has to start with a class. You can start by typing public class JavaCode. I will open brackets. Once I have opened the brackets I can write the remaining stuff.
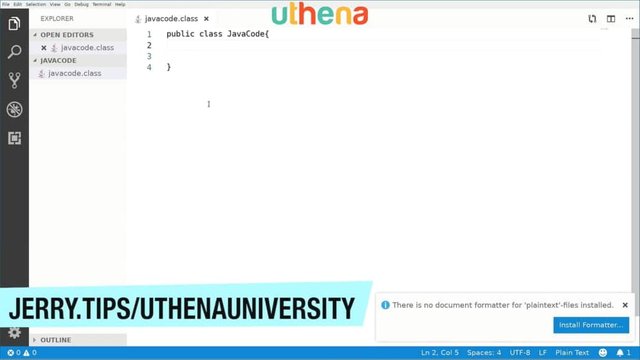
Inside the class, we can write the method and in this case, since we’re using pure Java, we want to write the main method. You’re going to say public static void main (String[] args} which are the arguments that might be passed to our program.
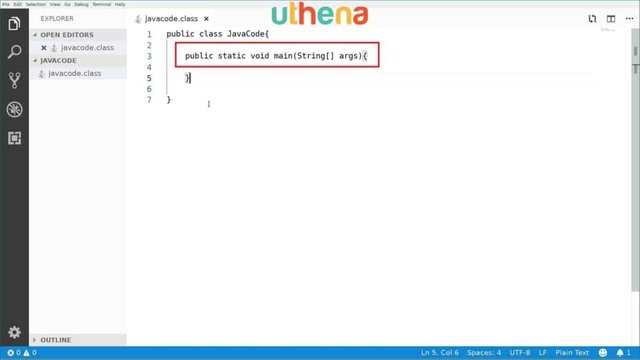
That’s kind of the starting point for us to learn how we can structure a Java program. We have a class here and inside the class we have the main method.
Please write the main method as I’m doing and now we’re going to print something on the screen.
To print something you’re going to say System.out.println(“Hello world”};. This will be our Hello World program. We have to end this line with a semicolon.
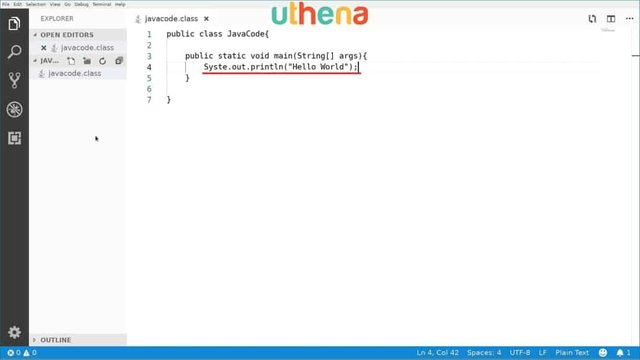
Our source code file is ready. For your convenience, if you don’t have the autosave feature enabled, you can enable it by going to file, and then click here to autosave. You won’t have to be saving every time you change or you make changes to your source code file.
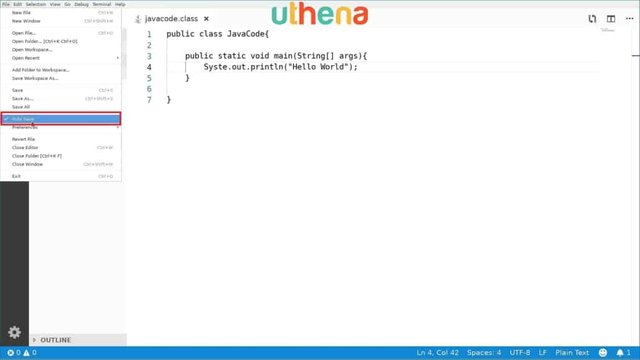
Now that the file is saved, we can compile this program. To open the terminal in Visual Studio code, we’ll press ctrl + the key that is just below the Escape key and TERMINAL, as you can see, will be opened. Here what we will write will be the following. We’ll write javac Javacode.class and after we’ve done this there’s an error, so maybe we don’t have to use the .class suffix here. We will change the name of the file to .java. I was confused about this previously.
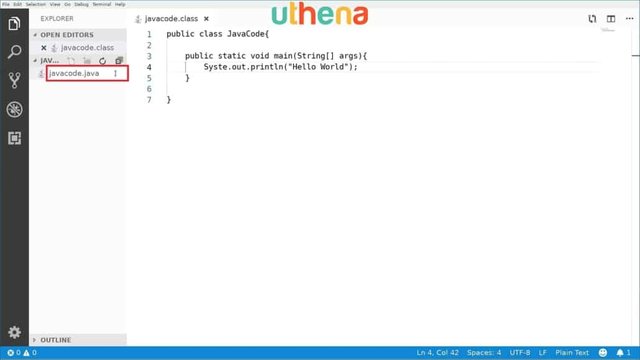
It’s Javacode.java so we will rename our file here, and we will say javac Javacode.java. After we’ve done this it still has some syntax errors here. You know what? I think the problem here is that the name of the class should be the same name of this inside class.
This starts with an uppercase J. We also have to use that uppercase J in the name of the file so let me define this to uppercase J and now we will run it again and apparently we still have a problem.
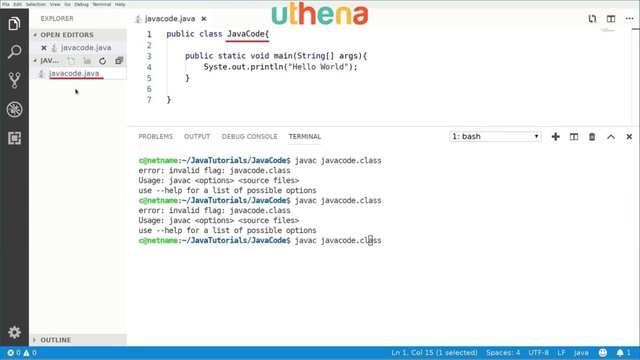
Let me see. If we press javac. I’m not using an uppercase here so we’ll use uppercase here and it’s saying invalid flag: Javacode.class but it’s not that class. It’s Javacode.java. Once we’ve done this we have an error here which is a typo error.
