Easy Programming Tip -- change a string to uppercase/lowercase (ASCII) C and C++ (beginner friendly)
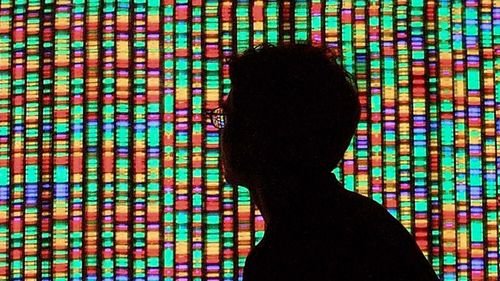
Image source
Hey guys! Here is a quick little programming tip about going from uppercase to lowercase, or lowercase to uppercase in a C or C++ string. Honestly, you can change a character to whatever character you want if you know the ASCII values. Here is an ASCII table:
We are comparing the "Dec" column to the "Chr" column (decimal to character). Notice how each corresponding decimal number of an uppercase letter is 32 less than the number of its lowercase match.
So to change a letter from uppercase to lowercase, all that we need to do is add 32:
char c = 'A';
printf("%c\n", c);
c += 32;
printf("%c\n", c);
Output:
A
a
Going the other way:
char c = 'a';
printf("%c\n", c);
c -= 32;
printf("%c\n", c);
Output:
a
A
It is that simple! Here is a program I wrote that changes all lowercase values in a string to uppercase:
#include<stdio.h>
#include<string.h>
int main()
{
printf("Enter a sentence (50 char max): ");
char input[50];
fgets(input, 50, stdin);
int x;
for(x = 0; x < strlen(input); x++)
if(input[x] > 96 && input[x] < 123) // only change if it is lowercase -- from 97 to 122
input[x] -= 32;
printf("%s", input);
return 0;
}
Output:
[cmw4026@omega test]$ gcc upper.c
[cmw4026@omega test]$ ./a.out
Enter a sentence (50 char max): I like EATING dogs?
I LIKE EATING DOGS?
[cmw4026@omega test]$
I hope that was useful! It is important to understand how ASCII characters work in my opinion. Leave any suggestions in the comments.
@charlie.wilson
Playing around with ASCII is cool
Thanks for sharing this.
No problem, thanks for reading!
Would it be difficult to program a Pidgin plugin that sends the text displayed to the windows clipboard? It would be cool if pidgin could talk, and most TTS has a clipboard monitor.
Probably not, but I have never tried it... It sounds simple enough.
If it seems interesting I'd be a willing guinea pig to try it out. It also could make for a fun programming post.