Steem-js and Materialize Css Join Forces, Tutorial to get created posts #1
Repository
- https://github.com/steemit/steem-js
- https://github.com/Dogfalo/materialize
- https://github.com/jquery/jquery
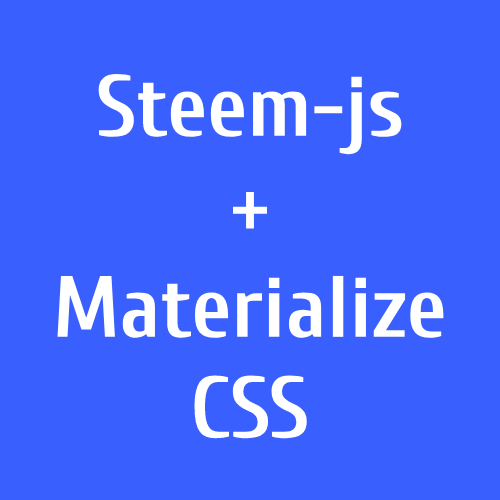
What Will I Learn?
- How to get posts from steemit.
- How to use collection items in Materialize css.
- How to show returned value from API to an HTML page.
- How to show posts with images.
- How to handle if no image is available in the steemit post.
Requirements :
- Get Materialize Css from Here and set up in your project directory.
- Get steem-js from here and follow the official GitHub page instructions to setup steem-js in your project directory.
- Get Jquery minified version from Here and the website instruction to add it into your project.
- Any Text Editor is required (Vs code/ Atom or any other).
- Basic Understanding of Materialize CSS collections
Difficulty
Choose one of the following options:
- Intermediate
Tutorial Contents
I will be covering that how to list steemit posts on HTML Web Page using Materialize CSS and Steem-js API. In this tutorial I will try to explain about steem-js API method to list all the posts accordingly, Then how to easily use Materialize CSS to show posts in a list view with images. Like this:
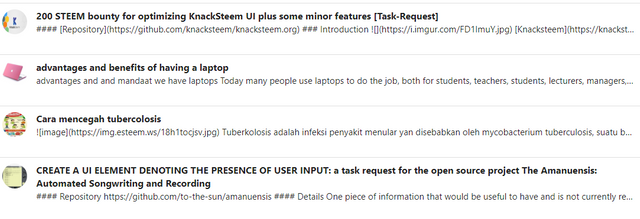.png)

Tutorial Starts From Here:
Setup a directory of project with Materialize CSS & Steem-js. You will then have index.html and project structure that looks like this.
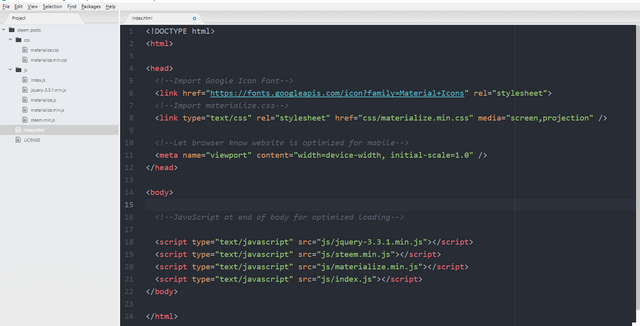.png)
Now you can see the index.html and the project structure on the left side. Make sure you have the same pattern too, because adding Jquery script
tag after Materialize script
tag will override the functions used by Materialize CSS. With this, the Materialize CSS functions will not be able to recognize by Jquery library.

Now using this function of steem-js, We will get list of newly created posts upto first 100.
// Steem-js method to return steemit posts based on created .
steem.api.getDiscussionsByCreated({"tag": "steemit", "limit": 20}, function(err, result) {
console.log(err, result);
});
The first argument is a query
in which the required fields limit
should be filled and the tag is optional. The tag
field is used to define, for what tag we want to get posts of and to the limit of posts we use limit
field, we decided 100 (max by steemd) so filled 20 over there and the tag will be steemit.
If you want to get a result for all the tags then simply remove the tag property from the query or give tag an empty string like this "tag":""
.

