How to make simple-order-taking-app android
Hello Steemians, nowadays, Android app is very popular especially in Asia. That makes many people want to become an Android Developer. I think it is very nice because there will be many amazing app in the play store. Now, i want to show you how to implement simple order taking app.
Requirements:
- Android Studio 3.0 version (recommended) or lower
- Java Sdk
- Laptop, PC or Macbook
- Willing to learn
- Android Emulator (GenyMotion, AVD, etc) or real Android device
The Beginning Steps
First of all, i assume you can install Android studio by yourself and Java Sdk. You can go to https://developer.android.com/studio/install.html for the detail. Okay, Let’s start. I make this become step by step so it is easy to follow.
- Open up your Android Studio
- Create new Project and name it with awesome name you want to, then click Next
- Choose your target devices, in this case i choose API 15 for minimum SDK which is recommended by me, Next
- Choose Empty Activity and click Next
- Then Finish and wait till the gradle sync finish
Create Project
Create Target Device
Choose Activity
Finish
Libraries that use in this simple project via gradle
- AppCompat support android
compile 'com.android.support:appcompat-v7:25.3.1'
- Jakewharton butterknife
compile 'com.jakewharton:butterknife:8.6.0'
- Simple Item decoration
compile 'com.bignerdranch.android:simple-item-decoration:1.0.0'
- RecyclerView
compile 'com.android.support:recyclerview-v7:25.3.1'
Butterknife we use to bind our view easily so you don't need to use findViewById which is very mess if your project contains a lot of view. RecyclerView is use to display list item that use loop and have virtualization inside it.
Libbove version is when i am making this project, you can use latest version too :).
Creating Model
Model is the one item representation, just imagine with one record in a table :).
If we want to use Recyclerview, it is good to make the model first. You can copy below code. There are accessors and mutators as well.
package upwork.andri.simpleordertaking.models;
import java.util.ArrayList;
public class ItemOrder {
private String name;
private int price;
private int qty;
public ItemOrder(String name, int price) {
this.name = name;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public int getQty() {
return qty;
}
public void setQty(int qty) {
this.qty = qty;
}
public void incQty() {
this.qty++;
}
public void decQty() {
this.qty--;
}
public static ArrayList<ItemOrder> createOrderItems() {
ArrayList <ItemOrder> items = new ArrayList<>();
String[] itemNames = {"Coffee", "Tea", "Latte", "Chocolate", "Milk"};
int[] itemPrice = {15, 10, 12, 20, 15};
for (int i = 0; i < itemNames.length; i++) {
items.add(new ItemOrder(itemNames[i], itemPrice[i]));
}
return items;
}
}
The name property is just an attribute describe the item name.
The price property is an int attribute that you may want to use to.
The qty property is quantity of one item.
The property you add another one depending on your need.
Creating Adapter
We can say adapter is like a controller that manipulates data from network, database, etc to bind to the view. Every RecyclerView Adapter must implements this three methods:
- onCreateViewHolder -> This is where we inflate the layout from xml using LayoutInflater on this method
- onBindViewHolder -> This is where we manipulates data from model to the view
- getItemCount -> This is where we determine size of the items in the adapter
And we need a ViewHolder class that extends RecyclerView.ViewHolder class to bind with each item in the RecyclerView. Below is the adapter code which i assigned a list when instantiate adapter via constructor.