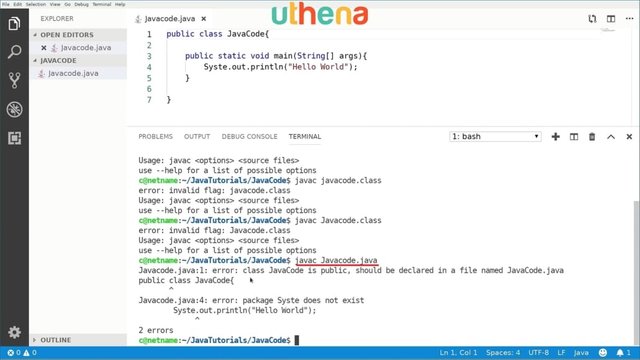
Instead of a system, I forgot to put an m here.
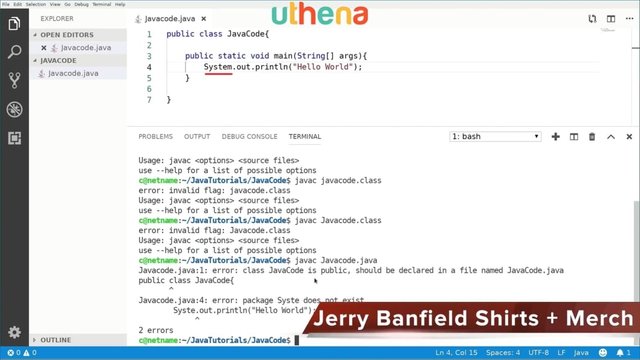
Let’s try it again and now we still have an error here. It says Javacode is public and should be declared in a file called Javacode.
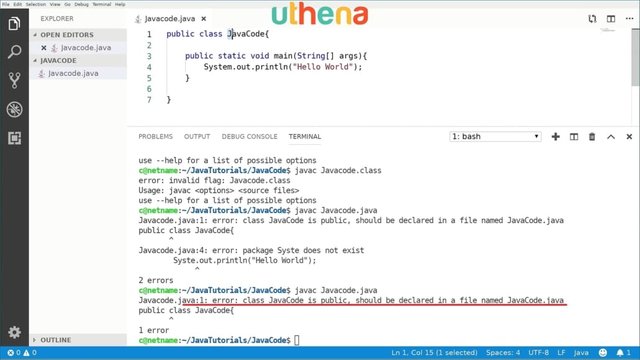
This is pretty important. Right here as the name of our class you should have the same exact name of your file. If it’s just the J’s the only uppercase letter then it should be the only uppercase letter and the code wants the uppercase.
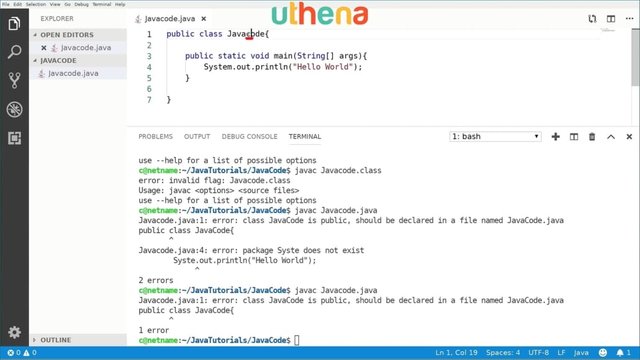
If we run it, it will finally be compiled after lots of errors.
Again, to clarify this, we use Javac because this is called the Java compiler. For the file name, we have to have the same file name as we have in the class name.
Again, Javac Javacode.java. We pressed enter and the program is now being compiled.
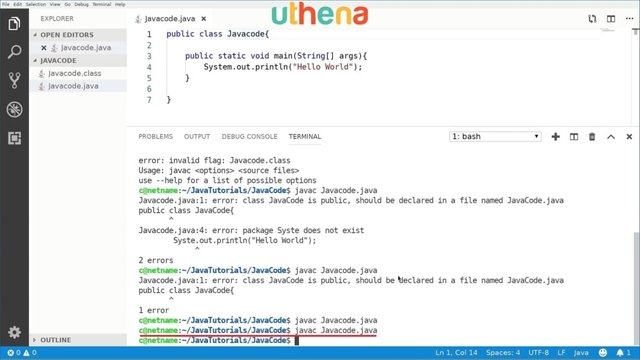
How Do We Run That Program?
We’ll to run this program, we will just say java Javacode. Notice that here I’m not using javac anymore I’m just using java and then just saying Javacode.
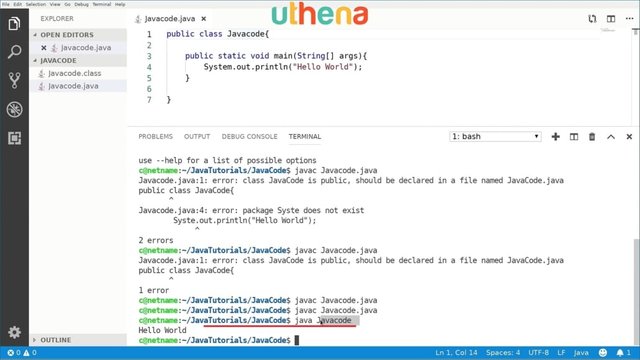
Why not .java? Because the .java is the source code file and this is the executable file. If we see which files we have we can see that we have the Javacode.class is equivalent to just Javacode and we have Javacode.java.
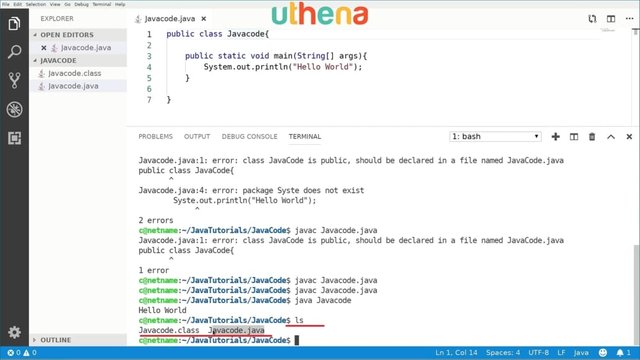
We have those two files and now this is how you create a simple hello world program for Java. As I told you Java is case-sensitive. You have to be aware of the cases so to avoid the errors I just made previously.
That’s how a simple, simple Java program works.
Let’s Go Back To The Java Source Code To Learn A Bit More About It.
The first word we have is public.
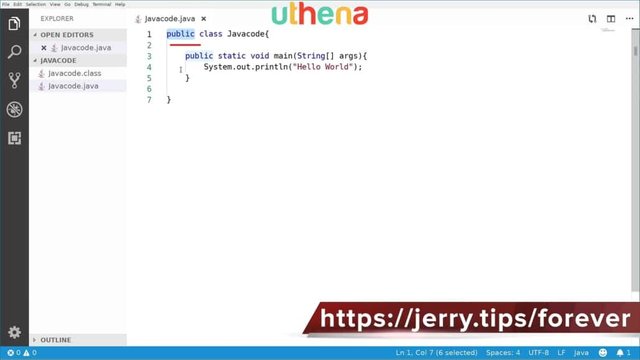
What does public mean?
