How Communicating Between Components With Vuex , State Management From Vue
image-source : https://vuex.vuejs.org/en/images/flow.png
A few days ago I shared , how to communicating components with props, this time I want to share another way of communicating between components, that is with vuex. by using vuex the data can be centralized, and we can easily access it in any component we need. there is no rule for folder structure inside vuex, but in this tutorial will use structure which recommended by vuex official.
When We Start Should Using Vuex ?
If you just learn and use vuejs, it should not use vuex. if you want to communicate data only one way, such as parent to child or child to parent, which between one and the other there is no data link, you can use Props. but if you have the data you want to share to several components at once and the components that receive the data can be as parent or child, you should start learning and using vuex. if you ever learn react, in react we know flux and redux. vuex has the same concept with flux and redux, but vuex is easier to learn for me.
How to Install Vuex ?
The first step you should open your file directory, this my file directory D:\vueproject\vuecli\vue-project open the command window here and then install with npm D:\vueproject\vuecli\vue-project>npm install vuex --save
npm install vuex --save
Register the vuex in main.js
// The Vue build version to load with the `import` command
// (runtime-only or standalone) has been set in webpack.base.conf with an alias.
import Vue from 'vue'
import App from './App'
import Vuex from 'vuex'
import {store} from './store';
Vue.use(Vuex);
Vue.config.productionTip = false
/* eslint-disable no-new */
new Vue({
el: '#app',
template: '< App/ >',
components: { App },
store
})
Import the vuex
import Vuex from 'vuex'
Vue.use(Vuex);
Import the store
import {store} from './store';
new Vue({
el: '#app',
template: '< App/ >',
components: { App },
store
})
import {store} from './store'
: Import the index.js from folder store, as the default index.js will be choosen
Make Structure Folder
We will separate the files with their respective functions
1 . State.js
State.js is our data source, data can come from API or statics data that we write hardcode. i will make a simple example , i will hardocode one object and this object will be use in all components . . in this block code, i just return the value of name .
export default{
name:'Jhon Doe'
}
2 . Getters.js
If the state contains pure data, but in getters.js contains data already in process, getters that provide data for our components. in this block code,
export default {
name:state =>{
return state.name
}
}
3 . Types.js
Types are a kind of abstraction, so when someone sees our code they will know what methods are available, or some sort of place to register the method or function of our application. although we can not use it, but in suggest to use it, types are also useful as references
export const CHANGE_NAME = 'CHANGE_NAME'
4 . Mutations.js
The only way to actually change state in a Vuex store is by committing a mutation. Vuex mutations are very similar to events: each mutation has a string type and a handler. The handler function is where we perform actual state modifications, and it will receive the state as the first argument
import * as mutations from './types'
export default {
[mutations.CHANGE_NAME](state){
state.name = "Michael Jackson"
},
}
5 . Actions
MapActions is a collection of methods that used in our web app
import * as mutations from './types'
export default{
changeName(context){
context.commit(mutations.CHANGE_NAME)
},
}
import * as mutations from './types'
: import the const in "type.js"
changeName(context)
: its the name of function and (context) is required parameters from vuex.
context.commit(mutations.CHANGE_NAME)
: its the way to call mutations , the name of mutations is "CHANGE_NAME"
6 . Index.js
Index.js will become the representative of all file file store.js to be imported, so we do not have to import the file one by one. we just need to import index.js to use state.js, actions.js, getter.js, and mutations.js
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
import state from './state';
import actions from './actions';
import getters from './getters';
import mutations from './mutations';
export default new Vuex.Store({
state,
getters,
mutations,
actions,
})
How to Use Vuex in Component
Inthe previous tutorial I have some components, The parentComponent.vue and firstComponent.vue
if we want to get data from getters we must import like this
import {mapGetters} from 'vuex';
if we want to use the data we must put the data in "Computed" property ,
for more details about computed property https://vuejs.org/v2/guide/computed.html
computed:{
...mapGetters([
'name'
])
}
'name' : its the name in getters.js . you can print it as usuall with {{name}}
Use Actions in Vuex
How to use action methods in vuex, one of them is event click @click
or v-on: click
. you can use this event @click = "nameofFunction()"
in this example the functions is @click="changeName()"
and import the mapActions
import {mapGetters,mapActions} from 'vuex';
Define in methods
methods:{
...mapActions([
'changeName'
]),
}
'changeName' : its a name of function in actions.js.this functions will be commit mutations
import * as mutations from './types'
export default {
[mutations.CHANGE_NAME](state){
state.name = "Michael Jackson"
},
}
In mutations , the data state will be changed when you Click button with action changeName() from Jhon doe to michael jakson
We have changed the existing data in our state.js by calling the action changeName () and then change it in the mutations part, so the change is in mutations.js, now we know how to communicating between components with vuex, this tutorial is a simple way,
the purpose of this tutorial so we know about the states, getters, mutations, and actions. so much of me, hopefully this tutorial useful for you guys
Posted on Utopian.io - Rewarding Open Source Contributors
Congratulations @alfarisi94! You have completed some achievement on Steemit and have been rewarded with new badge(s) :
Click on any badge to view your own Board of Honor on SteemitBoard.
For more information about SteemitBoard, click here
If you no longer want to receive notifications, reply to this comment with the word
STOP
Your contribution cannot be approved yet. See the Utopian Rules.
You may edit your post here, as shown below:
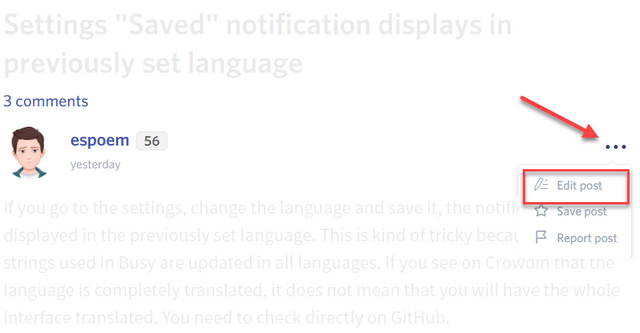
You can contact us on Discord.
[utopian-moderator]
i've edited my post. thank you :)
Thank you for the contribution. It has been approved.
You can contact us on Discord.
[utopian-moderator]
Hey @alfarisi94 I am @utopian-io. I have just upvoted you!
Achievements
Suggestions
Get Noticed!
Community-Driven Witness!
I am the first and only Steem Community-Driven Witness. Participate on Discord. Lets GROW TOGETHER!
Up-vote this comment to grow my power and help Open Source contributions like this one. Want to chat? Join me on Discord https://discord.gg/Pc8HG9x