package upwork.andri.simpleordertaking.ui;
import android.content.Context;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageButton;
import android.widget.TextView;
import java.util.List;
import butterknife.BindView;
import butterknife.ButterKnife;
import butterknife.OnClick;
import upwork.andri.simpleordertaking.R;
import upwork.andri.simpleordertaking.models.ItemOrder;
public class ItemOrderAdapter extends RecyclerView.Adapter<ItemOrderAdapter.ViewHolder> {
private List<ItemOrder> mItemOrders;
private Context mContext;
public ItemOrderAdapter(Context context, List<ItemOrder> itemOrders) {
mContext = context;
mItemOrders = itemOrders;
}
@Override
public ItemOrderAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
Context context = parent.getContext();
LayoutInflater inflater = LayoutInflater.from(context);
View itemOrderView = inflater.inflate(R.layout.item_order, parent, false);
return new ViewHolder(itemOrderView);
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
final ItemOrder itemOrder = mItemOrders.get(position);
holder.mItemName.setText(itemOrder.getName());
holder.mItemPrice.setText("$ ".concat(String.valueOf(itemOrder.getPrice())));
}
@Override
public int getItemCount() {
return mItemOrders.size();
}
class ViewHolder extends RecyclerView.ViewHolder {
@BindView(R.id.item_name)
TextView mItemName;
@BindView(R.id.item_price)
TextView mItemPrice;
@BindView(R.id.item_qty)
TextView mItemQty;
@OnClick(R.id.dec_qty) void incItem(){
mItemOrders.get(getAdapterPosition()).decQty();
mItemQty.setText(String.valueOf(mItemOrders.get(getAdapterPosition()).getQty()));
}
@OnClick(R.id.inc_qty) void decItem(){
mItemOrders.get(getAdapterPosition()).incQty();
mItemQty.setText(String.valueOf(mItemOrders.get(getAdapterPosition()).getQty()));
}
ViewHolder(View itemView) {
super(itemView);
ButterKnife.bind(this, itemView);
}
}
}
The item_order.xml layout code is below, you can change to what you prefer
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:orientation="vertical">
<TextView
android:id="@+id/item_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginBottom="@dimen/keyline_xs"
android:textAppearance="@style/TextAppearance.AppTheme.Title"
tools:text="Black Latte Coffee" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<TextView
android:id="@+id/item_price"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_gravity="center_vertical"
android:layout_height="wrap_content"
tools:text="$20"/>
<ImageButton
android:id="@+id/dec_qty"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@null"
android:contentDescription="@string/decrement"
android:src="@drawable/btn_minus_background" />
<TextView
android:id="@+id/item_qty"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_vertical"
android:ems="2"
android:gravity="center_horizontal"
android:text="@string/zero"
android:textAppearance="@style/TextAppearance.AppTheme.Medium"
android:textColor="?attr/colorPrimary"
android:textStyle="bold" />
<ImageButton
android:id="@+id/inc_qty"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@null"
android:contentDescription="@string/decrement"
android:src="@drawable/btn_plus_background" />
</LinearLayout>
(html comment removed: <TextView)
(html comment removed: android:id="@+id/item_name")
(html comment removed: android:layout_width="wrap_content")
(html comment removed: android:layout_height="wrap_content")
(html comment removed: tools:text="12" />)
</LinearLayout>
Note: tools:text just preview mode, it won't show when you're running the app.
If you want to set decoration line, you can copy the code, but this is optional.
package upwork.andri.simpleordertaking.thirdparty;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.drawable.Drawable;
import android.support.v4.content.ContextCompat;
import android.support.v7.widget.RecyclerView;
import android.view.View;
import upwork.andri.simpleordertaking.R;
public class SimpleDividerItemDecoration extends RecyclerView.ItemDecoration {
private Drawable mDivider;
public SimpleDividerItemDecoration(Context context) {
mDivider = ContextCompat.getDrawable(context, R.drawable.line_divider);
}
@Override
public void onDrawOver(Canvas c, RecyclerView parent, RecyclerView.State state) {
int left = parent.getPaddingLeft();
int right = parent.getWidth() - parent.getPaddingRight();
int childCount = parent.getChildCount();
for (int i = 0; i < childCount; i++) {
View child = parent.getChildAt(i);
RecyclerView.LayoutParams params = (RecyclerView.LayoutParams) child.getLayoutParams();
int top = child.getBottom() + params.bottomMargin;
int bottom = top + mDivider.getIntrinsicHeight();
mDivider.setBounds(left, top, right, bottom);
mDivider.draw(c);
}
}
}
Connect it all
After that, go to activity_main.xml setup recyclerview layout like below code. As always, you may change what you prefer.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="upwork.andri.simpleordertaking.MainActivity">
<android.support.v7.widget.RecyclerView
android:id="@+id/rv_item_order"
android:layout_width="match_parent"
app:layoutManager="android.support.v7.widget.LinearLayoutManager"
android:layout_height="wrap_content">
</android.support.v7.widget.RecyclerView>
<Button
android:id="@+id/order_button"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:text="@string/order"/>
</RelativeLayout>
Then, go to MainActivity.java and copy below code.