Public is a kind of identifier that enables us to know how accessible an element is. It can be public, private, and protected. What does it mean? If it’s public it means it can be accessed from the outside world. If it is private it means it can only be accessed from inside the class - from the inside world - if you want it that way. If it’s protected it can be accessed from inside the package. Imagine a class is your house and protected means that it can be accessed by the other houses in your block.
Public means that it can be accessed by anyone in the world. Privately it means that it can only be accessed by you or by another house or family members. This enables us to restrict access or to allow access to different instances of a program. We are able to have local variables and arrays, and all kinds of different data types that we have in another programming language.
We have this public static void which means this is a method that returns void which means doesn’t return anything. Absence of type basically means void. Static means that it exists only kind of statically in memory.
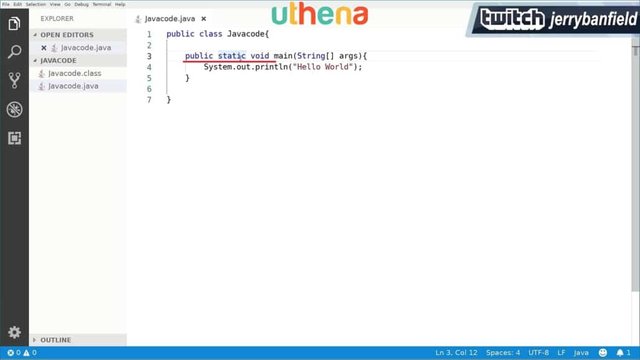
This means if we have a static variable we can keep the value of the variable between function calls. We can also write comments in Java. To write comments we start with // and we will say //Hello I am a comment.
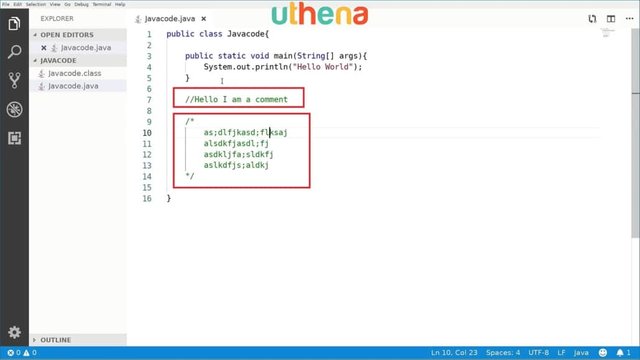
This is basically how we write comments and we also have multiple comments. If we want to write more than one line comments we say / * */ and inside these two kind of blocks we can write whatever we want in multi lines /* as;dl fjkasd;flksaj alsdkfjasdk’fj*/. Lots of comments.
We have a single line of comments and we have multi-line comment.
Now, we also know how to print out to the screen which uses the System.out.println which is a method that it’s available to us as part of the Java language in order to enable us to print to the screen.
What Are Objects?
Well, like in the real world we have different objects like tables, chairs, cars, whatever the case may be.
For example, if we consider an animal like a cat, we have that the state can be maybe, it can have a name, it can have a size, it can be of a certain color, it has some behavior, like making cat noises or liking to play with anything basically. An object defines how that object behaves and the characteristics of it.
Java is an object oriented programming language. If we want to define a dog, for example, how can we do it?
First we can create an inside class inside this to define a dog or we can also create something outside this class. If I create something outside this class just after the class bracket, I can say public class Dog {}.
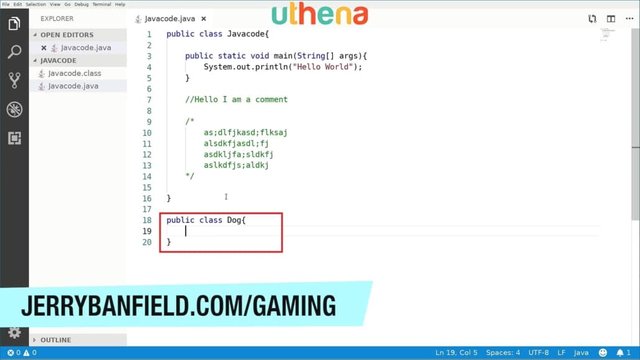
What I just did is create a dog object that will enable me to define it as a different sort of behavior, and characteristics, and the features that the dog has. For example, inside the class I would say String breed;.
String is basically a data type that enables us to start a sequence of characters like a sentence or word or a paragraph. This enables us to store text. That’s a string.
We also have int.
What Is An Int?
Well, an int is an integer value which enables us to store numbers so maybe the int will be the age.
I will say int age; and maybe we want to also define the color of the dog. Let’s say String color;. We can define maybe the weight using another int but if we want to be more precise and instead of using integer values we want to use floating-point values, we can say float, and defined a dog’s weight. So, float weight; maybe in kilograms or pounds or whatever the case may be.
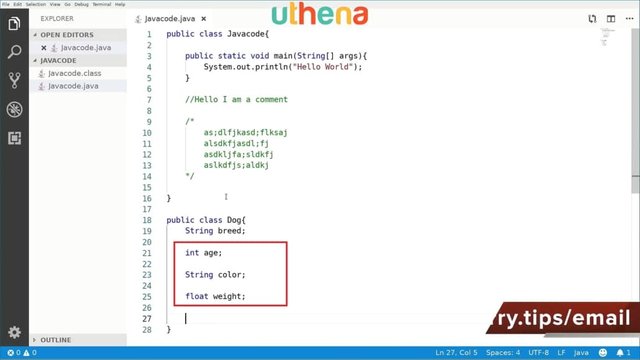
This is how we define a dog element. We also can define some behaviors of a dog, for example, barking. I’m going to create void barking {} and now, we have a new behavior that the dog is able to bark. That’s basically how we define a dog. You can extend this stack class by adding more features and more variables and more methods, and so on. This is how we can use this.
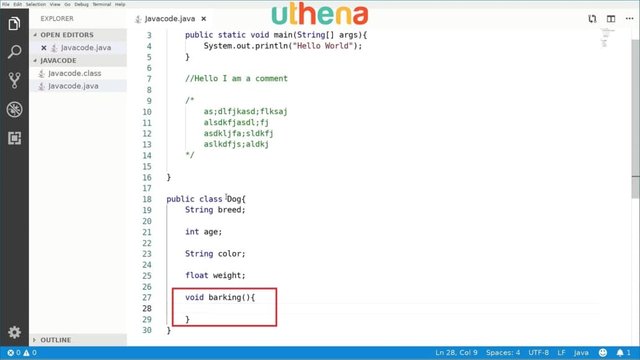
We Will Learn About Constructors.