We need Jquery code to append collection item(Steem posts) in HTML page. First, add this code inside the index.html
body
tag
<div class="container">
<ul class="collection">
(html comment removed: Expand in this tag )
</ul>
</div>
Now inside the ul
tag we will append list items that contains the steemit Post image,title,description(truncated). You can always read how the classes of material design works over the Materialize CSS page. Your index.html Should look like this.
<!DOCTYPE html>
<html>
<head>
(html comment removed: Import Google Icon Font)
<link href="https://fonts.googleapis.com/icon?family=Material+Icons" rel="stylesheet">
(html comment removed: Import materialize.css)
<link type="text/css" rel="stylesheet" href="css/materialize.min.css" media="screen,projection" />
(html comment removed: Let browser know website is optimized for mobile)
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
</head>
<body>
<div class="container">
<ul class="collection">
</ul>
</div>
<script type="text/javascript" src="js/jquery-3.3.1.min.js"></script>
<script type="text/javascript" src="js/steem.min.js"></script>
<script type="text/javascript" src="js/materialize.min.js"></script>
<script type="text/javascript" src="js/index.js"></script>
</body>
</html>

Add following code to your
index.js
file.
//Running steem method on document ready when script tag initialized.
//There is not a need for document.ready funcion but you can use them for practice.
steem.api.getDiscussionsByCreated({
"tag": "steemit",
"limit": 10
}, function(err, result) {
$('.collection').empty();
for (i = 0; i < result.length; i++) {
//Looking for image, if existed then use the first of the post and if not then the use defined image.
let imageUrl = JSON.parse(result[i].json_metadata);
let image = "https://48tools.com/wp-content/uploads/2015/09/shortlink.png";
if (imageUrl.hasOwnProperty('image')) {
console.log("Has property image");
if (imageUrl.image[0] !== "") {
image = imageUrl.image[0];
} else if (imageUrl.image[0] == "") {
image = "https://48tools.com/wp-content/uploads/2015/09/shortlink.png";
}
}
// Replace if any html tag occur in post
let bodyContent = result[i].body;
let prefined = bodyContent.replace(/(<([^>]+)>)/ig, "");
//Append <ul> tag body with proper steem post image, title and description.
$(".collection").append("<li class='collection-item avatar'> \
<a href='http://steemit.com" + result[i].url + "' style='color: inherit;'> \
<img src='" + image + "' alt='' class='circle'> \
<span class='title' style='font-weight:bold;'>" + result[i].root_title + "</span> \
<p class='truncate'>" + prefined + "</p> \
</a> \
</br>\
</li>");
}
});

Explaining code:
While running the above code. First, we fetch the first 10 latest posts from
steemit
tag.If the result is not an error then a loop starts that traverse each post from
0
index upto9
th index.In each loop cycle we look for JSON meta_data for the image property, If exist then add the first image as a thumbnail or use the default image link if not have
image
attribute in JSON or if it's empty.
Then we simply replacing whole body text of steem post with HTML symbols. So it will not disturb the overall layout inside
p
tag.Then we are appending
collection
class orul
to addli
for steemit posts.

Output:
Save all the files and then double click on
index.html
to open it inside the browser. Then the output display over it.
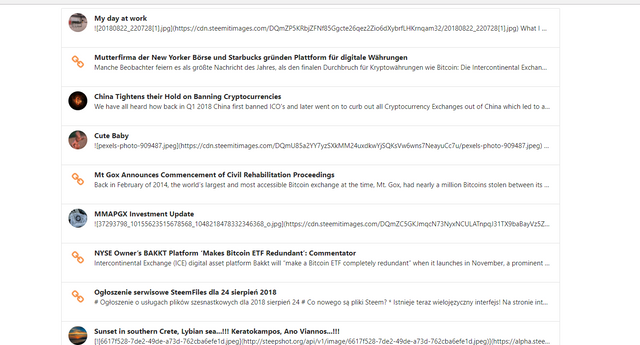.png)
Note: While some users use HTML tags in writing body of the post, So it will intercept over the original code and In the next part, I will try to cover how to use tab view and then how to show posts based on "tending", "hot", "promoted" and "created".
Proof of Work Done
https://github.com/genievot/steem-posts-tut

Thank you for your contribution.
After the review we have the following suggestions:
Your contribution has been evaluated according to Utopian policies and guidelines, as well as a predefined set of questions pertaining to the category.
To view those questions and the relevant answers related to your post, click here.
Need help? Write a ticket on https://support.utopian.io/.
Chat with us on Discord.
[utopian-moderator]
Thank you for your review, @portugalcoin!
So far this week you've reviewed 10 contributions. Keep up the good work!
Very interesting suggestions @portugalcoin, thanks for reviewing it.
Hey @genievot
Thanks for contributing on Utopian.
We’re already looking forward to your next contribution!
Want to chat? Join us on Discord https://discord.gg/h52nFrV.
Vote for Utopian Witness!
Hi @genievot! We are @steem-ua, a new Steem dApp, computing UserAuthority for all accounts on Steem. We are currently in test mode upvoting quality Utopian-io contributions! Nice work!