package upwork.andri.simpleordertaking;
import android.graphics.drawable.Drawable;
import android.os.Bundle;
import android.support.v4.content.ContextCompat;
import android.support.v7.app.AppCompatActivity;
import android.support.v7.widget.RecyclerView;
import android.util.Log;
import com.dgreenhalgh.android.simpleitemdecoration.linear.DividerItemDecoration;
import butterknife.BindView;
import butterknife.ButterKnife;
import butterknife.OnClick;
import butterknife.Unbinder;
import upwork.andri.simpleordertaking.models.ItemOrder;
import upwork.andri.simpleordertaking.thirdparty.SimpleDividerItemDecoration;
import upwork.andri.simpleordertaking.ui.ItemOrderAdapter;
public class MainActivity extends AppCompatActivity {
private Unbinder unbinder;
@BindView(R.id.rv_item_order)
protected RecyclerView mItemOrderRV;
@OnClick(R.id.order_button) void order(){
Log.d("TAG", "ORDER CLICKED");
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
unbinder = ButterKnife.bind(this);
ItemOrderAdapter itemOrderAdapter = new ItemOrderAdapter(this, ItemOrder.createOrderItems());
mItemOrderRV.setAdapter(itemOrderAdapter);
// mItemOrderRV.addItemDecoration(new SimpleDividerItemDecoration(this));
Drawable dividerDrawable = ContextCompat.getDrawable(this, R.drawable.line_divider);
mItemOrderRV.addItemDecoration(new DividerItemDecoration(dividerDrawable));
}
@Override
protected void onDestroy() {
super.onDestroy();
unbinder.unbind();
}
}
If you want to display line, just simply uncomment it the // mItemOrderRV.addItemDecoration(new SimpleDividerItemDecoration(this));
line.
This is the result :D
Okay, we’re done. You can play around with the code. I made this post to share my knowledge to everyone. If there are some mistakes i am sorry but i will try to correct it because we are all learners. I also give github link below, so you can clone or download this entire source. I will make another post about how to make something again and hopefully better and help everyone to get started easily with development. Note that this app still contains lack of validation. You're welcome if you want to contribute via pull request. Thank you for reading.
Proof of work
The link https://github.com/eternalBlast/simple-order-taking-app
Screenshot
Posted on Utopian.io - Rewarding Open Source Contributors
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @andrixyz I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x
Your contribution cannot be approved yet, because it is in the wrong category. The correct category for your post is
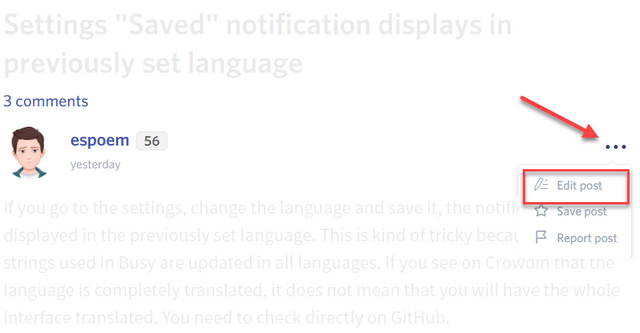
Tutorial
. See the Utopian Rules. Please edit your post to use the right category at this link, as shown below:You can contact us on Discord.
[utopian-moderator]
Congratulations @andrixyz! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Approved haha
Nice!!