We were talking just about classes, but a very important part of classes are constructors. So every class has a constructor. If we don’t write a constructor for a class the Java compiler will build one.
Every time a new object is created we have to somehow have a constructor either implicitly or explicitly but we have to. Constructors are just that they should have the same name as the class and, of course, a class can have more than one constructor.
Let’s understand how a constructor can work. We have a public class dog. The constructor method for dog would be public dog {} and that’s the constructor method.
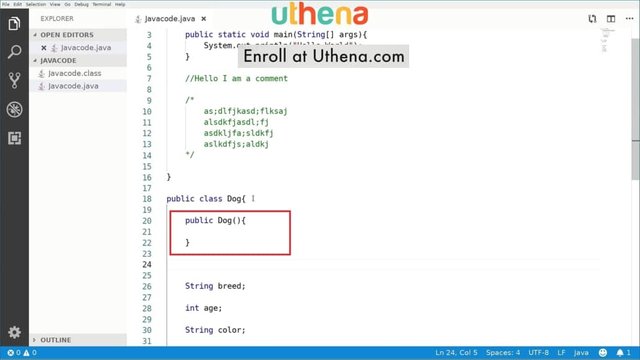
We also can have more than one constructor. In this case it would be for example public dog {} but in this case we can have a parameters here which are values that can be passed between methods.
We’re going to say public Dog(String name}, for example. We can initialize the dog by name.
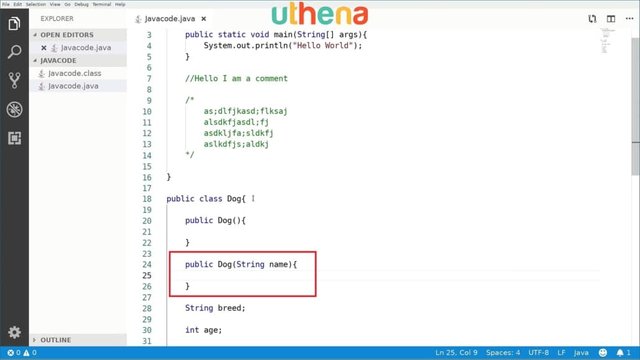
This is how constructor methods work inside classes. Pretty easy so far, right?
How Can We Create An Object?
Maybe we want to create an object inside our main method up here.
If we want to create a dog how do we do it? After we print “Hello World” we will say something like Dog and I will call it myDog as a name, and when I do this I can either create or call a constructor. To create a new element we type Dog myDog = new Dog{};, for example.
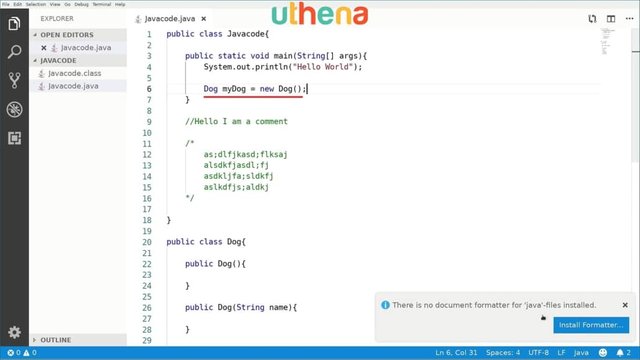
In Visual Studio code we can also install a code formatter for some programming languages. Let me see if I can do it now. I will click on this tall formatter. I will install the first one that appears here.
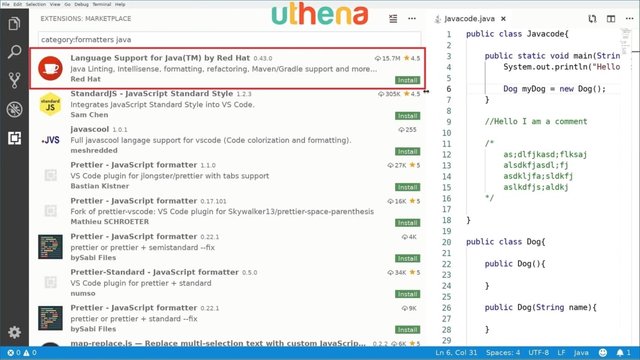
What this will enable us to do is it will color the code out and enable us to indent and order the code, and depending on the plugin it may have different features. This is kind of an advantage of using Visuals Studios code because we can download these additional plugins and make our lives a lot easier. As you can see it’s being installed. If you want you can also install it. I’m using this Language Support for Java by Red Hat which was the first that appeared in my list. It looks very popular. It has over 15 million downloads so it should be good.
Now, it says installed. It says, do you want to exclude the VS Code Java project settings files and all of this? I don't want to exclude. Because only syntax errors will be reported. Okay, no problem. That’s okay.
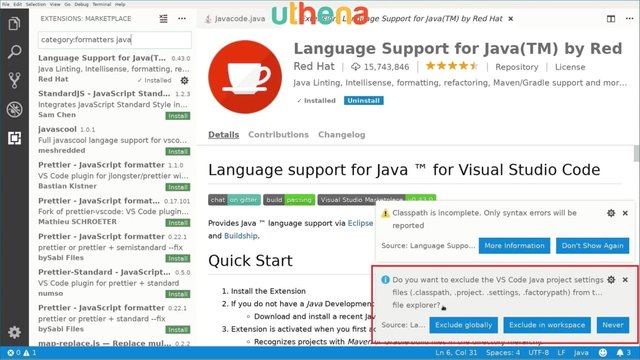
If I go back to us with my source code file, I can see that it has some colors coded right here which is kind of nicer. As you can see these strings are now in red and the other in blue and some green for the comments and so on.
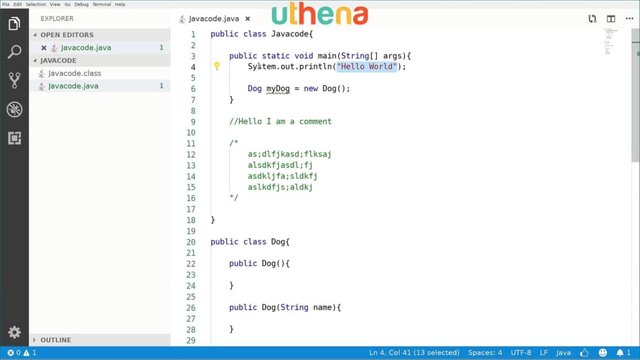
This is how we create a new element. We say myDog = new Dog and maybe I can also call the other constructor by saying Dog myDogWithName, for example. I will call the new Dog and I will pass the name of the dog. In this case, it will be Max.
