Programming tutorials for beginners PART 2: object-oriented programming (OOP)
If you missed part 1, i suggest to take a look. It has some good information in it to understand what programming is really all about.
Introduction
Programming is all about information flow. To make information flow in programming we have the following tools to our disposal:
- Events
- Methods
- Properties
1. Events
Events are signals that something has happened. Events execute an empty piece of code automatically, which programmers can fill with anything that needs to happen. Common examples of events are: "a key on the keyboard has been pressed" or "a button has been clicked".
2. Methods
Methods are collections of code that belong together that can be executed at any time you want. Common examples of methods are: "update data on screen" or "initialization".
3. Properties
Properties are pieces of information that are saved in the memory of the computer. Common examples of properties are: "color" or "size".
Object-ortiented programming
Object-oriented programming (OOP) is a very structured way of programming to make it clearer for humans to write code. As the name suggests, it works with objects. An object is a structured collection of information and can be put into the memory of a computer by executing code from something named a class so it can be used by anything that has access to the memory. This process is called "instancing" (creating an instance of an object).
Classes
A class is a blueprint of an object. A class describes all events, methods and properties that the object will gain when it's instanced. The beautiful thing about this blueprint is that you can also use this blueprint in other blueprints! An example of this is a "vehicle". Cars and bicycles are both vehicles , but have distinct differences.
Practical example
Let's look at how much easier programming is when using OOP. We are going to use the car and bicycle in the following example project:
The user has to pick a vehicle. After picking the vehicle the user can:
- See properties of the chosen vehicle (we'll keep it simple with just the weight and color)
- Execute a warning sound based on what vehicle was chosen (horn for the car and bell for the bicycle)
Implementation 1 (no OOP):
Main application
Events:
- User has chosen a vehicle (VehicleChosen with the property ChosenVehicle as parameter)
- User wants to see properties of the chosen vehicle (VehiclePropertiesRequest)
- User wants to execute a warning sound (WarningSoundRequest)
Methods:
- Execute code at the start of the application (Initialize)
- Show bicycle properties (ShowBicycleProperties)
- Show car properties (ShowCarProperties)
- Ring the bell of the bicycle (RingBell)
- Hunk the horn of the car (HunkHorn)
Properties:
- ChosenVehicle (number)
- Car weight (number)
- Car color (color)
- Bicycle weight (number)
- Bicycle color (color)
VehicleChosen
- If bicycle is chosen set ChosenVehicle to 1
- If car is chosen set ChosenVehicle to 2
VehiclePropertiesRequest
- If the user has chosen the bicycle: ShowBicycleProperties
- If the user has chosen the car: ShowCarProperties
WarningSoundRequest
- If the user has chosen the bicycle: RingBell
- If the user has chosen the bicycle: HunkHorn
Initialize
- Load the vehicle data
ShowBicycleProperties
- Show bicycle weight and car color
ShowCarProperties
- Show car weight and car color
RingBell
- Ring the bell
HunkHorn
- Hunk the horn
Implementation 2 (OOP):
Blueprint for vehicle
Methods:
- Execute code at the start of the application (Initialize)
- WarningSound
Properties:
- Weight (number)
- Color (color)
Blueprint for bicycle
Inherit everything from the vehicle blueprint.
WarningSound
- Ring the bell
Blueprint for car
Inherit everything from the vehicle blueprint.
WarningSound
- Hunk the horn
Main application
Events:
- User has chosen a vehicle (VehicleChosen with the property ChosenVehicle as parameter)
- User wants to see properties of the chosen vehicle (VehiclePropertiesRequest)
- User wants to execute a warning sound (WarningSoundRequest)
Properties:
- ChosenVehicle (vehicle)
VehicleChosen
- If bicycle is chosen set ChosenVehicle to bicycle
- If car is chosen set ChosenVehicle to car
VehiclePropertiesRequest
- Show ChosenVehicle.weight and ChosenVehicle.color
Initialize
- Load the vehicle data
WarningSoundRequest
- Execute ChosenVehicle.WarningSound
Closing arguments
You can see how much more logical and clear it is with OOP and this is a relatively simple application. Imagine having to write software with 100's of objects without OOP, it's going to make your head explode really quickly! I hope you start to see how awesome programming is if you know what you're doing. So if you like a challenge and a good salary, programming might be just the thing for you :)
See you in the next lesson!
Great that you give beginners a chance to learn how to programm! You do really support the steemit community. Many up votes for you mate :)
Thanks @calamus056
Now I be like
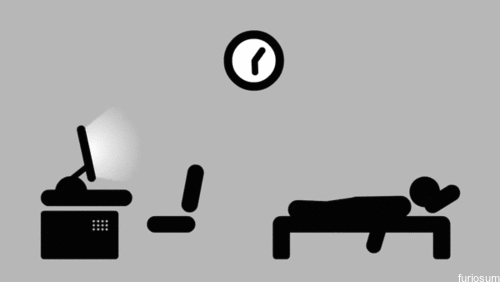
Welcome to the club (of no sleep) :)
Thanks for breaking that down in laymen's terms. I'm am just starting to get my feet wet in coding, but could easily follow along with your content.
Awesome to hear. Boy oh boy, did i have a hard time trying to explain it to beginners. One thing that's hard for programmers is to keep things simple haha.
Haha it really is another language and once you think in it it becomes second nature so you have to stop yourself and think how you learned it which usually helps a lot. Some people are just wired to understand it though.