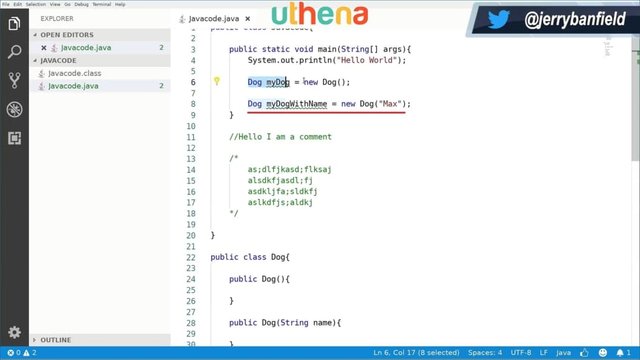
What I’m doing in the first case is I’m just calling a new dog. This means this will go down to this class and we call this method here but in the second case, I’m calling a new dog with a string parameter – with a dog name - so it will be called through here. This is how we can access an object and create an object and then maybe access with the different parameters. Maybe we want sometimes to create a dog with a name, maybe with an age, maybe with or without color, so it really depends on how we can do this.
What if I want to make a dog bark? How can I do it?
Right here after I have created myDogwithName, I can say myDog. and as you can see I can set the dog age, so I will say myDog.age = 10. I can also call a method so I can say myDog.barking.
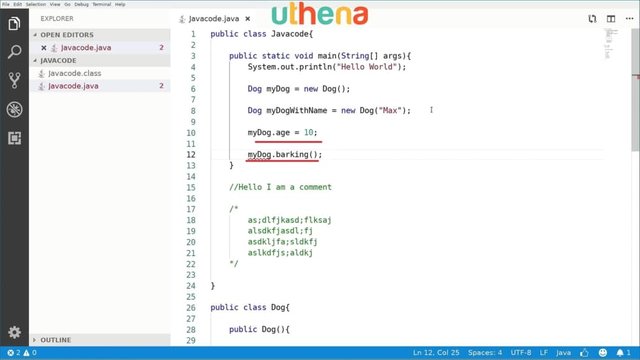
If I call this method the dog will bark if I have set that here. For example, I can make that by taking system.out.println. This println is the same as we did here when we printed (“Hello world”} and we’ll say system.out.println(“ barking”}.
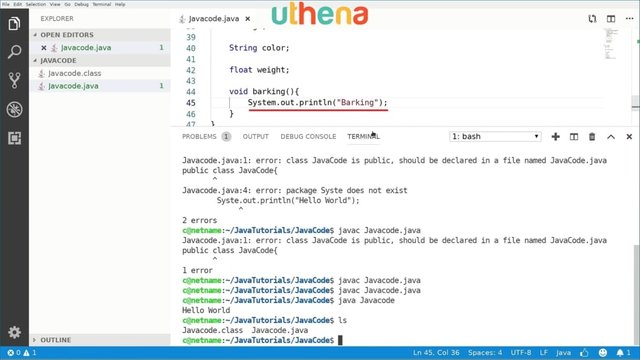
If we compile this and run so I’m going to say javac javcode.java it will be compiled, and here we have an error. As you can see it says dog is public should be declared in a file named Dog.java.
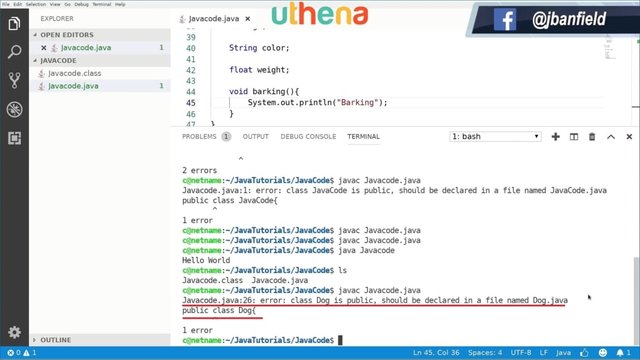
This says that dog the dog class should have its own file because it’s public so what if I want to make it private or no modifier at all? If I don’t use any modifier and I go again, I can see it is now compiled.
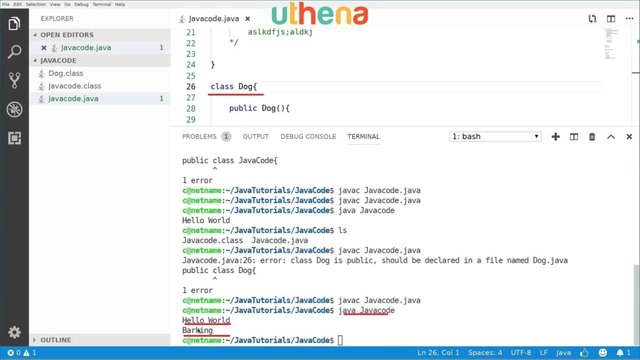
To run this I would say java Javacode. As you can see it says Hello World and then it says Barking. Why does it say Barking? Well because we’re creating a dog object here and we’re calling it the Barking method of the dog.
That’s what we can call different methods and access different ages. In this case, we’re asking the age or different types of data and so on. We can also create a method that will enable us to maybe set the dog age in a programmatic way.
We go inside the dog class and we’ll say public void setAge{}, for example, and we will pass an int right here, so public void setAge(intAge}.
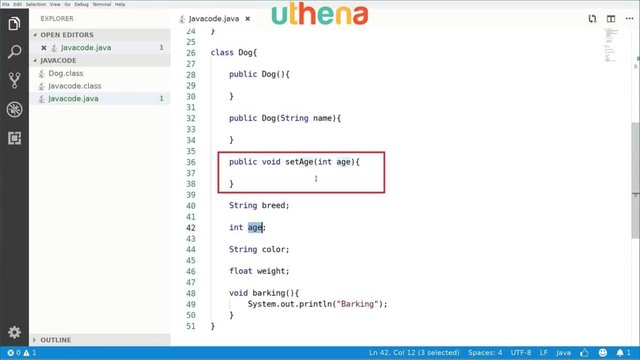
I want to access this age variable from here. How do I do it?
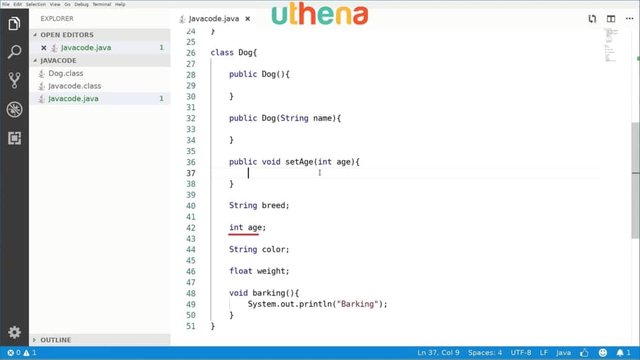
To access a variable from outside we will do the following: We will say this.age = age. What does it mean? This refers to this class so when I say this.age, the that I’m referring to is not this one but this one because I’m referring to the age that is inside the dog class. You can say this.age = age; and this will be set to the local age which is this one here.
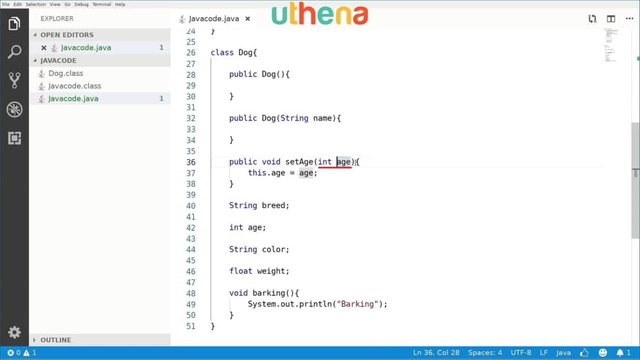
If we compile it compiles great so it works.
That’s how we can set the age but we can also get the age. To get the age you can say public int getAge() and when we call getAge what we will return is return age we can just return age. We can just return to age or we can return to this.age. We will return the same but here we don’t have another age to cause confusion to us.
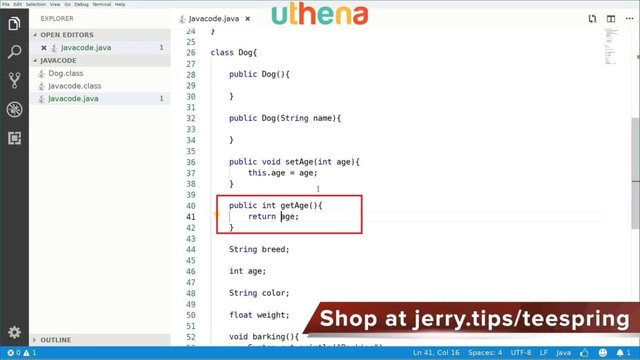
When we call this method from outside, for example, if we set the age here and then you say myDog.getAge() it will return a 10. If I create an integer here, int age = myDog.getAge() it will receive the number 10.
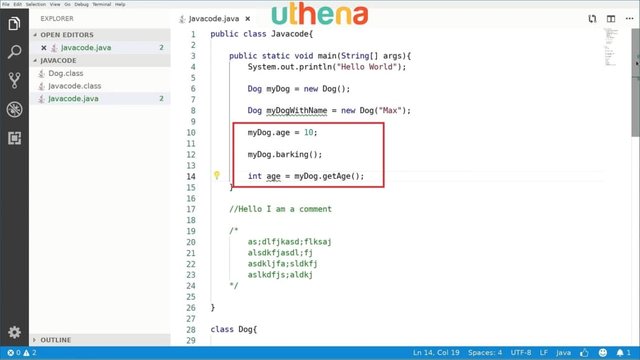
In this tutorial we learned how to set up Java Development, we learned how to create classes, we learned how to create methods, how to print something to the screen. We learned how to access objects and call constructors and call different modifiers and in the next class we will keep learning about more object-oriented concepts.
Part Two Of The Java Programming Language Course.
In the previous tutorial we learned the basics of Java; how to compile it help, how to fix errors, how to debug it and how to create object classes. In this tutorial, we will keep learning about these concepts but we’ll also learn about data types, integer, and floating-point data types. If we continue experimenting with this, we have created a dog, then we have created a dog with name, then we set the age of the dog and then we called barking.
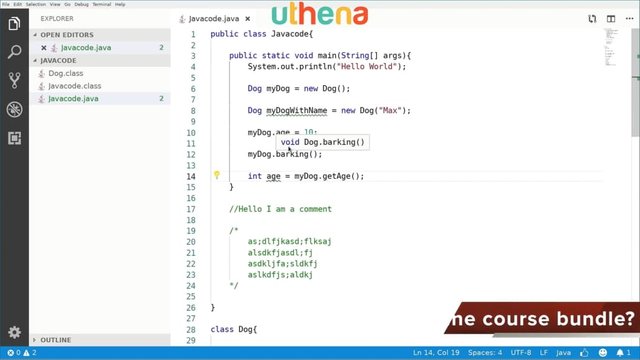
If you remember if we compile the code and if we run it, it says “Hello World” and then it says Barking which is exactly what we want, but now how can I know if the age that I set here took effect or not, right? I am calling this method right here the int age = myDog.get Age() method which if we go back to the dog class, this public int getAge{} will return the age of the dog.
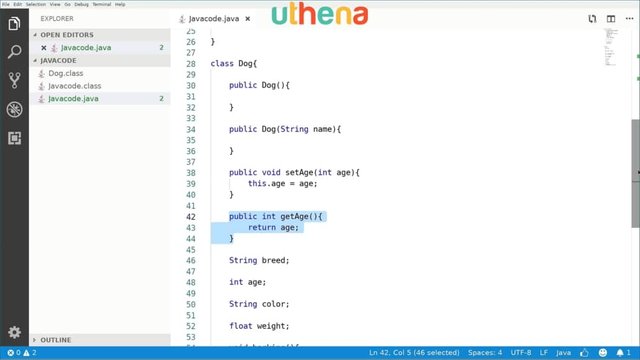
We will print this to check if it’s indeed 10. It is very simple. We call system.out.println(“ ”) and inside here we will pass the thing we want to print.
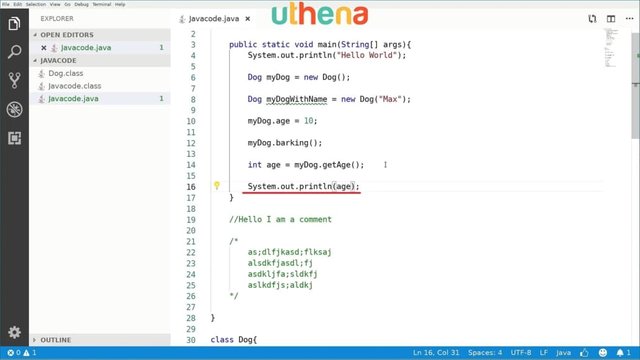
In this case, we want to print an integer so to transform from an integer to a string maybe, we can do the following: If we do nothing and just pass the age right here system.out.println(age); and if we compile it and then if we run it then the compiler is smart enough to transform this into a printable way. As you can see it has the 10 value right here.
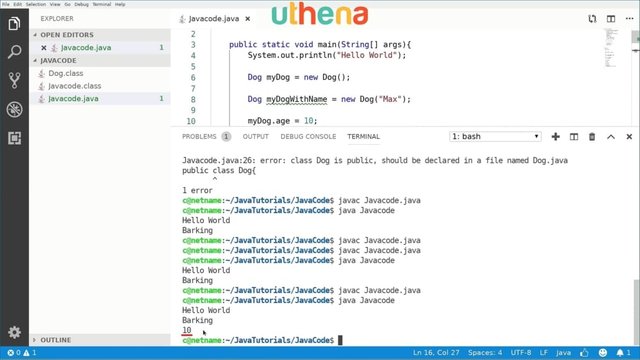
So the age we just said here it’s actually working. So if I comment on this and I run it again. Then it is set to zero because the default value of an integer is zero.
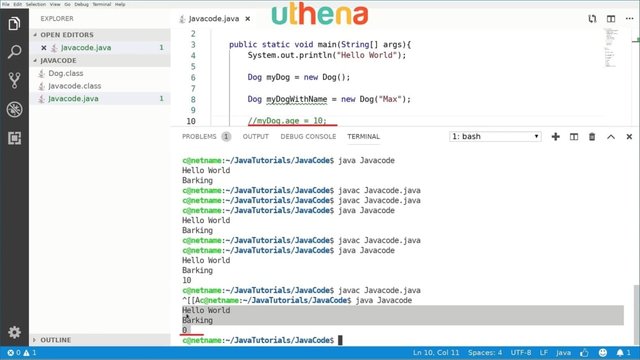
What if I want to tell it to set the age in an alternative way?
How?
Well, basically saying myDog setAge(10}; here. If I compile this and run it I will have 10.
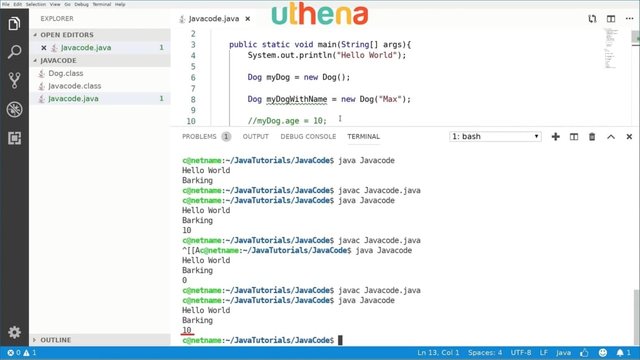
Here we notice that we have two ways of accessing class members like age. We can either do and say myDog.age = 10 or myDog.setAge(10}; and we will pass the 10 value here.
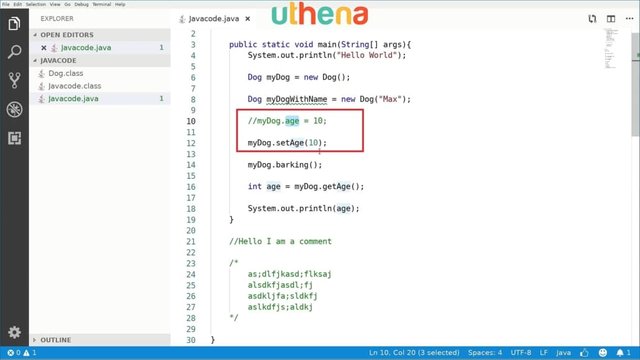
This is what we can basically call two things in two different ways. This is how this works. We will go and start learning about primitive data types, so we will start with integer data types. After these multi-line comments I will start writing about how the data types in Java work.
We have the smallest one called a byte. A byte, I call it my byte for example and I will set it to zero. I will put a comment describing what a byte does. This can contain different ranges of integers. So it goes from -128 to 127.
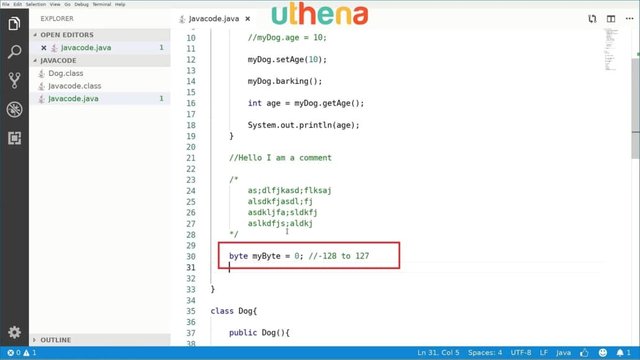
This means that mybyte variable can contain 256 possible values and those values are between are between the ones I just specified right here.
Then we have a short. So short, myShort. So all of these variables enabled us to store numbers. Byte, we can store small numbers. In short, we can start with a bit larger numbers. We can set it to 1,000 maybe because we really are able to store larger numbers in myShort but in myByte, we cannot go beyond 127. The largest number it can have is 127.
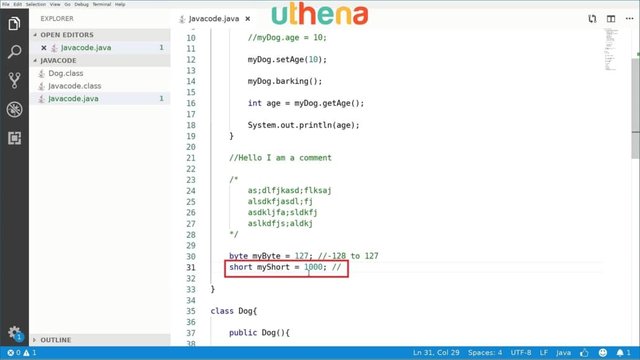
We have MyShort and the short data type is able to store values from the range starting at -32,768 to 32,767. So you can start with kind of 65,000, different numbers.
We also have larger like int. So an integer can store larger numbers. I will call it myInt. We’ll set this up for 100,000. They are really, really, big, big, big values. For simplicity reasons it will start with -2 billion to positive 2 billion and those are pretty, pretty, big numbers.
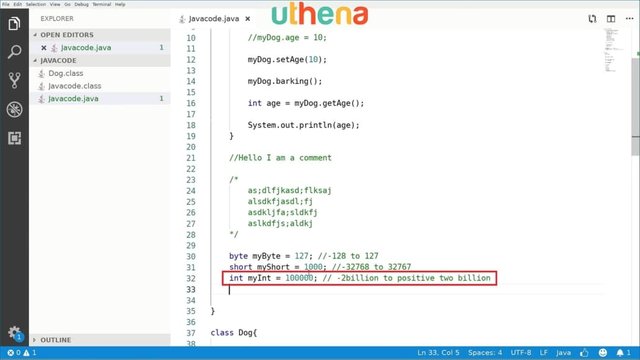
The integer is the most commonly used data type as you can see I used it to define the age of a dog. That’s how we use an integer. So integers are just numbers.
Then we have long’s. Well long is an even larger one. I will call long myLong and this is just insanely large. So it goes from, I don’t even know how to pronounce it, so let me see if I can figure out what this number is. We have, let me see, millions, billions, trillions, quadrillions, quintillions or something. I don’t know. It’s a huge number. So you can really store very large numbers in long. It’s very hard as you will learn you will be using those numbers. It says that the literal 100000000000 of type int is out of range but that’s because the default way that the Java program handles large numbers is an integer.
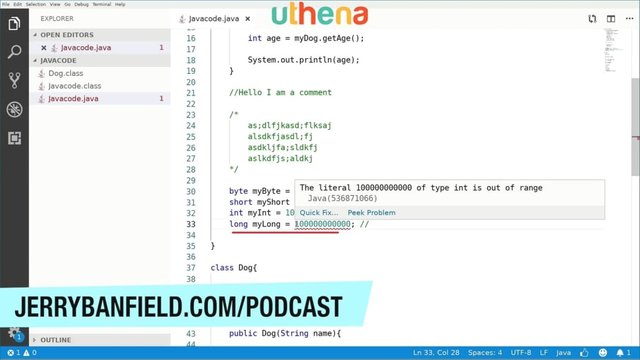
If we want to cast this as long it will be better understood by the program. Is this too large for a long? I don’t think this is too large for a long. Maybe the problem here is that this thing with the Visuals Studio code is not working as it should. That’s why we will ignore this warning because it’s kind of a bug of the system.
These are insanely large numbers. That’s what long is able to do. Just to make sure I didn’t type an error I will compile java Javacode.java and it says integer too large.
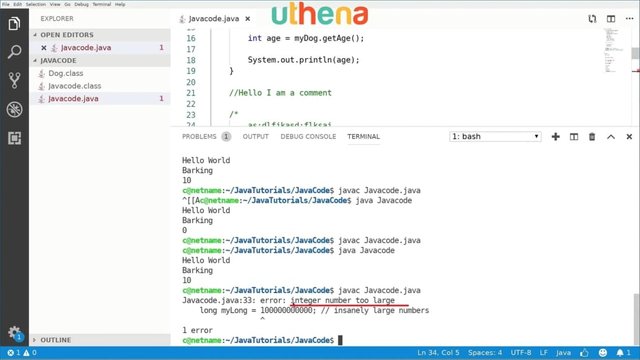
Somehow the thing is that it should fit here. We’ll figure this out later but we will decrease it. We will put it in a 10,000,000 or maybe a 100,000,000 or a 1,000,000,000. Now if we run it again it won’t say anything. So it says the integer number is too large. Well, I don’t really know why this is but it shouldn’t be the case.
Long, we can store insanely large numbers and we also have a char or a char which is used to store letters. So we can store different characters like letters of the alphabet or whatever characters we may want. I’ll call it myChar and to store a character, I have to do it between single quotes, for example, I put in char myChar = ‘R’;. That’s my character. How many possible bodies can this have? Well, a lot actually. It has values from 0 to 65,535.
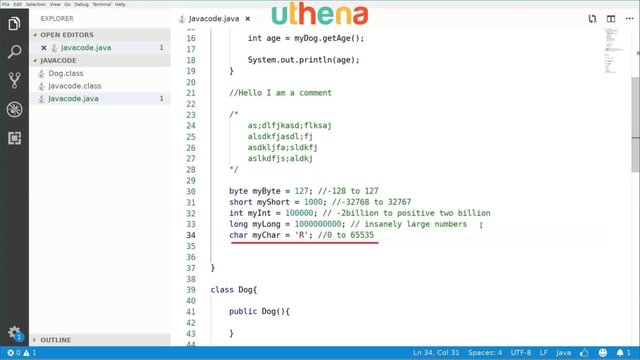
This is basically how we can define these different integer data types that will enable us to store numbers or, in this case, a character. So let’s compile it to check again everything is still working.
Yes, it’s still working.
These are the basic data types.
What can we do with these guys?
First of all we can compare them. So we can check if the number is equal, smaller or larger than the other one.
GET MORE OF THIS JAVA COURSE
Would you like to continue learning about Java Programming for Beginners Coding Android Apps from Development Kit Installation to Exceptions Course? If you are interested will you please buy the complete course, Java Programming for Beginners Coding Android Apps from Development Kit Installation to Exceptions Course, on the Uthena Education Platform..
You can also get the first I hour 35 minutes of the course completely for free on YouTube.
Thank you for reading the blog post or watching the course on YouTube.
I love you.
You’re awesome.
Thank you very much for checking out Java Programming for Beginners Coding Android Apps from Development Kit Installation to Exceptions Course and I hope to see you again in the next blog post or video.
Love,
Jerry Banfield.
Posted from my blog with SteemPress : https://jerrybanfield.com/java-programming-beginners-development-kit-installation-exceptions/
Java appears to be more complex than HTML. Perhaps not by much. I know Java is a kind of code. I know some HTML. But is Java also an operating system or program? I'm asking because it appears as if there is a java terminal. I use Ubuntu as an operation system for my laptop. So, I have a terminal. So, I thought I saw what looked like a Java terminal. I could be wrong.
Congratulations! Your post has been selected as a daily Steemit truffle! It is listed on rank 4 of all contributions awarded today. You can find the TOP DAILY TRUFFLE PICKS HERE.
I upvoted your contribution because to my mind your post is at least 5 SBD worth and should receive 389 votes. It's now up to the lovely Steemit community to make this come true.
I am
TrufflePig
, an Artificial Intelligence Bot that helps minnows and content curators using Machine Learning. If you are curious how I select content, you can find an explanation here!Have a nice day and sincerely yours,
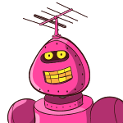
TrufflePig